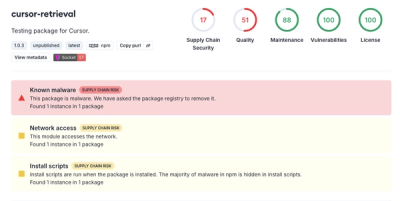
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
@fullcalendar/interaction
Advanced tools
Calendar functionality for event drag-n-drop, event resizing, date clicking, and date selecting
@fullcalendar/interaction is an add-on for the FullCalendar library that provides a set of features to enable user interactions with the calendar. This includes functionalities like dragging and dropping events, resizing events, and selecting date ranges.
Event Dragging
This feature allows users to drag and drop events within the calendar. The 'editable' property is set to true to enable this functionality.
{
"import": "import interactionPlugin from '@fullcalendar/interaction';",
"calendarOptions": {
"plugins": ["interactionPlugin"],
"editable": true,
"events": [
{ "title": "Event 1", "start": "2023-10-01" },
{ "title": "Event 2", "start": "2023-10-02" }
]
}
}
Event Resizing
This feature allows users to resize events by dragging the edges of the event. The 'editable' property is set to true to enable this functionality.
{
"import": "import interactionPlugin from '@fullcalendar/interaction';",
"calendarOptions": {
"plugins": ["interactionPlugin"],
"editable": true,
"events": [
{ "title": "Event 1", "start": "2023-10-01", "end": "2023-10-02" },
{ "title": "Event 2", "start": "2023-10-02", "end": "2023-10-03" }
]
}
}
Date Selection
This feature allows users to select date ranges on the calendar. The 'selectable' property is set to true, and a callback function is provided to handle the selection event.
{
"import": "import interactionPlugin from '@fullcalendar/interaction';",
"calendarOptions": {
"plugins": ["interactionPlugin"],
"selectable": true,
"select": function(info) {
alert('Selected range: ' + info.startStr + ' to ' + info.endStr);
}
}
}
React Big Calendar is a full-featured calendar component for React. It offers similar functionalities such as drag-and-drop, resizing, and date selection. However, it is specifically designed for React, whereas @fullcalendar/interaction can be used with various frameworks.
TUI Calendar is a JavaScript calendar library that provides a wide range of features including drag-and-drop, resizing, and date selection. It is similar to @fullcalendar/interaction but offers additional features like multiple view types and customizable themes.
DHTMLX Scheduler is a JavaScript event calendar that offers drag-and-drop, resizing, and date selection functionalities. It is highly customizable and provides a variety of views and configurations, making it a strong alternative to @fullcalendar/interaction.
Calendar functionality for event drag-n-drop, event resizing, date clicking, and date selecting
Install the FullCalendar core package, the interaction plugin, and any other plugins (like daygrid):
npm install @fullcalendar/core @fullcalendar/interaction @fullcalendar/daygrid
Instantiate a Calendar with the necessary plugins and options:
import { Calendar } from '@fullcalendar/core'
import interactionPlugin from '@fullcalendar/interaction'
import dayGridPlugin from '@fullcalendar/daygrid'
const calendarEl = document.getElementById('calendar')
const calendar = new Calendar(calendarEl, {
plugins: [
interactionPlugin,
dayGridPlugin
],
initialView: 'dayGridMonth',
editable: true, // important for activating event interactions!
selectable: true, // important for activating date selectability!
events: [
{ title: 'Meeting', start: new Date() }
]
})
calendar.render()
6.1.15 (2024-07-12)
FAQs
Calendar functionality for event drag-n-drop, event resizing, date clicking, and date selecting
The npm package @fullcalendar/interaction receives a total of 475,148 weekly downloads. As such, @fullcalendar/interaction popularity was classified as popular.
We found that @fullcalendar/interaction demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.