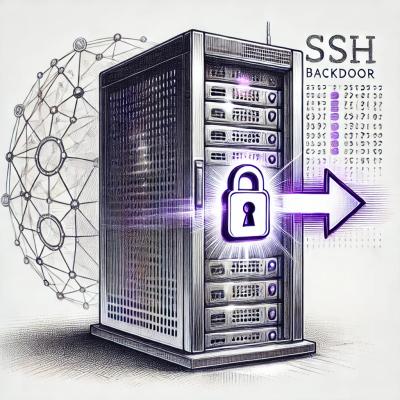
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@geoapify/geocoder-autocomplete
Advanced tools
Geoapify Geocoder Autocomplete is a JavaScript(TypeScript) library that provides autocomplete functionality for the Geoapify Geocoding API:
The library makes a call to Geoapify Place Details API on user select events. The API lets to get detailed information about the place selected as well as place category and place geometry (building polygon or region boundary). Note, that the Place Details API call costs an additional 'geocoding & places' request. Use the skipDetails
option to switch the functionality off.
You can also:
You will need an API key to execute Geoapify Geocoding requests. Register and get an API key for Free on myprojects.geoapify.com. Geoapify has a Freemium pricing model. You can start for Free and extend when you need it.
npm install @geoapify/geocoder-autocomplete
# or
yarn add @geoapify/geocoder-autocomplete
<html>
<head>
<script src=".../index.min.js"></script>
<link rel="stylesheet" type="text/css" href=".../minimal.css">
...
</head>
...
</html>
You can use UNPKG to refer or download the library:
https://unpkg.com/@geoapify/geocoder-autocomplete@^1/dist/index.min.js
https://unpkg.com/@geoapify/geocoder-autocomplete@^1/styles/minimal.css
The autocomplete input will be added into the container element and will take the element full width:
<div id="autocomplete" class="autocomplete-container"></div>
The container element must have position: absolute;
or position: relative;
.autocomplete-container {
position: relative;
}
import { GeocoderAutocomplete } from '@geoapify/geocoder-autocomplete';
const autocomplete = new GeocoderAutocomplete(
document.getElementById("autocomplete"),
'YOUR_API_KEY',
{ /* Geocoder options */ });
autocomplete.on('select', (location) => {
// check selected location here
});
autocomplete.on('suggestions', (suggestions) => {
// process suggestions here
});
autocomplete
when you added it as a script:const autocompleteInput = new autocomplete.GeocoderAutocomplete(
document.getElementById("autocomplete"),
'YOUR_API_KEY',
{ /* Geocoder options */ });
autocompleteInput.on('select', (location) => {
// check selected location here
});
autocompleteInput.on('suggestions', (suggestions) => {
// process suggestions here
});
We provide several Themes within the library:
minimal
and round-borders
- for webpages with light background colorminimal-dark
and round-borders-dark
for webpages with dark background color.You can import the appropriate css-file to your styles:
@import "~@geoapify/geocoder-autocomplete/styles/minimal.css";
or as a link in a HTML-file:
<link rel="stylesheet" type="text/css" href="https://unpkg.com/@geoapify/geocoder-autocomplete@^1/styles/minimal.css">
Option | Type | Description |
---|---|---|
type | country , state , city , postcode , street , amenity | Type of the location |
lang | LanguageCode | Results language |
limit | number | The maximal number of returned suggestions |
placeholder | string | An input field placeholder |
debounceDelay | number | A delay between user input and the API call to prevent unnecessary calls. The default value is 100ms. |
skipIcons | boolean | Don't add icons to suggestions |
skipDetails | boolean | Skip Place Details API call on selection change |
filter | FilterOptions | Filter places by country, boundary, circle, place |
bias | BiasOptions | Prefer places by country, boundary, circle, location |
allowNonVerifiedHouseNumber | boolean | Allow the addition of house numbers that are not verified by the Geocoding API or missing in the database. Check the "Working with non-verified values" section for details. |
