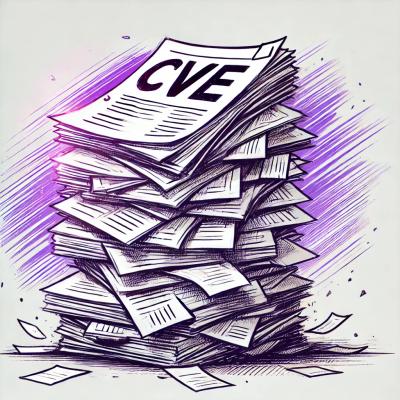
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@fresh8/copacetic
Advanced tools
A package to help your service check the health of its dependencies.
A package to help your service check the health of its dependencies.
Node v6.4.0 and above
npm install @fresh8/copacetic --save
const Copacetic = require('@fresh8/copacetic')
const level = require('@fresh8/copacetic').dependencyLevel
const copacetic = Copacetic()
// Register a dependency
copacetic.registerDependency({
name: 'My-Dependency',
url: 'https://my-Dependency.io',
// Defaults to SOFT
level: level.HARD
})
// Check its health
copacetic
.check({
name: 'My-Dependency',
retries: 2
})
.on('healthy', (Dependency) => {
/**
* { name: 'My-Dependency',
* healthy: true/false,
* lastChecked: Date,
* level: 'SOFT'
* }
*/
})
.on('unhealthy', (Dependency) => {
// Handle degraded state...
})
// in promise mode
copacetic.eventEmitterMode = false
copacetic
.check({ name: 'my-web-service' })
.then((res) => {
console.log(`${res.name} is healthy!`)
})
.catch((err) => {
console.log(err)
})
import * as Copacetic from '@fresh8/copacetic'
const copacetic = Copacetic('my-service')
const myDependencyOverHttp : Copacetic.DependencyOptions = {
name: 'my-web-service',
url: 'http://example.com'
}
copacetic.registerDependency(myDependencyOverHttp)
instance
.check({ name: 'my-web-service' })
.on('healthy', (res: Copacetic.Health) => {
// do something with your healthy dependency :)
})
.on('unhealthy', (res: Copacetic.Health) => {
// handle degraded state
})
// in promise mode
copacetic.eventEmitterMode = false
async function waitForWebService () {
try {
const res = await instance
.check<Copacetic.Health>({ name: 'my-web-service' })
console.log(`${res.name} is healthy!`)
} catch (err) {
console.log(err)
}
}
const Copacetic = require('@fresh8/copacetic')
const level = require('@fresh8/copacetic').dependencyLevel
const copacetic = Copacetic("A name", false)
Copacetic.cluster.attach(copacetic)
if (process.worker) {
//register your usual dependencies like databases
//use the line below to have the worker ask the master process a full health report
copacetic.checkCluster() //`checkCluster` is only defined if the process is a worker and if you called `attach()`
.then(console.log)
} else {
copacetic.checkAll()
.then(() => {
console.log(copacetic.healthReport) //Contains health information as reported by the workers
})
}
EventEmitter
Object
The full health report including isHealthy and dependencies
EventEmitter
Kind: global class
Extends: EventEmitter
EventEmitter
Boolean
Array.<DependencyHealth>
HealthReport
Dependency
Boolean
Copacetic
Copacetic
Copacetic
Copacetic
Copacetic
Copacetic
Promise
Promise
Param | Type | Default | Description |
---|---|---|---|
[name] | String | '' | The name of your service |
Boolean
Kind: instance property of Copacetic
Returns: Boolean
- Copacetic is healthy when all hard dependencies are healthy
Array.<DependencyHealth>
Kind: instance property of Copacetic
Returns: Array.<DependencyHealth>
- Health information on all dependencies
HealthReport
Kind: instance property of Copacetic
Returns: HealthReport
- A full report of health information and dependencies
Dependency
Kind: instance method of Copacetic
Param | Type |
---|---|
dependency | Dependency | String |
Boolean
Kind: instance method of Copacetic
Returns: Boolean
- Whether the dependency has been registered
Param | Type |
---|---|
dependency | Dependency | String |
Copacetic
Adds a dependency to a Copacetic instance
Kind: instance method of Copacetic
Param | Type | Description |
---|---|---|
opts | Object | The configuration for a dependency |
Copacetic
Removes a dependency from a Copacetic instance
Kind: instance method of Copacetic
Param | Type | Description |
---|---|---|
name | String | The name used to identify a dependency |
Copacetic
Polls the health of all registered dependencies
Kind: instance method of Copacetic
Param | Type | Default |
---|---|---|
[interval] | String | '5 seconds' |
[parallel] | Boolean | true |
[schedule] | String | 'start' |
Copacetic
