Frontegg Client
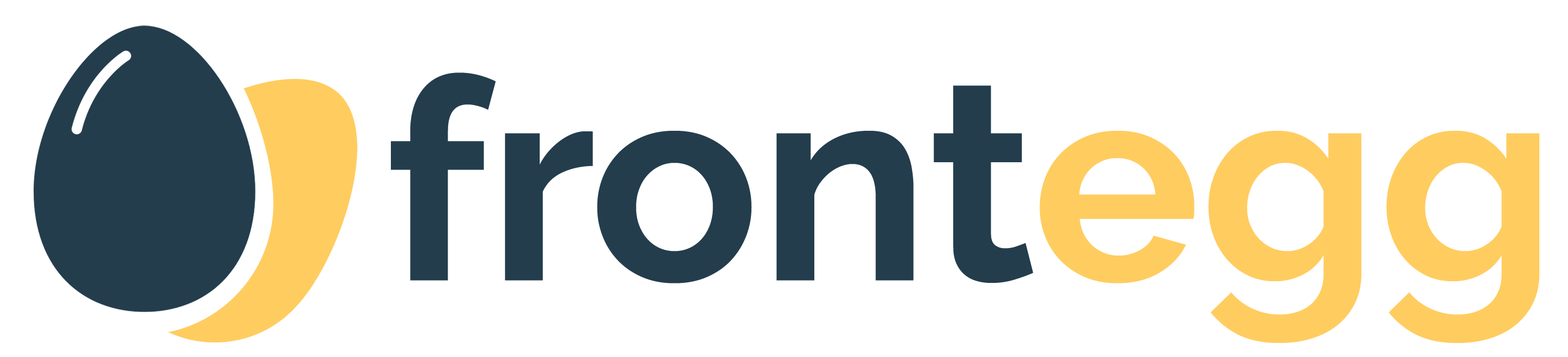
Frontegg is a web platform where SaaS companies can set up their fully managed, scalable and brand aware - SaaS features and integrate them into their SaaS portals in up to 5 lines of code.
Installation
Use the package manager npm to install frontegg client.
npm install @frontegg/client
Breaking changes
v2.0 Events client upgraded to use the v3 Events API
As we grow as a company we also provide new and improved APIs such as the new v3 Events API.
This will allow your to configure more and worry less about channels. It is still not perfect, but we're getting there.
With the new v3 api you'll be able to use the frontegg portal to map events to channels in order to allow your customers to configure their own channels.
The new client will no longer send the channels you wish to trigger, and just use the event's payload.
Please note that email & slack may still require further data (like the email template or slack message format).
Unlike previous clients, the new client requires that you pass a separate authenticator.
Example:
const authenticator = new FronteggAuthenticator();
await authenticator.init('<YOUR_CLIENT_ID>', '<YOUR_API_KEY>')
const eventsClient = new EventsClient(authenticator);
const id = await eventsClient.send(
'my-tenant-id',
{
eventKey: 'my.event',
data: {
description: 'my description',
title: 'my title'
},
channelConfiguration: {
email: {
from: 'email@email.com',
html: '<div>{{title}}</div>',
subject: 'subject'
},
slack: {
emails: ['email@email.com'],
slackMessageConfig: {
}
}
}
})
Usage
Frontegg offers multiple components for integration with the Frontegg's scaleable back end and front end libraries
Middleware
When using Frontegg's managed UI features and UI libraries, Frontegg allow simple integration via middleware usage
To use the Frontegg's middleware import the frontegg middleware from the @frontegg/client library
const { frontegg, FronteggPermissions } = require('@frontegg/client');
And use the following lines after the authentication verification
app.use('/frontegg', frontegg({
clientId: 'my-client-id',
apiKey: 'my-api-key',
contextResolver: (req) => {
const email = req.context.user;
const tenantId = req.context.tenantId;
const permissions = [FronteggPermissions.All];
return {
email,
tenantId,
permissions
};
}
}))
NextJS Middleware
To use the Frontegg's middleware inside NextJS project:
-
Add a new route to your project /pages/api/frontegg/[...param].ts
-
Import fronteggNextJs from the @frontegg/client library
const { fronteggNextJs, FronteggPermissions } = require('@frontegg/client');
-
And export the middleware from /pages/api/frontegg/[...param].ts
export default fronteggNextJs({
clientId: 'my-client-id',
apiKey: 'my-api-key',
contextResolver: (req) => {
const email = req.context.user;
const tenantId = req.context.tenantId;
const permissions = [FronteggPermissions.All];
return {
email,
tenantId,
permissions
};
}
})
Frontegg permissions
When using the Frontegg middleware library, you can choose which functionality is enabled for your user based on his role or based on any other business logic your application holds.
Controlling Frontegg permissions
Controlling the permissions is done via the Frontegg middleware by injecting the permissions array
const permissions = [FronteggPermissions.All];
const permissions = [FronteggPermissions.Audit];
const permissions = [FronteggPermissions.Audit.Read, FronteggPermissions.Audit.Stats];
Audits
Let your customers record the events, activities and changes made to their tenant.
Frontegg’s Managed Audit Logs feature allows a SaaS company to embed an end-to-end working feature in just 5 lines of code.
Sending audits
const { AuditsClient } = require('@frontegg/client')
const audits = new AuditsClient()
await audits.init('MY-CLIENT-ID', 'MY-AUDITS-KEY')
await audits.sendAudit({
tenantId: 'my-tenant-id',
time: Date(),
user: 'info@frontegg.com',
resource: 'Portal',
action: 'Login',
severity: 'Medium',
ip: '1.2.3.4'
})
Fetching audits
const { AuditsClient } = require('@frontegg/client')
const audits = new AuditsClient()
await audits.init('MY-CLIENT-ID', 'MY-AUDITS-KEY')
const { data, total } = await audits.getAudits({
tenantId: 'my-tenant-id',
filter: 'any-text-filter',
sortBy: 'my-sort-field',
sortDirection: 'asc | desc'
offset: 0,
count: 50
})
Notifications
Allow your customers to be aware of any important business related event they need to pay attention to, both in the dashboard and even when offline.
Frontegg’s Managed Notifications feature allows a SaaS company to embed an end-to-end working feature in just 5 lines of code.
Sending notifications
import { NotificationsClient } from '@frontegg/client'
const notificationsClient = new NotificationsClient();
await notificationsClient.init('YOUR-CLIENT-ID', 'YOUR-API-KEY');
const notification = {
"title": "Notification Title",
"body": "Notification Body",
"severity": "info",
"url": "example.com"
}
await notificationsClient.sendToUser('RECEPIENT-USER-ID', 'RECEPIENT-TENANT-ID', notification)
await notificationsClient.sendToTenantUsers('RECEPIENT-TENANT-ID', notification)
await notificationsClient.sendToAllUsers(notification)
Role Based Access Middleware
Protect your routes and controllers with a simple middleware and policy
The middleware needs to run BEFORE the rest of your routes and controllers and is initiated via a simple policy and context hook
app.use(RbacMiddleware({
contextResolver: async (req) => { return { roles: ['admin'], permissions: ['rule.delete'] } },
policy: {
default: 'deny',
rules: [{
url: '/api/admin/*',
method: '*',
requiredPermissions: ['write']
}, {
url: '/api/*',
method: '*',
requiredPermissions: ['read']
}, {
url: '*',
method: '*',
requiredRoles: [],
requiredPermissions: []
}]
}
}));