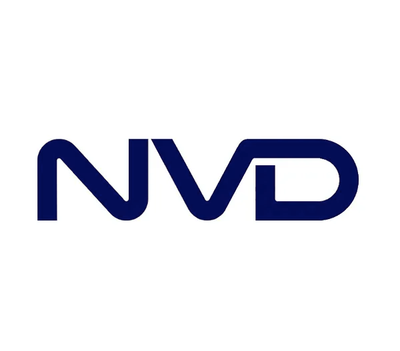
Security News
NVD Backlog Tops 20,000 CVEs Awaiting Analysis as NIST Prepares System Updates
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
@handy-common-utils/misc-utils
Advanced tools
Miscellaneous utilities
First add it as a dependency:
npm install @handy-common-utils/misc-utils
Then you can use it in the code:
import { shortBase64UrlFromUInt32 } from '@handy-common-utils/misc-utils';
const urlSafeBase64 = shortBase64UrlFromUInt32(12345);
// use chalk (chalk is not a dependency of this package, you need to add chalk as a dependency separately)
import chalk from 'chalk';
import { LineLogger } from '@handy-common-utils/misc-utils';
// this.flags is an object with properties "debug" and "quiet"
this.output = LineLogger.consoleWithColour(this.flags, chalk);
this.output.warn('Configuration file not found, default configuration would be used.'); // it would be printed out in yellow
line-logger.LineLogger
A LineLogger logs/prints one entire line of text before advancing to another line. This class is useful for encapsulating console.log/info/warn/error functions. By having an abstraction layer, your code can switching to a different output with nearly no change.
Please note that although the name contains "Logger", this class is not intended to be used as a generic logger. It is intended for "logging for humans to read" scenario.
LineLogger.console()
and LineLogger.consoleWithColour()
are ready to use convenient functions.
Or you can use the constructor to build your own wrappers.
example
// Just a wrapper of console.log/info/warn/error
const consoleLogger = LineLogger.console();
// Wrapper of console.log/info/warn/error but it mutes console.log
const lessVerboseConsoleLogger = LineLogger.console({debug: false});
// Wrapper of console.log/info/warn/error but it mutes console.log and console.info
const lessVerboseConsoleLogger = LineLogger.console({quiet: true});
// use chalk (chalk is not a dependency of this package, you need to add chalk as a dependency separately)
import chalk from 'chalk';
// this.flags is an object with properties "debug" and "quiet"
this.output = LineLogger.consoleWithColour(this.flags, chalk);
this.output.warn('Configuration file not found, default configuration would be used.'); // it would be printed out in yellow
Name | Type |
---|---|
DEBUG_FUNC | extends Function |
INFO_FUNC | extends Function |
WARN_FUNC | extends Function |
ERROR_FUNC | extends Function |
• new LineLogger<DEBUG_FUNC
, INFO_FUNC
, WARN_FUNC
, ERROR_FUNC
>(debugFunction
, infoFunction
, warnFunction
, errorFunction
, isDebug?
, isQuiet?
)
Constructor
Name | Type |
---|---|
DEBUG_FUNC | extends Function |
INFO_FUNC | extends Function |
WARN_FUNC | extends Function |
ERROR_FUNC | extends Function |
Name | Type | Default value | Description |
---|---|---|---|
debugFunction | DEBUG_FUNC | undefined | function for outputting debug information |
infoFunction | INFO_FUNC | undefined | function for outputting info information |
warnFunction | WARN_FUNC | undefined | function for outputting warn information |
errorFunction | ERROR_FUNC | undefined | function for outputting error information |
isDebug | boolean | false | is debug output enabled or not, it could be overriden by isQuiet |
isQuiet | boolean | false | is quiet mode enabled or not. When quiet mode is enabled, both debug and info output would be discarded. |
Property | Description |
---|---|
• debug: DEBUG_FUNC | |
• error: ERROR_FUNC | |
• info: INFO_FUNC | |
• isDebug: boolean = false | |
• isQuiet: boolean = false | |
• warn: WARN_FUNC | |
▪ Static Protected NO_OP_FUNC: () => void | Type declaration: ▸ (): void Returns: void |
▸ Static
console<FLAGS
>(flags?
, debugFlagName?
, quietFlagName?
): LineLogger
<(message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
>
Build an instance with console.log/info/warn/error.
