What is @googlemaps/markerclusterer?
@googlemaps/markerclusterer is an npm package that provides functionality for clustering a large number of markers on a Google Map. This helps in improving the performance and user experience by grouping nearby markers into clusters, which can be expanded to show individual markers as the user zooms in.
What are @googlemaps/markerclusterer's main functionalities?
Basic Marker Clustering
This feature allows you to create a basic marker clusterer on a Google Map. You initialize the map, create an array of markers, and then use the MarkerClusterer to cluster these markers.
const map = new google.maps.Map(document.getElementById('map'), {
center: { lat: -28.024, lng: 140.887 },
zoom: 4,
});
const markers = locations.map((location, i) => {
return new google.maps.Marker({
position: location,
});
});
new MarkerClusterer({
markers,
map
});
Custom Cluster Icons
This feature allows you to customize the icons used for clusters. You can specify a custom renderer function that returns a marker with a custom icon and label.
const map = new google.maps.Map(document.getElementById('map'), {
center: { lat: -28.024, lng: 140.887 },
zoom: 4,
});
const markers = locations.map((location, i) => {
return new google.maps.Marker({
position: location,
});
});
new MarkerClusterer({
markers,
map,
renderer: {
render: ({ count, position }) => {
return new google.maps.Marker({
position,
label: String(count),
icon: {
url: 'path/to/custom/icon.png',
scaledSize: new google.maps.Size(50, 50)
}
});
}
}
});
Cluster Click Event
This feature allows you to add an event listener for cluster clicks. When a cluster is clicked, you can access the markers within that cluster and perform custom actions.
const map = new google.maps.Map(document.getElementById('map'), {
center: { lat: -28.024, lng: 140.887 },
zoom: 4,
});
const markers = locations.map((location, i) => {
return new google.maps.Marker({
position: location,
});
});
const markerCluster = new MarkerClusterer({
markers,
map
});
markerCluster.addListener('clusterclick', (cluster) => {
const markers = cluster.getMarkers();
console.log('Cluster clicked:', markers);
});
Other packages similar to @googlemaps/markerclusterer
leaflet.markercluster
leaflet.markercluster is a popular plugin for the Leaflet library that provides similar functionality for clustering markers on a map. It offers a variety of customization options and is widely used in the Leaflet community. Compared to @googlemaps/markerclusterer, it is designed specifically for Leaflet maps rather than Google Maps.
supercluster
supercluster is a fast, geospatial point clustering library for browsers and Node. It is highly efficient and can handle large datasets. Unlike @googlemaps/markerclusterer, supercluster is not tied to any specific mapping library and can be used with various mapping solutions.
Google Maps JavaScript MarkerClusterer

Description
The library creates and manages per-zoom-level clusters for large amounts of markers.
Try the demo
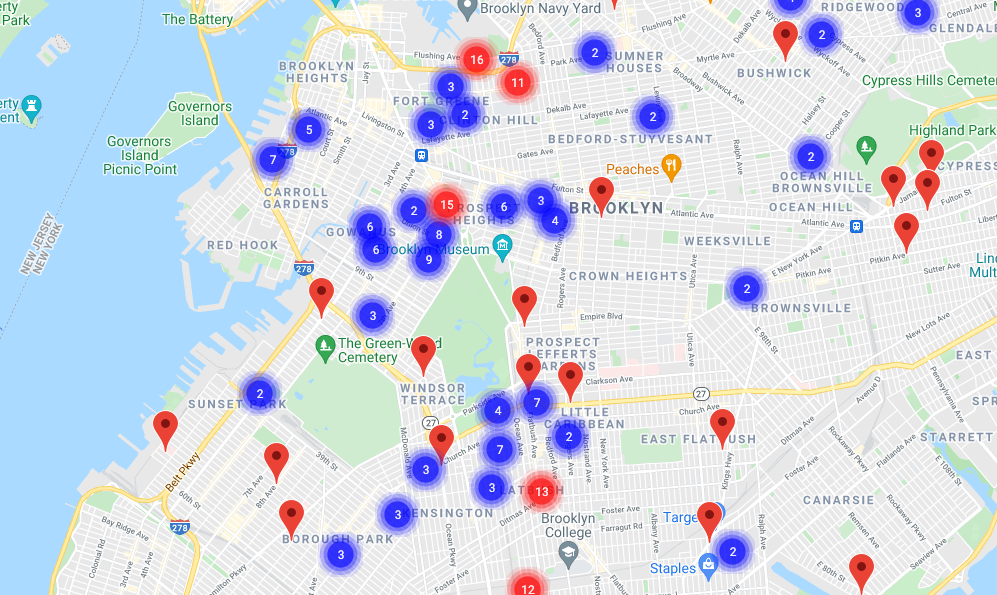
See the history section and migration section for how this library relates to @google/markerclusterer and @googlemaps/markerclustererplus.
