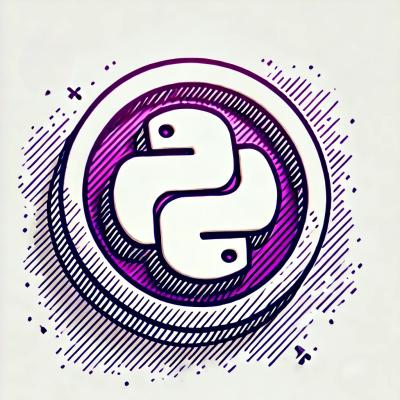
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
@graphql-tools/stitch
Advanced tools
@graphql-tools/stitch is a part of the GraphQL Tools ecosystem that allows you to combine multiple GraphQL schemas into a single unified schema. This is particularly useful for microservices architectures where each service has its own GraphQL schema, and you want to expose a single GraphQL endpoint to clients.
Schema Stitching
This feature allows you to combine multiple GraphQL schemas into a single unified schema. The code sample demonstrates how to stitch together a user schema and a product schema.
const { stitchSchemas } = require('@graphql-tools/stitch');
const { makeExecutableSchema } = require('@graphql-tools/schema');
const userSchema = makeExecutableSchema({
typeDefs: `
type User {
id: ID!
name: String!
}
type Query {
user(id: ID!): User
}
`,
resolvers: {
Query: {
user: () => ({ id: '1', name: 'John Doe' })
}
}
});
const productSchema = makeExecutableSchema({
typeDefs: `
type Product {
id: ID!
name: String!
}
type Query {
product(id: ID!): Product
}
`,
resolvers: {
Query: {
product: () => ({ id: '1', name: 'Laptop' })
}
}
});
const stitchedSchema = stitchSchemas({
subschemas: [
{ schema: userSchema },
{ schema: productSchema }
]
});
console.log(stitchedSchema.getTypeMap());
Schema Delegation
Schema delegation allows you to forward a query from one schema to another. The code sample demonstrates how to delegate a query to the stitched schema to fetch user data.
const { delegateToSchema } = require('@graphql-tools/stitch');
const { makeExecutableSchema } = require('@graphql-tools/schema');
const userSchema = makeExecutableSchema({
typeDefs: `
type User {
id: ID!
name: String!
}
type Query {
user(id: ID!): User
}
`,
resolvers: {
Query: {
user: () => ({ id: '1', name: 'John Doe' })
}
}
});
const productSchema = makeExecutableSchema({
typeDefs: `
type Product {
id: ID!
name: String!
}
type Query {
product(id: ID!): Product
}
`,
resolvers: {
Query: {
product: () => ({ id: '1', name: 'Laptop' })
}
}
});
const stitchedSchema = stitchSchemas({
subschemas: [
{ schema: userSchema },
{ schema: productSchema }
]
});
const result = delegateToSchema({
schema: stitchedSchema,
operation: 'query',
fieldName: 'user',
args: { id: '1' }
});
result.then(data => console.log(data));
Schema Merging
Schema merging allows you to combine multiple schemas into one. The code sample demonstrates how to merge a user schema and a product schema into a single schema.
const { mergeSchemas } = require('@graphql-tools/merge');
const { makeExecutableSchema } = require('@graphql-tools/schema');
const userSchema = makeExecutableSchema({
typeDefs: `
type User {
id: ID!
name: String!
}
type Query {
user(id: ID!): User
}
`,
resolvers: {
Query: {
user: () => ({ id: '1', name: 'John Doe' })
}
}
});
const productSchema = makeExecutableSchema({
typeDefs: `
type Product {
id: ID!
name: String!
}
type Query {
product(id: ID!): Product
}
`,
resolvers: {
Query: {
product: () => ({ id: '1', name: 'Laptop' })
}
}
});
const mergedSchema = mergeSchemas({
schemas: [userSchema, productSchema]
});
console.log(mergedSchema.getTypeMap());
Apollo Server is a community-maintained open-source GraphQL server that works with any GraphQL schema. It provides a straightforward setup for creating a GraphQL server and supports schema stitching through its federation capabilities. Compared to @graphql-tools/stitch, Apollo Server is more focused on providing a complete server solution with additional features like caching, monitoring, and more.
GraphQL Yoga is a fully-featured GraphQL server with focus on easy setup, performance, and great developer experience. It supports schema stitching and merging, similar to @graphql-tools/stitch, but also includes built-in support for subscriptions and other advanced features. GraphQL Yoga is designed to be a more opinionated and feature-rich alternative to setting up a GraphQL server from scratch.
merge-graphql-schemas is a utility library for merging GraphQL schemas. It provides a simple API for combining multiple schema files into a single executable schema. While it offers similar functionality to @graphql-tools/stitch in terms of schema merging, it is more lightweight and focused solely on the merging aspect without additional features like schema delegation.
Check API Reference for more information about this package; https://www.graphql-tools.com/docs/api/modules/stitch_src
You can also learn more about Schema Stitching in this chapter; https://www.graphql-tools.com/docs/stitch-combining-schemas
FAQs
A set of utils for faster development of GraphQL tools
The npm package @graphql-tools/stitch receives a total of 0 weekly downloads. As such, @graphql-tools/stitch popularity was classified as not popular.
We found that @graphql-tools/stitch demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.