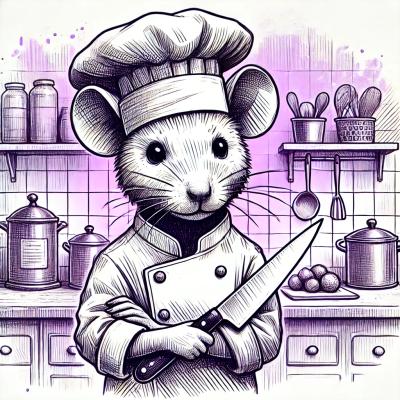
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@fullcalendar/react
Advanced tools
@fullcalendar/react is a powerful and flexible calendar component for React applications. It allows developers to create interactive and customizable calendars with ease. The package supports a wide range of features including event management, date navigation, and various view options.
Basic Calendar Setup
This code sets up a basic calendar using the dayGridPlugin to display a month view. It demonstrates how to import and use the FullCalendar component in a React application.
import React from 'react';
import FullCalendar from '@fullcalendar/react';
import dayGridPlugin from '@fullcalendar/daygrid';
function MyCalendar() {
return (
<FullCalendar
plugins={[dayGridPlugin]}
initialView="dayGridMonth"
/>
);
}
export default MyCalendar;
Event Management
This code demonstrates how to manage events in the calendar. It shows how to pass an array of event objects to the FullCalendar component to display events on specific dates.
import React from 'react';
import FullCalendar from '@fullcalendar/react';
import dayGridPlugin from '@fullcalendar/daygrid';
function MyCalendar() {
const events = [
{ title: 'Event 1', date: '2023-10-01' },
{ title: 'Event 2', date: '2023-10-02' }
];
return (
<FullCalendar
plugins={[dayGridPlugin]}
initialView="dayGridMonth"
events={events}
/>
);
}
export default MyCalendar;
Customizing Event Appearance
This code shows how to customize the appearance of events in the calendar. It uses the eventContent prop to render events with custom styles based on their properties.
import React from 'react';
import FullCalendar from '@fullcalendar/react';
import dayGridPlugin from '@fullcalendar/daygrid';
function MyCalendar() {
const events = [
{ title: 'Event 1', date: '2023-10-01', color: 'red' },
{ title: 'Event 2', date: '2023-10-02', color: 'blue' }
];
return (
<FullCalendar
plugins={[dayGridPlugin]}
initialView="dayGridMonth"
events={events}
eventContent={(eventInfo) => (
<div style={{ color: eventInfo.event.extendedProps.color }}>
{eventInfo.event.title}
</div>
)}
/>
);
}
export default MyCalendar;
react-big-calendar is a similar package that provides a calendar component for React applications. It offers a range of views (month, week, day) and supports event management. Compared to @fullcalendar/react, react-big-calendar is more focused on simplicity and ease of use, but it may lack some of the advanced customization options available in @fullcalendar/react.
react-calendar is another alternative that provides a simple and lightweight calendar component for React. It is suitable for basic date picking and calendar display needs. While it is easier to set up and use, it does not offer the same level of functionality and customization as @fullcalendar/react.
rc-calendar is a React component for date and time picking. It is part of the rc-components library and provides a range of features for date selection and calendar display. Compared to @fullcalendar/react, rc-calendar is more focused on date picking rather than full-featured calendar views and event management.
The official React Component for FullCalendar
Install the React connector, the core package, and any plugins (like daygrid):
npm install @fullcalendar/react @fullcalendar/core @fullcalendar/daygrid
Render a FullCalendar
component, supplying options as props:
import FullCalendar from '@fullcalendar/react'
import dayGridPlugin from '@fullcalendar/daygrid'
const events = [
{ title: 'Meeting', start: new Date() }
]
export function DemoApp() {
return (
<div>
<h1>Demo App</h1>
<FullCalendar
plugins={[dayGridPlugin]}
initialView='dayGridMonth'
weekends={false}
events={events}
eventContent={renderEventContent}
/>
</div>
)
}
// a custom render function
function renderEventContent(eventInfo) {
return (
<>
<b>{eventInfo.timeText}</b>
<i>{eventInfo.event.title}</i>
</>
)
}
You must install this repo with PNPM:
pnpm install
Available scripts (via pnpm run <script>
):
build
- build production-ready dist filesdev
- build & watch development dist filestest
- test headlesslytest:dev
- test interactivelylint
clean
FAQs
The official React Component for FullCalendar
The npm package @fullcalendar/react receives a total of 417,334 weekly downloads. As such, @fullcalendar/react popularity was classified as popular.
We found that @fullcalendar/react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.