Name | Type |
---|---|
FLAGS | extends Record <string , any > |
Name | Type | Default value | Description |
---|---|---|---|
flags | FLAGS | undefined | The flag object that contains fields for knowning whether debug is enabled and whether quiet mode is enabled. Values of those fields are evaluated only once within this function. They are not evaluated when debug/info/warn/error functions are called. |
debugFlagName | keyof FLAGS | 'debug' | Name of the debug field in the flags object |
quietFlagName | keyof FLAGS | 'quiet' | Name of the quiet field in the flags object |
LineLogger
<(message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
>
An instance that uses console.log/info/warn/error.
▸ Static
consoleLike(log
): LineLogger
<(message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
>
Build an instance from 'log' (https://github.com/medikoo/log).
info
of the LineLogger is mapped to notice
of the medikoo log.
Name | Type | Description |
---|---|---|
log | Logger | instance of the logger |
LineLogger
<(message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
>
instance of LineLogger that is actually ConsoleLineLogger type
▸ Static
consoleWithColour<FLAGS
, COLOURER
>(flags
, colourer
, debugColourFuncName?
, infoColourFuncName?
, warnColourFuncName?
, errorColourFuncName?
, debugFlagName?
, quietFlagName?
): LineLogger
<(message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
>
Build an instance with console.log/info/warn/error and chalk/colors/cli-color. This package does not depend on chalk or colors or cli-color, you need to add them as dependencies separately.
Name | Type |
---|---|
FLAGS | extends Record <string , any > |
COLOURER | extends Record <string , any > |
Name | Type | Default value | Description |
---|---|---|---|
flags | FLAGS | undefined | The flag object that contains fields for knowning whether debug is enabled and whether quiet mode is enabled. Values of those fields are evaluated only once within this function. They are not evaluated when debug/info/warn/error functions are called. |
colourer | COLOURER | undefined | Supplier of the colouring function, such as chalk or colors or cli-color |
debugColourFuncName | keyof COLOURER | 'grey' | Name of the function within colourer that will be used to add colour to debug messages, or null if colouring is not desired. |
infoColourFuncName? | keyof COLOURER | undefined | Name of the function within colourer that will be used to add colour to info messages, or null if colouring is not desired. |
warnColourFuncName | keyof COLOURER | 'yellow' | Name of the function within colourer that will be used to add colour to warn messages, or null if colouring is not desired. |
errorColourFuncName | keyof COLOURER | 'red' | Name of the function within colourer that will be used to add colour to error messages, or null if colouring is not desired. |
debugFlagName | keyof FLAGS | 'debug' | Name of the debug field in the flags object |
quietFlagName | keyof FLAGS | 'quiet' | Name of the quiet field in the flags object |
LineLogger
<(message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
>
An instance that uses console.log/info/warn/error and also adds colour to the messages using chalk/colors/cli-color.
Re-exports ConsoleLineLogger
Re-exports LineLogger
Re-exports consoleLike
Re-exports consoleWithColour
Re-exports consoleWithoutColour
▸ base64FromUInt32<T
>(ui32
): Exclude
<T
, number
> | string
Encode an unsigned 32-bit integer into BASE64 string.
Name | Type |
---|---|
T | extends undefined | null | number |
Name | Type | Description |
---|---|---|
ui32 | T | A 32-bit integer number which could also be null or undefined. It must be a valid unsigned 32-bit integer. Behavior is undefined when valueis anything other than an unsigned 32-bit integer. If you don't care about loosing precision, you can convert a number by doing n >>> 0 (See https://stackoverflow.com/questions/22335853/hack-to-convert-javascript-number-to-uint32) |
Exclude
<T
, number
> | string
BASE64 string representing the integer input, or the original input if it is null or undefined.
▸ base64UrlFromUInt32<T
>(ui32
, replacements?
): Exclude
<T
, number
> | string
Encode an unsigned 32-bit integer into URL/path safe BASE64 string.
Name | Type |
---|---|
T | extends undefined | null | number |
Name | Type | Default value | Description |
---|---|---|---|
ui32 | T | undefined | A 32-bit integer number which could also be null or undefined. It must be a valid unsigned 32-bit integer. Behavior is undefined when valueis anything other than an unsigned 32-bit integer. If you don't care about loosing precision, you can convert a number by doing n >>> 0 (See https://stackoverflow.com/questions/22335853/hack-to-convert-javascript-number-to-uint32) |
replacements | string | '_-=' | A string containing replacement characters for "/", "+", and "=". If omitted, default value of '_-=' would be used. |
Exclude
<T
, number
> | string
URL/path safe BASE64 string representing the integer input, or the original input if it is null or undefined.
▸ shortBase64FromUInt32<T
>(ui32
): Exclude
<T
, number
> | string
Encode an unsigned 32-bit integer into BASE64 string without trailing '='.
