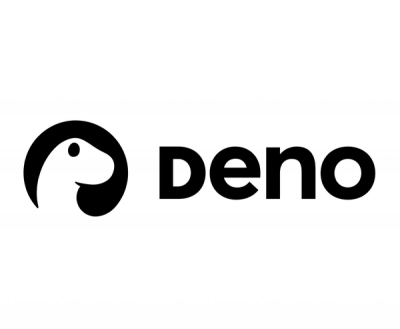
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@goodware/mysql
Advanced tools
Better documentation is coming
ES 2017
npm i --save @goodware/mysql
a) The app instantiates multiple instances to access the same database server. It is recommended to use a single global instance to avoid this issue. b) The app hangs when exiting
The options provided by the constructor and all other methods accept an optional 'logger' function or object. If an object is provided, it must have the method log():
interface Logger {
/**
* @param tags Typically includes a logging level name, like info or debug.
* @param message An object with at least a message property
*/
log(tags: string[] | string, message: Record<string, unknown>): void;
}
The following program outputs 'success' to the console.
const mysql = require('@goodware/mysql');
const pack = require('../package.json');
const config = {
host: 'localhost',
port: 3306,
user: 'root',
password: process.env.DB_PASSWORD,
database: 'mydb',
};
const connector = new mysql(config, console.log); // The second parameter is a logger function
async () => {
const result = await connector.execute( async (connection) => {
const [results] = await connection.query(`select 'success' AS status`);
return results[0].status;
});
console.log(result);
await connector.stop();
}().then(console.info, console.error);
FAQs
A mysql2-based connection helper
The npm package @goodware/mysql receives a total of 4 weekly downloads. As such, @goodware/mysql popularity was classified as not popular.
We found that @goodware/mysql demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.