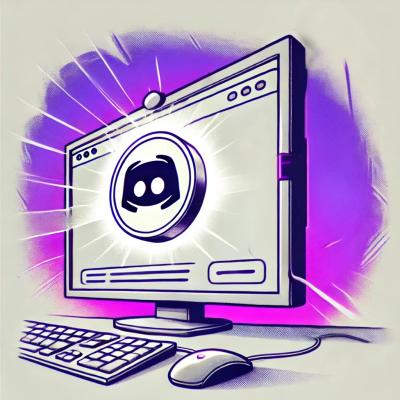
Research
Security News
Malicious PyPI Package ‘pycord-self’ Targets Discord Developers with Token Theft and Backdoor Exploit
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
@graphql-inspector/core
Advanced tools
Tooling for GraphQL. Compare GraphQL Schemas, check documents, find breaking changes, find similar types.
@graphql-inspector/core is a powerful tool for GraphQL schema management. It helps developers detect changes, validate schemas, and compare different versions of GraphQL schemas. This package is particularly useful for maintaining the integrity of GraphQL APIs over time.
Schema Comparison
This feature allows you to compare two GraphQL schemas and detect changes between them. The code sample demonstrates how to use the `diff` function to find differences between an old schema and a new schema.
const { diff } = require('@graphql-inspector/core');
const oldSchema = `type Query { hello: String }`;
const newSchema = `type Query { hello: String, goodbye: String }`;
const changes = diff(oldSchema, newSchema);
console.log(changes);
Schema Validation
This feature helps you validate a GraphQL schema to ensure it adheres to the GraphQL specification. The code sample shows how to use the `validate` function to check a schema for errors.
const { validate } = require('@graphql-inspector/core');
const schema = `type Query { hello: String }`;
const errors = validate(schema);
console.log(errors);
Schema Coverage
This feature allows you to check how well your GraphQL queries cover your schema. The code sample demonstrates how to use the `coverage` function to analyze the coverage of a schema based on provided documents.
const { coverage } = require('@graphql-inspector/core');
const schema = `type Query { hello: String }`;
const documents = [{ content: `{ hello }` }];
const result = coverage(schema, documents);
console.log(result);
The `graphql` package is the reference implementation of GraphQL for JavaScript. It provides the core functionality for building GraphQL schemas and executing queries. While it does not offer schema comparison or validation out of the box, it is a fundamental building block for any GraphQL-related tool.
The `graphql-tools` package provides a set of utilities for building and manipulating GraphQL schemas. It includes features for schema stitching, mocking, and schema transformations. While it overlaps with some of the functionality of @graphql-inspector/core, it is more focused on schema construction and manipulation rather than inspection and validation.
The `apollo-server` package is a popular GraphQL server implementation that includes tools for schema creation, query execution, and performance monitoring. It provides some validation and schema management features, but its primary focus is on serving GraphQL APIs rather than inspecting and comparing schemas.
GraphQL Inspector outputs a list of changes between two GraphQL schemas. Every change is precisely explained and marked as breaking, non-breaking or dangerous. It helps you validate documents and fragments against a schema and even find similar or duplicated types.
Major features:
GraphQL Inspector has a CLI and also a programmatic API, so you can use it however you want to and even build tools on top of it.
pnpm add @graphql-inspector/core
import {
diff,
validate,
similar,
coverage,
Change,
InvalidDocument,
SimilarMap,
SchemaCoverage
} from '@graphql-inspector/core'
// diff
const changes: Change[] = diff(schemaA, schemaB)
// validate
const invalid: InvalidDocument[] = validate(documentsGlob, schema)
// similar
const similar: SimilarMap = similar(schema, typename, threshold)
// coverage
const schemaCoverage: SchemaCoverage = coverage(schema, documents)
// ...
MIT © Kamil Kisiela
FAQs
Tooling for GraphQL. Compare GraphQL Schemas, check documents, find breaking changes, find similar types.
The npm package @graphql-inspector/core receives a total of 234,335 weekly downloads. As such, @graphql-inspector/core popularity was classified as popular.
We found that @graphql-inspector/core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.