Polls the health of a set of dependencies
Kind: instance method of Copacetic
Emits: Copacetic#event:health
Param | Type | Default | Description |
---|---|---|---|
[name] | String | The identifier of a single dependency to be checked | |
[dependencies] | Array.<Object> | An explicit set of dependencies to be polled | |
[interval] | String | '5 seconds' | |
[parallel] | Boolean | true | Kick of health checks in parallel or series |
[schedule] | String | 'start' | Schedule the next check to start (interval - ms) |
Example
copacetic.poll({
dependencies: [
{ name: 'my-dep' },
{ name: 'my-other-dep', retries: 2, maxDelay: '2 seconds' }
],
schedule: 'end',
interval: '1 minute 30 seconds'
})
.on('health', (serviceHealth, stop) => {
// Do something with the result
// [{ name: String, health: Boolean, level: HARD/SOFT, lastCheck: Date }]
// stop polling
stop()
})
Example
copacetic.poll({ name: 'my-dependency' })
.on('health', () => { ... Do something })
stops polling registered dependencies
Kind: instance method of Copacetic
Copacetic
Checks the health of all registered dependencies
Kind: instance method of Copacetic
Param | Type | Default | Description |
---|---|---|---|
[parallel] | Boolean | true | Kick of health checks in parallel or series |
Copacetic
Checks the health of a set, or single dependency
Kind: instance method of Copacetic
Emits: Copacetic#event:health
, Copacetic#event:healthy
, Copacetic#event:unhealthy
Param | Type | Default | Description |
---|---|---|---|
[name] | String | The identifier of a single dependency to be checked | |
[dependencies] | Array.<Object> | An explicit set of dependencies to be checked | |
[retries] | Integer | 1 | How many times should a dependency be checked, until it is deemed unhealthy |
[parallel] | Boolean | true | Kick of health checks in parallel or series |
Example
copacetic.check({ name: 'my-dependency' })
Example
copacetic.check({ name: 'my-dependency', retries: 5 })
.on('healthy', serviceHealth => { ... Do stuff })
.on('unhealthy', serviceHealth => { ... Handle degraded state })
Example
copacetic.check({ dependencies: [
{ name: 'my-dep' },
{ name: 'my-other-dep', retries: 2, maxDelay: '1 second' }
] })
.on('health', (servicesHealth) => {
// Do something with the result
// [{ name: String, health: Boolean, level: HARD/SOFT, lastCheck: Date }]
})
Example
copacetic.check({ name: 'my-dependency' })
.then((health) => { ... Do Stuff })
.catch((err) => { ... Handle degraded state })
Convenience method that waits for a single, or set of dependencies to become healthy. Calling this means copacetic will keep re-checking indefinitely until the dependency(s) become healthy. If you want more control, use .check().
Kind: instance method of Copacetic
Param | Type | Description |
---|---|---|
opts | Object | options accepted by check() |
Example
// wait indefinitely
copacetic.waitFor({ name: 'my-dependency'})
.on('healthy', serviceHealth => { ... Do stuff })
Example
// give up after 5 tries
copacetic.waitFor({ name: 'my-dependency', retries: 5})
.on('healthy', serviceHealth => { ... Do stuff })
Promise
Kind: instance method of Copacetic
Param | Type | Description |
---|---|---|
name | String | The name used to identify a dependency |
maxDelay | Integer | The maximum interval of time to wait when retrying |
Promise
Checks an array of dependencies in parallel or sequantially
Kind: instance method of Copacetic
Param | Type |
---|---|
dependencies | Array.<Dependency> |
parallel | Boolean |
Object
The full health report including isHealthy and dependencies
Kind: global typedef
Properties
Name | Type | Description |
---|---|---|
isHealthy | Boolean | The result of isHealthy |
Name | String | |
dependencies | Array.<DependencyHealth> | The result of healthInfo |
FAQs
A package to help your service check the health of its dependencies.
The npm package @fresh8/copacetic receives a total of 48 weekly downloads. As such, @fresh8/copacetic popularity was classified as not popular.
We found that @fresh8/copacetic demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 14 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.