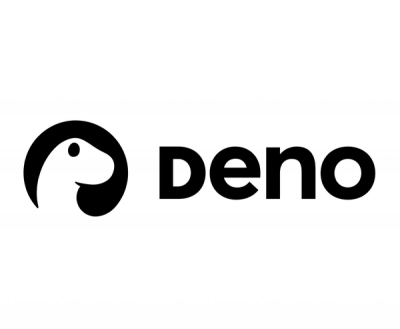
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@gary50613/discord.js-command-handler
Advanced tools
Simple command handler for discord.js
run this in your terminal
npm i @gary50613/discord.js-command-handler
npm i
npm test
basic how to initialize with options
const Discord = require('discord.js')
// import a command
const ping = require("./commands/ping")
const bot = new Discord.Client()
require("@gary50613/djs-command-handler")(bot, {
prefix: '.',
// options
})
// load a whole folder's commands
bot.commands.loadCommands("./commands")
// register a command
bot.commands.register(new ping())
// or register multiple command at the same time
bot.commands.register(new ping(), ..., ...)
// listen to event
bot.commands.on("dm", (m) => {
m.channel.send("u can only use command in a guild!")
})
bot.login(process.env.TOKEN)
make a command
const { Command } = require("@gary50613/djs-command-handler")
class Ping extends Command {
constructor() {
super(
"ping", // name
"ping the bot", // description
".ping", // usage
"general", // group
["pong"] // alias
);
}
// execute function to call
async execute(bot, message, args) {
// just write like normal discord.js
message.reply('pong!')
}
}
module.exports = Ping
basic how to initialize with options
import { Client } from "discord.js"
import init from "@gary50613/discord.js-command-handler"
// import a command
import ping from "./commands/Ping"
const bot = new Client()
init(bot, {
prefix: ".",
// options
})
// load a whole folder's commands
bot.commands.loadFolder("./commands")
// register a command
bot.commands.register(new ping())
// or register multiple command at the same time
bot.commands.register(new ping(), ..., ...)
// listen to event
bot.commands.on("dm", (m) => {
m.channel.send("u can only use command in a guild!")
})
bot.login(process.env.TOKEN)
make a command
import { Command } from "@gary50613/discord.js-command-handler";
import { Client, Guild, GuildMember, Message } from "discord.js";
export default class Ping extends Command {
public constructor() {
super(
"ping", // name
"ping the bot", // description
".ping", // usage
"general", // group
["pong"] // alias
);
}
public async execute(bot: Client, message: Message, args: string[]) {
// just write like normal discord.js
message.reply("pong!")
}
}
type | description | parameter |
---|---|---|
dm | user execute a command in dm | Message |
ratelimit | user get ratelimited | Millisecond, Message |
execute | command successfully executed | Command, Message |
error | command execute error | Error, Command, Message |
promiseError | promise rejection | Error, Command, Message |
{
ratelimit: {
enable: false, // whether enable ratelimit
interval: 5000, // interval to limit
bypass: {
users: [], // specific users ID can bypass ratelimit
permissions: ["ADMINISTRATOR"], // specific perimissions FLAG can bypass ratelimit
roles: [] // // specific roles ID can bypass ratelimit
}
},
prefix: "PREFIX", // bot's prefix
dm: false, // whether accept dm commands
bot: false // whether accept bot execute command
}
🧑💻 Kane
Feel free to open issue or join my discord server
FAQs
simple discord.js v13 command handler
The npm package @gary50613/discord.js-command-handler receives a total of 20 weekly downloads. As such, @gary50613/discord.js-command-handler popularity was classified as not popular.
We found that @gary50613/discord.js-command-handler demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.