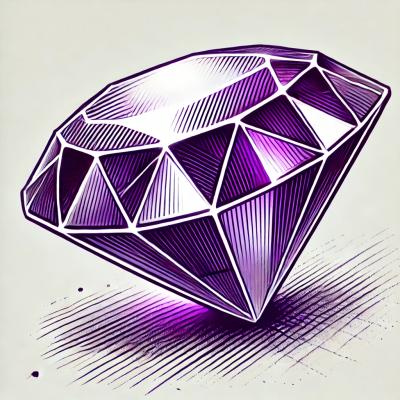
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@griffel/react
Advanced tools
@griffel/react is a CSS-in-JS library designed to provide a performant and flexible way to style React components. It focuses on runtime performance and developer experience, offering features like atomic CSS, theming, and server-side rendering support.
Atomic CSS
Griffel generates atomic CSS classes for each style rule, ensuring minimal CSS output and better performance.
const useStyles = makeStyles({
root: { color: 'red' },
button: { backgroundColor: 'blue' }
});
function MyComponent() {
const classes = useStyles();
return (
<div className={classes.root}>
<button className={classes.button}>Click me</button>
</div>
);
}
Theming
Griffel supports theming, allowing you to define and use theme variables throughout your application.
const theme = {
color: 'blue',
background: 'lightgray'
};
const useStyles = makeStyles({
root: theme => ({
color: theme.color,
backgroundColor: theme.background
})
});
function MyComponent() {
const classes = useStyles(theme);
return <div className={classes.root}>Themed Component</div>;
}
Server-Side Rendering
Griffel supports server-side rendering, ensuring that styles are correctly rendered on the server and sent to the client.
import { renderToString } from 'react-dom/server';
import { GriffelProvider, makeStyles } from '@griffel/react';
const useStyles = makeStyles({
root: { color: 'red' }
});
function MyComponent() {
const classes = useStyles();
return <div className={classes.root}>Hello SSR</div>;
}
const html = renderToString(
<GriffelProvider>
<MyComponent />
</GriffelProvider>
);
styled-components is a popular CSS-in-JS library that allows you to write actual CSS to style your components. It offers features like theming, automatic critical CSS, and server-side rendering. Compared to @griffel/react, styled-components focuses more on developer experience with a more expressive syntax but may have larger runtime overhead.
Emotion is another CSS-in-JS library that provides powerful and flexible styling capabilities. It offers both string and object styles, theming, and server-side rendering. Emotion is known for its performance and flexibility, making it a strong competitor to @griffel/react.
npm install @griffel/react
# or
yarn add @griffel/react
makeStyles()
Is used to define styles, returns a React hook that should be called inside a component:
import { makeStyles } from '@griffel/react';
const useClasses = makeStyles({
button: { color: 'red' },
icon: { paddingLeft: '5px' },
});
function Component() {
const classes = useClasses();
return (
<div>
<button className={classes.button} />
<span className={classes.icon} />
</div>
);
}
mergeClasses()
💡 It is not possible to simply concatenate classes returned by
useClasses()
.
There are cases where you need to merge classes from multiple useClasses
calls.
To properly merge the classes, you need to use mergeClasses()
function, which performs merge and deduplication of atomic classes generated by makeStyles()
.
import { mergeClasses, makeStyles } from '@griffel/react';
const useClasses = makeStyles({
blueBold: {
color: 'blue',
fontWeight: 'bold',
},
red: {
color: 'red',
},
});
function Component() {
const classes = useClasses();
const firstClassName = mergeClasses(classes.blueBold, classes.red); // { color: 'red', fontWeight: 'bold' }
const secondClassName = mergeClasses(classes.red, classes.blueBold); // { color: 'blue', fontWeight: 'bold' }
return (
<>
<div className={firstClassName} />
<div className={secondClassName} />
</>
);
}
shorthands
shorthands
provides a set of functions to mimic CSS shorthands and improve developer experience as CSS shorthands are not supported by Griffel.
