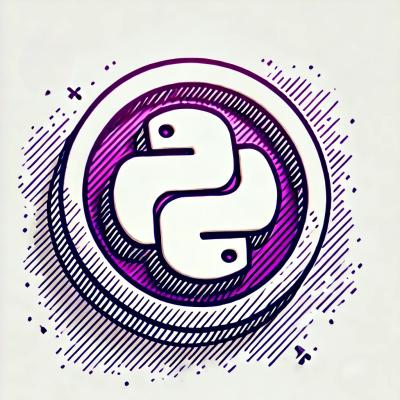
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
@getzep/zep-js
Advanced tools
This is the Javascript client package for the Zep service. For more information about Zep, see https://github.com/getzep/zep
npm install zep-js
Ensure that you have a Zep server running. See https://github.com/getzep/zep.
import {ZepClient, Memory, Message, SearchPayload, SearchResult, ZepClientError, UnexpectedResponseError} from "zep-js";
async function main() {
const base_url = "http://localhost:8000"; // Replace with Zep API URL
const client = new ZepClient(base_url);
try {
// Example usage
const sessionId = "1a1ab1c";
// Add memory
const role = "user";
const content = "I'm looking to plan a trip to Iceland. Can you help me?"
const message = new Message({ role, content });
const memory = new Memory();
memory.messages = [message];
const result = await client.addMemoryAsync(sessionId, memory);
// Search memory
const searchText = "Iceland";
const search_payload = new SearchPayload({ meta: {}, text: searchText });
const search_results = await client.searchMemoryAsync(
sessionId,
search_payload
);
// Iterate through results and print
for (const search_result of search_results) {
const message_content = search_result.message?.content;
console.log("Search Result: ", message_content);
}
// Get Memory
const memories = await client.getMemoryAsync(sessionId);
// Delete memory
const deleteResult = await client.deleteMemoryAsync(sessionId);
} catch (error) {
if (error instanceof ZepClientError) {
console.error("ZepClientError:", error.message);
} else if (error instanceof UnexpectedResponseError) {
console.error("UnexpectedResponseError:", error.message);
} else {
console.error("Unknown error:", error);
}
}
}
Zep JS Client Sync API.
ZepClient
is a TypeScript class for interacting with the Zep API.
constructor(baseURL: string)
: Constructs a new ZepClient instance with the given base URL of the Zep API.getMemory(sessionId: string, lastn?: number)
: Retrieves memory for a specific session.
sessionId
: The ID of the session to retrieve memory for.lastn
: (Optional) The number of most recent memories to retrieve.Memory
objects.addMemory(sessionId: string, memory: Memory)
: Adds a new memory to a specific session.
sessionId
: The ID of the session to add the memory to.memory
: The Memory
object to add to the session.deleteMemory(sessionId: string)
: Deletes the memory of a specific session.
sessionId
: The ID of the session for which the memory should be deleted.searchMemory(sessionId: string, searchPayload: SearchPayload, limit?: number)
: Searches the memory of a specific session based on the search payload provided.
sessionID
: The ID of the session for which the memory should be searched.searchPayload
: The SearchPayload
object containing the search criteria.limit
: (Optional) Limit on the number of search results returned.SearchResult
objects.uuid
: Optional string for the unique identifier of the message.created_at
: Optional string for the creation timestamp of the message.role
: String for the role of the message sender.content
: String for the content of the message.token_count
: Optional number for the token count of the message.metadata?
: Optional metadataconstructor(data: MessageData)
: Constructs a new Message instance.toDict(): MessageData
: Converts the Message instance to a dictionary.uuid
: String for the unique identifier of the summary.created_at
: String for the creation timestamp of the summary.content
: String for the content of the summary.recent_message_uuid
: String for the unique identifier of the most recent message related to the summary.token_count
: Number for the token count of the summary.constructor(data: SummaryData)
: Constructs a new Summary instance.toDict(): SummaryData
: Converts the Summary instance to a dictionary.messages
: Optional array of MessageData for the messages in the memory.metadata
: Optional object for metadata associated with the memory.summary
: Optional SummaryData for the summary of the memory.uuid
: Optional string for the unique identifier of the memory.created_at
: Optional string for the creation timestamp of the memory.token_count
: Optional number for the token count of the memory.constructor(data: MemoryData)
: Constructs a new Memory instance.toDict(): MemoryData
: Converts the Memory instance to a dictionary.metadata
: Object for the metadata of the search payload.text
: String for the text to be searched.constructor(data: MemorySearchPayloadData)
: Constructs a new MemorySearchPayload instance.message
: Optional MessageData for the message in the search result.metadata
: Metadata associated with the search result.summary
: Optional string for the summary of the search result.dist
: Optional number for the distance of the search result.constructor(data: MemorySearchResultData)
: Constructs a new MemorySearchResult instance.constructor(code: number, message: string)
: Constructs a new APIError instance.constructor(message: string, response_data?: any)
: Constructs a new ZepClientError instance.constructor(message: string)
: Constructs a new UnexpectedResponseError instance.Constructor: (message: string)
: Constructs a NotFoundError instanceConstructor: (message: string)
: Constructs an AuthenticationError instanceFAQs
Zep: Fast, scalable building blocks for production LLM apps
The npm package @getzep/zep-js receives a total of 0 weekly downloads. As such, @getzep/zep-js popularity was classified as not popular.
We found that @getzep/zep-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.