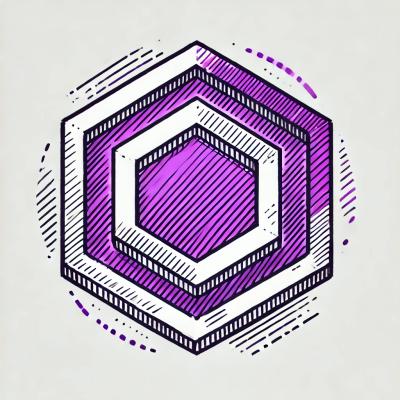
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
@francoisv/react-store
Advanced tools
React store
# with yarn
yarn add @francoisv/react-store
# with npm
npm i -S @francoisv/react-store
First, let's get our dependencies:
import React from 'react'
import store, { withStore } from '@francoisv/react-store'
Now let's create a store array for the todos. For the sake of simplicity, todos are plain strings. We'll start with one todo in the list.
const todos = store.Array('Buy milk')
Now let's just create a list component with it
const TodosView = () => (
<ul>
{ store.get(todos).map(todo => ( <li key={ todo }>{ todo }</li> )) }
</ul>
)
We want the component to update whenever todos
changes. For that, we'll use the HOC withStore
const Todos = withStore(todos)(TodosView)
Let's add a form to add a new todo.
const newTodo = store.string()
const AddTodo = withStore(newTodo)(() => (
<form>
<input
value={ store.get(newTodo) }
onChange={ event => store.set(newTodo, event.target.value) }
/>
<button onClick={ () => store.push(todos, store.get(newTodo)) }>
Add
</button>
</form>
))
Now let's create a way to delete todos when they are done. Let's add a checkbox next to each todo to do that.
const Todo = props => (
<div>
<input
type="checkbox"
onChange={ () => store.filter(todos, todo => todo !== props.todo) }
/>
{ props.todo }
</div>
)
We can now call each todo such as:
{ store.get(todos).map(todo => ( <li key={ todo }><Todo todo={ todo } /></li> )) }
Let's say we want to edit the todo. Let's level our Todo component. We'll use the props to create a local store that will be passed in the props
const state = props => ({ nextTodo: store.string() })
const Todo = props => {
const onSave = () => store.map(
todos,
todo => {
// if this current todo, change it with new value
if (todo === props.todo) {
return store.get(props.nextTodo)
}
return todo
}
)
return (
<div>
<input
type="checkbox"
onChange={ () => store.filter(todos, todo => todo !== props.todo) }
/>
<input
value={ store.get(props.nextTodo) }
onChange={ event => store.set(props.nextTodo, event.target.value) }
/>
<button onClick={ onSave }>
Change
</button>
</div>
)
}
FAQs
react-store ===
The npm package @francoisv/react-store receives a total of 4 weekly downloads. As such, @francoisv/react-store popularity was classified as not popular.
We found that @francoisv/react-store demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.