shorthands.border
import { makeStyles, shorthands } from '@griffel/react';
const useClasses = makeStyles({
root: {
...shorthands.border('2px'),
...shorthands.border('2px', 'solid'),
...shorthands.border('2px', 'solid', 'red'),
},
});
shorthands.borderBottom
, shorthands.borderTop
, shorthands.borderLeft
, shorthands.borderRight
import { makeStyles, shorthands } from '@griffel/react';
const useClasses = makeStyles({
root: {
// The same is true for "borderTop", "borderLeft" & "borderRight"
...shorthands.borderBottom('2px'),
...shorthands.borderBottom('2px', 'solid'),
...shorthands.borderBottom('2px', 'solid', 'red'),
},
});
shorthands.borderColor
import { makeStyles, shorthands } from '@griffel/react';
const useClasses = makeStyles({
root: {
...shorthands.borderColor('red'),
...shorthands.borderColor('red', 'blue'),
...shorthands.borderColor('red', 'blue', 'green'),
...shorthands.borderColor('red', 'blue', 'green', 'yellow'),
},
});
shorthands.borderStyle
import { makeStyles, shorthands } from '@griffel/react';
const useClasses = makeStyles({
root: {
...shorthands.borderStyle('solid'),
...shorthands.borderStyle('solid', 'dashed'),
...shorthands.borderStyle('solid', 'dashed', 'dotted'),
...shorthands.borderStyle('solid', 'dashed', 'dotted', 'double'),
},
});
shorthands.borderWidth
import { makeStyles, shorthands } from '@griffel/react';
const useClasses = makeStyles({
root: {
...shorthands.borderWidth('12px'),
...shorthands.borderWidth('12px', '24px'),
...shorthands.borderWidth('12px', '24px', '36px'),
...shorthands.borderWidth('12px', '24px', '36px', '48px'),
},
});
shorthands.gap
import { makeStyles, shorthands } from '@griffel/react';
const useClasses = makeStyles({
root: {
...shorthands.gap('12px'),
...shorthands.gap('12px', '24px'),
},
});
shorthands.margin
import { makeStyles, shorthands } from '@griffel/react';
const useClasses = makeStyles({
root: {
...shorthands.margin('12px'),
...shorthands.margin('12px', '24px'),
...shorthands.margin('12px', '24px', '36px'),
...shorthands.margin('12px', '24px', '36px', '48px'),
},
});
shorthands.overflow
import { makeStyles, shorthands } from '@griffel/react';
const useClasses = makeStyles({
root: {
...shorthands.overflow('visible'),
...shorthands.overflow('visible', 'hidden'),
},
});
shorthands.padding
import { makeStyles, shorthands } from '@griffel/react';
const useClasses = makeStyles({
root: {
...shorthands.padding('12px'),
...shorthands.padding('12px', '24px'),
...shorthands.padding('12px', '24px', '36px'),
...shorthands.padding('12px', '24px', '36px', '48px'),
},
});
makeStaticStyles()
Creates styles attached to a global selector. Styles can be defined via objects:
import { makeStaticStyles } from '@griffel/react';
const useStaticStyles = makeStaticStyles({
'@font-face': {
fontFamily: 'Open Sans',
src: `url("/fonts/OpenSans-Regular-webfont.woff2") format("woff2"),
url("/fonts/OpenSans-Regular-webfont.woff") format("woff")`,
},
body: {
background: 'red',
},
/**
* ⚠️ nested and pseudo selectors are not supported for this scenario via nesting
*
* Not supported:
* .some {
* .class { ... },
* ':hover': { ... }
* }
*
* Supported:
* '.some.class': { ... }
* '.some.class:hover': { ... }
*/
});
function App() {
useStaticStyles();
return <div />;
}
Or with string & arrays of strings/objects:
import { makeStaticStyles } from '@griffel/react';
const useStaticStyles1 = makeStaticStyles('body { background: red; } .foo { color: green; }');
const useStaticStyles2 = makeStaticStyles([
{
'@font-face': {
fontFamily: 'My Font',
src: `url(my_font.woff)`,
},
},
'html { line-height: 20px; }',
]);
function App() {
useStaticStyles1();
useStaticStyles2();
return <div />;
}
createDOMRenderer()
, RendererProvider
createDOMRenderer
is paired with RendererProvider
component and is useful for child window rendering and SSR scenarios. This is the default renderer for web, and will make sure that styles are injected to a document.
import { createDOMRenderer, RendererProvider } from '@griffel/react';
function App(props) {
const { targetDocument } = props;
const renderer = React.useMemo(() => createDOMRenderer(targetDocument), [targetDocument]);
return (
<RendererProvider renderer={renderer} targetDocument={targetDocument}>
{/* Children components */}
{/* ... */}
</RendererProvider>
);
}
A map of attributes that's passed to the generated style elements. For example, is useful to set "nonce" attribute.
import { createDOMRenderer } from '@griffel/react';
const renderer = createDOMRenderer(targetDocument, {
styleElementAttributes: {
nonce: 'random',
},
});
makeStyles()
supports pseudo, class selectors and at-rules.
import { makeStyles } from '@griffel/react';
const useClasses = makeStyles({
root: {
':active': { color: 'pink' },
':hover': { color: 'blue' },
// :link, :focus, etc.
'.foo': { color: 'black' },
':nth-child(2n)': { backgroundColor: '#fafafa' },
'@media screen and (max-width: 992px)': { color: 'orange' },
'@supports (display: grid)': { color: 'red' },
'@layer utility': { marginBottom: '1em' }
},
});
Another useful feature is :global()
selector, it allows connecting local styles with global selectors.
import { makeStyles } from '@griffel/react';
const useClasses = makeStyles({
root: {
':global(html[data-whatintent="mouse"])': { backgroundColor: 'yellow' },
// outputs: html[data-whatintent="mouse"] .abcd { background-color: yellow }
},
});
keyframes
(animations)keyframes
are supported via animationName
property that can be defined as an object or an array of objects:
import { makeStyles } from '@griffel/react';
const useClasses = makeStyles({
root: {
animationIterationCount: 'infinite',
animationDuration: '3s',
animationName: {
from: { transform: 'rotate(0deg)' },
to: { transform: 'rotate(360deg)' },
},
},
array: {
animationIterationCount: 'infinite',
animationDuration: '3s',
animationName: [
{
from: { transform: 'rotate(0deg)' },
to: { transform: 'rotate(360deg)' },
},
{
from: { height: '100px' },
to: { height: '200px' },
},
],
},
});
Any CSS property accepts an array of values which are all added to the styles. Every browser will use the latest valid value (which might be a different one in different browsers, based on supported CSS in that browser):
import { makeStyles } from '@griffel/react';
const useClasses = makeStyles({
root: {
overflowY: ['scroll', 'overlay'],
},
});
FAQs
React implementation of Atomic CSS-in-JS
The npm package @griffel/react receives a total of 60,234 weekly downloads. As such, @griffel/react popularity was classified as popular.
We found that @griffel/react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.