Install
Available via npm as the package @googlemaps/markerclusterer.
npm i @googlemaps/markerclusterer
Alternativly you may add the umd package directly to the html document using the unpkg link.
<script src="https://unpkg.com/@googlemaps/markerclusterer/dist/index.min.js"></script>
When adding via unpkg, the MarkerClusterer
can be accessed at markerClusterer.MarkerClusterer
.
TypeScript
This library uses the official TypeScript typings for Google Maps Platform, @types/google.maps.
npm i -D @types/google.maps
Documentation
The reference documentation is generated from the TypeScript definitions.
Examples
import { MarkerClusterer } from "@googlemaps/markerclusterer";
const markerCluster = new MarkerClusterer({ map, markers });
View the package in action:
- Algorithm Comparisons - This example demonstrates the different algorithms. Please note that spacing and many other options can be changed for each algorithm.
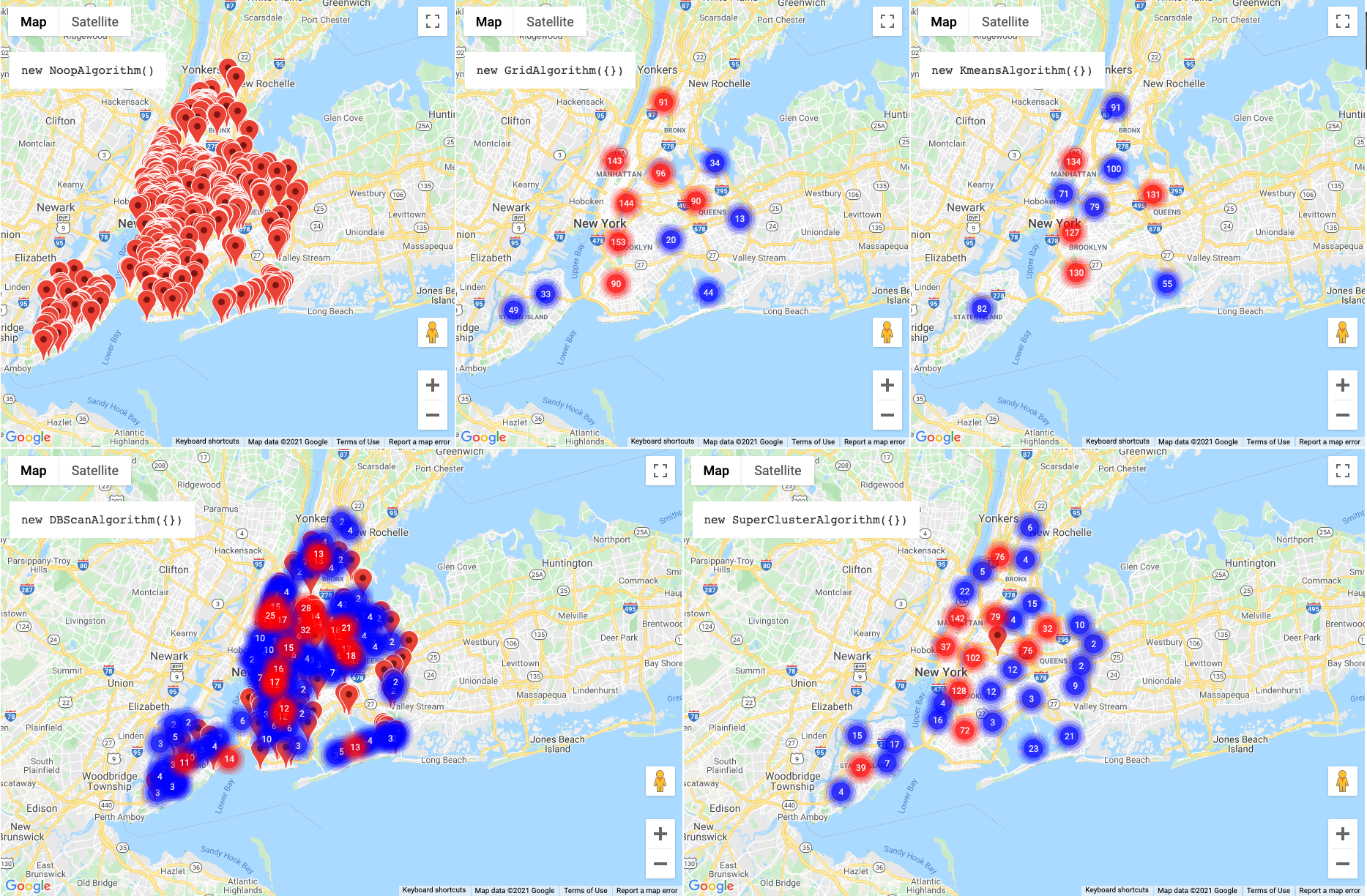
- Renderer Usage - This example demonstrates different renderers similar to the image below.
