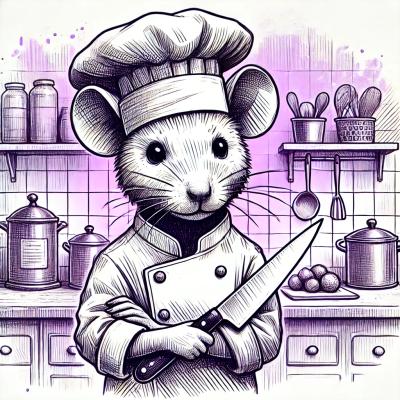
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@gar/promisify
Advanced tools
The @gar/promisify npm package is designed to convert callback-based functions into Promises. This is particularly useful when working with older Node.js or JavaScript libraries that do not natively support Promises, allowing developers to write cleaner, more modern asynchronous code using async/await syntax or .then() chains.
Promisifying a single function
Converts a single callback-based function (in this example, fs.readFile) into a function that returns a Promise. This allows for the use of .then() and .catch() for handling asynchronous operations.
const promisify = require('@gar/promisify');
const fs = require('fs');
const readFileAsync = promisify(fs.readFile);
readFileAsync('example.txt', 'utf8').then(contents => {
console.log(contents);
}).catch(error => {
console.error(error);
});
Promisifying an object's methods
Converts all callback-based functions within an object (in this example, all methods of the 'fs' module) into functions that return Promises. This simplifies working with modules that have multiple asynchronous methods.
const promisify = require('@gar/promisify');
const fs = promisify.all(require('fs'));
fs.readFile('example.txt', 'utf8').then(contents => {
console.log(contents);
}).catch(error => {
console.error(error);
});
Built into Node.js as part of the 'util' module, util.promisify performs a similar function to @gar/promisify by converting callback-based functions into Promises. The main difference is that util.promisify is a native feature of Node.js, requiring no additional installation, but it does not offer the ability to promisify all methods of an object at once.
Bluebird is a comprehensive promise library that offers a wide range of features including but not limited to promisification. It provides a 'promisify' and 'promisifyAll' method similar to @gar/promisify, but also includes utilities for concurrency control, mapping, reducing, and more. Bluebird is known for its performance and additional features beyond promisification.
Pify is a lightweight promisification library similar to @gar/promisify. It allows for promisifying single functions or entire modules with an easy-to-use API. Compared to @gar/promisify, pify is focused solely on promisification and does not include additional utilities or features, making it a simple and effective choice for projects that only require promisification.
This module leverages es6 Proxy and Reflect to promisify every function in an object or class instance.
It assumes the callback that the function is expecting is the last
parameter, and that it is an error-first callback with only one value,
i.e. (err, value) => ...
. This mirrors node's util.promisify
method.
In order that you can use it as a one-stop-shop for all your promisify
needs, you can also pass it a function. That function will be
promisified as normal using node's built-in util.promisify
method.
node's custom promisified
functions
will also be mirrored, further allowing this to be a drop-in replacement
for the built-in util.promisify
.
Promisify an entire object
const promisify = require('@gar/promisify')
class Foo {
constructor (attr) {
this.attr = attr
}
double (input, cb) {
cb(null, input * 2)
}
const foo = new Foo('baz')
const promisified = promisify(foo)
console.log(promisified.attr)
console.log(await promisified.double(1024))
Promisify a function
const promisify = require('@gar/promisify')
function foo (a, cb) {
if (a !== 'bad') {
return cb(null, 'ok')
}
return cb('not ok')
}
const promisified = promisify(foo)
// This will resolve to 'ok'
promisified('good')
// this will reject
promisified('bad')
FAQs
Promisify an entire class or object
The npm package @gar/promisify receives a total of 2,547,321 weekly downloads. As such, @gar/promisify popularity was classified as popular.
We found that @gar/promisify demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.