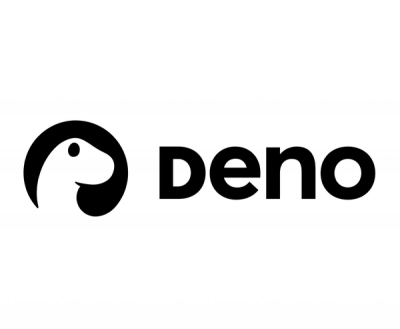
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@google-cloud/common
Advanced tools
@google-cloud/common is a library that provides common functionality for Google Cloud Node.js client libraries. It includes utilities for logging, error handling, and making authenticated API requests.
Logging
The logging feature allows you to create and use a logger to log messages at different levels (info, error, etc.).
const { logger } = require('@google-cloud/common');
const log = logger();
log.info('This is an info message');
log.error('This is an error message');
Error Handling
The error handling feature provides a standardized way to create and handle API errors.
const { ApiError } = require('@google-cloud/common');
try {
throw new ApiError({ message: 'An error occurred', code: 500 });
} catch (err) {
console.error(err.message); // 'An error occurred'
console.error(err.code); // 500
}
Authenticated API Requests
This feature allows you to make authenticated API requests to Google Cloud services.
const { Service } = require('@google-cloud/common');
const service = new Service({
baseUrl: 'https://example.googleapis.com',
scopes: ['https://www.googleapis.com/auth/cloud-platform']
});
service.request({
method: 'GET',
uri: '/v1/resource'
}, (err, response) => {
if (err) {
console.error(err);
} else {
console.log(response);
}
});
Axios is a promise-based HTTP client for the browser and Node.js. It provides similar functionality for making HTTP requests but does not include built-in support for Google Cloud authentication.
Winston is a versatile logging library for Node.js. It offers more advanced logging features compared to @google-cloud/common but does not include other utilities like error handling or authenticated requests.
Request is a simplified HTTP client for Node.js. It provides similar functionality for making HTTP requests but is now deprecated in favor of more modern alternatives like axios.
Node.js Common package
Google Cloud Common node.js module contains stuff used by other Cloud API modules.
Read more about the client libraries for Cloud APIs, including the older Google APIs Client Libraries, in Client Libraries Explained.
Table of contents:
Select or create a Cloud Platform project.
Enable billing for your project.
Set up authentication with a service account so you can access the API from your local workstation.
npm install --save @google-cloud/common
It's unlikely you will need to install this package directly, as it will be
installed as a dependency when you install other @google-cloud
packages.
This library follows Semantic Versioning.
This library is considered to be in alpha. This means it is still a work-in-progress and under active development. Any release is subject to backwards-incompatible changes at any time.
More Information: Google Cloud Platform Launch Stages
Contributions welcome! See the Contributing Guide.
Apache Version 2.0
See LICENSE
FAQs
Common components for Cloud APIs Node.js Client Libraries
We found that @google-cloud/common demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.