allowNonVerifiedStreet | boolean | Allow the addition of streets that are not verified by the Geocoding API or missing in the database. Check the "Working with non-verified values" section for details. |
2-character ISO 639-1 language code: ab
, aa
, af
, ak
, sq
, am
, ar
, an
, hy
, as
, av
, ae
, ay
, az
, bm
, ba
, eu
, be
, bn
, bh
, bi
, bs
, br
, bg
, my
, ca
, ch
, ce
, ny
, zh
, cv
, kw
, co
, cr
, hr
, cs
, da
, dv
, nl
, en
, eo
, et
, ee
, fo
, fj
, fi
, fr
, ff
, gl
, ka
, de
, el
, gn
, gu
, ht
, ha
, he
, hz
, hi
, ho
, hu
, ia
, id
, ie
, ga
, ig
, ik
, io
, is
, it
, iu
, ja
, jv
, kl
, kn
, kr
, ks
, kk
, km
, ki
, rw
, ky
, kv
, kg
, ko
, ku
, kj
, la
, lb
, lg
, li
, ln
, lo
, lt
, lu
, lv
, gv
, mk
, mg
, ms
, ml
, mt
, mi
, mr
, mh
, mn
, na
, nv
, nb
, nd
, ne
, ng
, nn
, no
, ii
, nr
, oc
, oj
, cu
, om
, or
, os
, pa
, pi
, fa
, pl
, ps
, pt
, qu
, rm
, rn
, ro
, ru
, sa
, sc
, sd
, se
, sm
, sg
, sr
, gd
, sn
, si
, sk
, sl
, so
, st
, es
, su
, sw
, ss
, sv
, ta
, te
, tg
, th
, ti
, bo
, tk
, tl
, tn
, to
, tr
, ts
, tt
, tw
, ty
, ug
, uk
, ur
, uz
, ve
, vi
, vo
, wa
, cy
, wo
, fy
, xh
, yi
, yo
, za
.
The Geocoder Autocomplete allows specify the following types of filters:
Name | Filter | Filter Value | Description | Examples |
---|---|---|---|---|
By circle | circle | { lon: number ,lat: number, radiusMeters: number } | Search places inside the circle | filter['circle'] = {lon: -87.770231, lat: 41.878968, radiusMeters: 5000} |
By rectangle | rect | { lon1: number ,lat1: number, lon2: number ,lat2: number} | Search places inside the rectangle | filter['rect'] = {lon1: 89.097540, lat1: 39.668983, lon2: -88.399274, lat2: 40.383412} |
By country | countrycode | CountyCode[] | Search places in the countries | filter['countrycode'] = ['de', 'fr', 'es'] |
By place | place | string | Search for places within a given city or postal code. For example, search for streets within a city. Use the 'place_id' returned by a another search to specify a filter. | filter['place'] = '51ac66e77e9826274059f9426dc08c114840f00101f901dcf3000000000000c00208' |
You can provide filters as initial options or add by calling a function:
options.filter = {
'circle': {lon: -87.770231, lat: 41.878968, radiusMeters: 5000}
};
// or
autocomplete.addFilterByCircle({lon: -87.770231, lat: 41.878968, radiusMeters: 5000});
You can combine several filters (but only one of each type) in one request. The AND logic is applied to the multiple filters.
You can chage priority of the search by setting bias. The Geocoder Autocomplete allows specify the following types of bias:
Name | Bias | Bias Value | Description | Examples |
---|---|---|---|---|
By circle | circle | { lon: number ,lat: number, radiusMeters: number } | First, search places inside the circle, then worldwide | bias['circle'] = {lon: -87.770231, lat: 41.878968, radiusMeters: 5000} |
By rectangle | rect | { lon1: number ,lat1: number, lon2: number ,lat2: number} | First, search places inside the rectangle, then worldwide | bias['rect'] = {lon1: 89.097540, lat1: 39.668983, lon2: -88.399274, lat2: 40.383412} |
By country | countrycode | CountyCode[] | First, search places in the countries, then worldwide | bias['countrycode'] = ['de', 'fr', 'es'] |
By location | proximity | {lon: number ,lat: number} | Prioritize results by farness from the location | bias['proximity'] = {lon: -87.770231, lat: 41.878968} |
You can combine several bias parameters (but only one of each type) in one request. The OR logic is applied to the multiple bias.