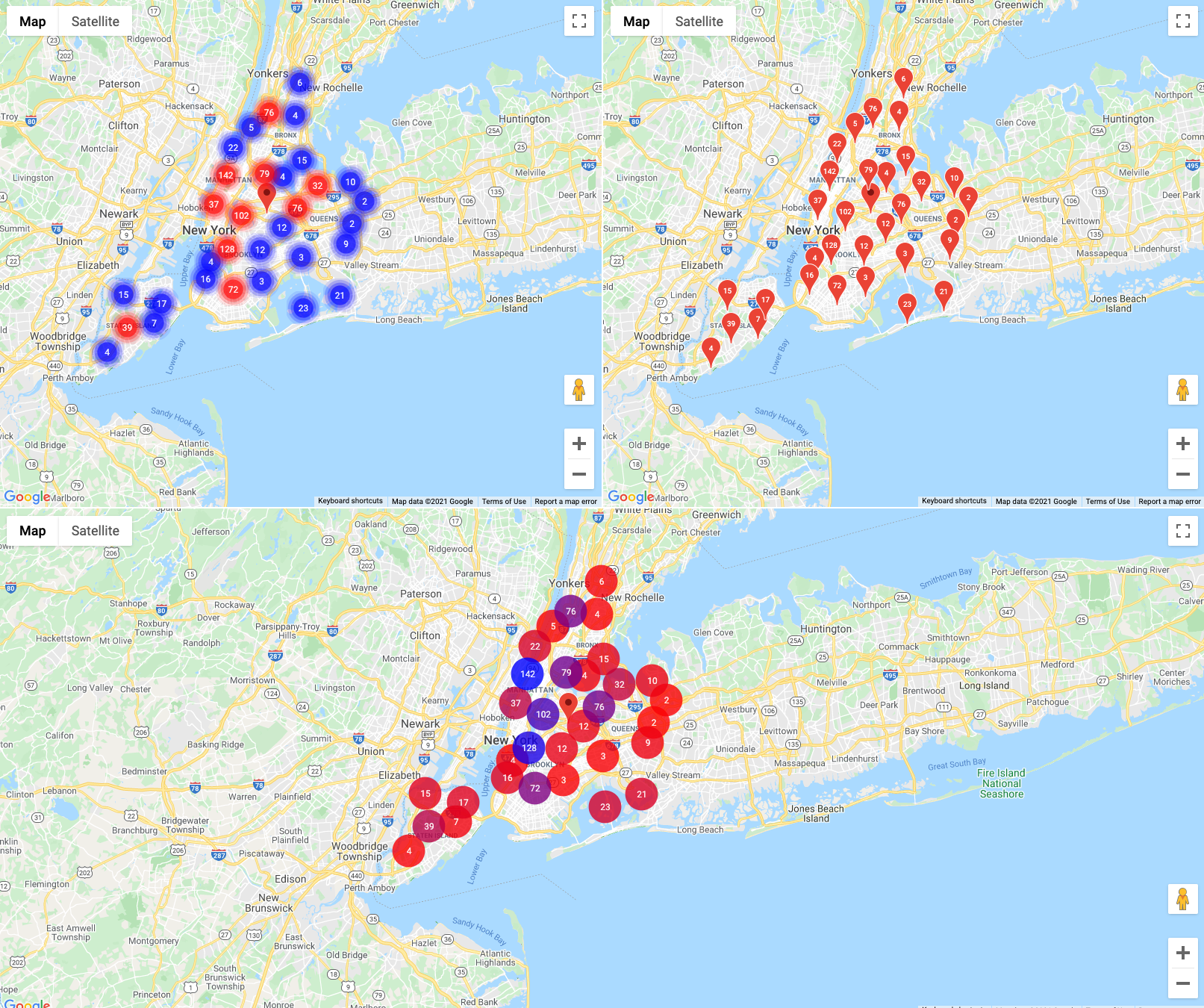
History
This library has a heritage in @google/markerclusterer and @googlemaps/markerclustererplus, originally made available on code.google.com and then transferred to GitHub at https://github.com/googlemaps/v3-utility-library. The following is an approximate timeline.
Migration
The API of @googlemaps/markerclustererplus has changed in a number of ways from @googlemaps/markerclustererplus.
- The
MarkerClusterer
class now accepts an algorithm
and renderer
parameter to allow for more flexibility. The interface looks like the following:
{
algorithm?: Algorithm;
map?: google.maps.Map;
markers?: google.maps.Marker[];
renderer?: Renderer;
onClusterClick?: onClusterClickHandler;
}
- The
MarkerClusterer
accepts a single options argument instead of positional parameters. - The traditional
GridAlgorithm
is still supported, but is not the default. The default is supercluster which uses k-d trees for improved performance. - Styling of clusters has been simplifed and moved to the renderer interface.
- The
MarkerClusterer
class is still an instance of google.maps.OverlayView
, but uses google.maps.Marker
s instead of google.maps.Overlay
to render the clusters. This solves issues related to the usage of map panes and click handlers. - @googlemaps/markerclusterer supports Marker and Map a11y improvements.