NOTE! The default bias for the geocoding requests is "countrycode:auto", the API detects a country by IP address and provides the search there first. Set bias['countrycode'] = ['none']
to avoid prioritization by country.
You can provide filters as initial options or add by calling a function:
options.bias = {
'circle': {lon: -87.770231, lat: 41.878968, radiusMeters: 5000},
'countrycode': ['none']
};
// or
autocomplete.addBiasByCircle({lon: -87.770231, lat: 41.878968, radiusMeters: 5000});
ad
, ae
, af
, ag
, ai
, al
, am
, an
, ao
, ap
, aq
, ar
, as
, at
, au
, aw
, az
, ba
, bb
, bd
, be
, bf
, bg
, bh
, bi
, bj
, bm
, bn
, bo
, br
, bs
, bt
, bv
, bw
, by
, bz
, ca
, cc
, cd
, cf
, cg
, ch
, ci
, ck
, cl
, cm
, cn
, co
, cr
, cu
, cv
, cx
, cy
, cz
, de
, dj
, dk
, dm
, do
, dz
, ec
, ee
, eg
, eh
, er
, es
, et
, eu
, fi
, fj
, fk
, fm
, fo
, fr
, ga
, gb
, gd
, ge
, gf
, gh
, gi
, gl
, gm
, gn
, gp
, gq
, gr
, gs
, gt
, gu
, gw
, gy
, hk
, hm
, hn
, hr
, ht
, hu
, id
, ie
, il
, in
, io
, iq
, ir
, is
, it
, jm
, jo
, jp
, ke
, kg
, kh
, ki
, km
, kn
, kp
, kr
, kw
, ky
, kz
, la
, lb
, lc
, li
, lk
, lr
, ls
, lt
, lu
, lv
, ly
, ma
, mc
, md
, me
, mg
, mh
, mk
, ml
, mm
, mn
, mo
, mp
, mq
, mr
, ms
, mt
, mu
, mv
, mw
, mx
, my
, mz
, na
, nc
, ne
, nf
, ng
, ni
, nl
, no
, np
, nr
, nu
, nz
, om
, pa
, pe
, pf
, pg
, ph
, pk
, pl
, pm
, pr
, ps
, pt
, pw
, py
, qa
, re
, ro
, rs
, ru
, rw
, sa
, sb
, sc
, sd
, se
, sg
, sh
, si
, sj
, sk
, sl
, sm
, sn
, so
, sr
, st
, sv
, sy
, sz
, tc
, td
, tf
, tg
, th
, tj
, tk
, tm
, tn
, to
, tr
, tt
, tv
, tw
, tz
, ua
, ug
, um
, us
, uy
, uz
, va
, vc
, ve
, vg
, vi
, vn
, vu
, wf
, ws
, ye
, yt
, za
, zm
, zw
.Learn more about Geoapify Geocoder options on Geoapify Documentation page.
The library provides an API that allows to set Geoapify Geocoder options during runtime:
const autocomplete = new GeocoderAutocomplete(...);
// set location type
autocomplete.setType(options.type);
// set results language
autocomplete.setLang(options.lang);
// set dropdown elements limit
autocomplete.setLimit(options.limit);
// set filter
autocomplete.addFilterByCountry(codes);
autocomplete.addFilterByCircle(filterByCircle);
autocomplete.addFilterByRect(filterByRect);
autocomplete.addFilterByPlace(placeId);
autocomplete.clearFilters()
// set bias
autocomplete.addBiasByCountry(codes);
autocomplete.addBiasByCircle(biasByCircle);
autocomplete.addBiasByRect(biasByRect);
autocomplete.addBiasByProximity(biasByProximity);
autocomplete.clearBias();
// get and change dropdown list state
autocomplete.isOpen();
autocomplete.open();
autocomplete.close();
You can also set initial value or change display value:
const autocomplete = new GeocoderAutocomplete(...);
autocomplete.setValue(value);
By adding preprocessing and post-processing hooks and suggestions filter you can change the entered/displayed values and suggestions list:
// add preprocess hook
autocomplete.setPreprocessHook((value: string) => {
// return augmented value here
return `${value} ${someAdditionalInfo}`
});
// remove the hook
autocomplete.setPreprocessHook(null);
autocomplete.setPostprocessHook((feature) => {
// feature is GeoJSON feature containing structured address
// return a part of the address to be displayed
return feature.properties.street;
});
// remove the hook
autocomplete.setPostprocessHook(null);
autocomplete.setSuggestionsFilter((features: any[]) => {
// features is an array of GeoJSON features, that contains suggestions
// return filtered array
const processedStreets = [];
const filtered = features.filter(feature => {
if (!feature.properties.street || processedStreets.indexOf(feature.properties.street) >= 0) {
return false;
} else {
processedStreets.push(feature.properties.street);
return true;
}
});
return filtered;
});
// remove the filter
autocomplete.setSuggestionsFilter(null);
You can add event listeners to the Geocoder Autocomplete:
autocomplete.on('select', (location) => {
// check selected location here
});
autocomplete.on('suggestions', (suggestions) => {
// process suggestions here
});
autocomplete.on('input', (userInput) => {
// process user input here
});
autocomplete.on('open', () => {
// dropdown list is opened
});
autocomplete.on('close', () => {
// dropdown list is closed
});
autocomplete.once('open', () => {
// dropdown list is opened, one time callback
});
autocomplete.once('close', () => {
// dropdown list is closed, one time callback
});
The location have GeoJSON.Feature type, suggestions have GeoJSON.Feature[] type. The feature properties contain information about address and location.
Use off()
function to remove event listerers:
// remove open event
autocomplete.off('open', this.onOpen);
// remove all open events
autocomplete.off('open');
Learn more about Geocoder result properties on Geoapify Documentation page.
If you are considering using the Geocoder Autocomplete library to collect postal addresses, it is best to make it more tolerant and allow adding non-verified address parts - house numbers and streets. For example, it may occur that new streets or houses are not yet included in the databases. Therefore, restricting customer addresses will not be a proper strategy. To allow users to add non-verified address parts, use the allowNonVerifiedHouseNumber and allowNonVerifiedStreet parameters.
The API returns a parsed address, the found address, and a match type as results. Using this data, the library extends the result by adding missing values, such as house numbers. The non-verified part has a "non-verified" class which makes the text red by default. The GPS coordinates of the results will point to the actual results and should be adjusted by the user if necessary.
In addition, the result object is extended by the list of non-verified properties. For example:
{
"type": "Feature",
"properties": {
...
"address_line1": "Bürgermeister-Heinrich-Straße 60",
"address_line2": "93077 Bad Abbach, Germany",
"housenumber": "60",
"nonVerifiedParts": [
"housenumber"
]
},
...
}
We provide several Themes within the library:
minimal
and round-borders
- for webpages with light background colorminimal-dark
and round-borders-dark
for webpages with dark background color.However, you have also opportunity to style the component by yourself. Here are the classes used:
Name | Description |
---|---|
.geoapify-autocomplete-input | The input element |
.geoapify-autocomplete-items | The dropdown list |
.geoapify-autocomplete-items .active | Selected item in the dropdown list |
.geoapify-autocomplete-item | The dropdown list item |
.geoapify-autocomplete-item.icon | The dropdown list item icon |
.geoapify-autocomplete-item.text | The dropdown list item text |
.geoapify-close-button | The clear button |
.geoapify-autocomplete-items .main-part .non-verified | A portion of the street address could not be verified by Geocoder |
FAQs
Postal address autocomplete input field
The npm package @geoapify/geocoder-autocomplete receives a total of 2,891 weekly downloads. As such, @geoapify/geocoder-autocomplete popularity was classified as popular.
We found that @geoapify/geocoder-autocomplete demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.