Name | Type |
---|---|
T | extends undefined | null | number |
Name | Type | Description |
---|---|---|
ui32 | T | A 32-bit integer number which could also be null or undefined. It must be a valid unsigned 32-bit integer. Behavior is undefined when valueis anything other than an unsigned 32-bit integer. If you don't care about loosing precision, you can convert a number by doing n >>> 0 (See https://stackoverflow.com/questions/22335853/hack-to-convert-javascript-number-to-uint32) |
Exclude
<T
, number
> | string
BASE64 string without trailing '=' representing the integer input, or the original input if it is null or undefined.
▸ shortBase64UrlFromUInt32<T
>(ui32
, replacements?
): Exclude
<T
, number
> | string
Encode an unsigned 32-bit integer into URL/path safe BASE64 string without trailling '='.
Name | Type |
---|---|
T | extends undefined | null | number |
Name | Type | Default value | Description |
---|---|---|---|
ui32 | T | undefined | A 32-bit integer number which could also be null or undefined. It must be a valid unsigned 32-bit integer. Behavior is undefined when valueis anything other than an unsigned 32-bit integer. If you don't care about loosing precision, you can convert a number by doing n >>> 0 (See https://stackoverflow.com/questions/22335853/hack-to-convert-javascript-number-to-uint32) |
replacements | string | '_-' | A string containing replacement characters for "/" and "+". If omitted, default value of '_-' would be used. |
Exclude
<T
, number
> | string
URL/path safe BASE64 string without trailing '=' representing the integer input, or the original input if it is null or undefined.
▸ urlSafe<T
>(base64Input
, replacements?
): T
Make a "normal" (BASE64) string URL/path safe.
Name | Type |
---|---|
T | extends undefined | null | string |
Name | Type | Default value | Description |
---|---|---|---|
base64Input | T | undefined | A (BASE64) string which could be null or undefined. |
replacements | string | '_-=' | A string containing replacement characters for "/", "+", and "=". If omitted, default value of '_-=' would be used. |
T
URL/path safe version of the (BASE64) input string, or the original input if it is null or undefined.
Ƭ ConsoleLineLogger: ReturnType
<typeof console
>
Type of the object returned by LineLogger.console()
or LineLogger.consoleWithColour()
.
It has the same function signatures as console.log/info/warn/error.
▸ consoleLike(log
): LineLogger
<(message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
>
Build an instance from 'log' (https://github.com/medikoo/log).
info
of the LineLogger is mapped to notice
of the medikoo log.
Name | Type | Description |
---|---|---|
log | Logger | instance of the logger |
LineLogger
<(message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
>
instance of LineLogger that is actually ConsoleLineLogger type
▸ consoleWithColour<FLAGS
, COLOURER
>(flags
, colourer
, debugColourFuncName?
, infoColourFuncName?
, warnColourFuncName?
, errorColourFuncName?
, debugFlagName?
, quietFlagName?
): LineLogger
<(message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
>
Build an encapsulation of console output functions with console.log/info/warn/error and chalk/colors/cli-color.
Name | Type |
---|---|
FLAGS | extends Record <string , any > |
COLOURER | extends Record <string , any > |
Name | Type | Default value | Description |
---|---|---|---|
flags | FLAGS | undefined | The flag object that contains fields for knowning whether debug is enabled and whether quiet mode is enabled. Values of those fields are evaluated only once within this function. They are not evaluated when debug/info/warn/error functions are called. |
colourer | COLOURER | undefined | Supplier of the colouring function, such as chalk or colors or cli-color |
debugColourFuncName | keyof COLOURER | 'grey' | Name of the function within colourer that will be used to add colour to debug messages, or null if colouring is not desired. |
infoColourFuncName? | keyof COLOURER | undefined | Name of the function within colourer that will be used to add colour to info messages, or null if colouring is not desired. |
warnColourFuncName | keyof COLOURER | 'yellow' | Name of the function within colourer that will be used to add colour to warn messages, or null if colouring is not desired. |
errorColourFuncName | keyof COLOURER | 'red' | Name of the function within colourer that will be used to add colour to error messages, or null if colouring is not desired. |
debugFlagName | keyof FLAGS | 'debug' | Name of the debug field in the flags object |
quietFlagName | keyof FLAGS | 'quiet' | Name of the quiet field in the flags object. Quiet flag can override debug flag. |
LineLogger
<(message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
>
An LineLogger instance that uses console.log/info/warn/error and also adds colour to the messages using chalk/colors/cli-color.
▸ consoleWithoutColour<FLAGS
>(flags?
, debugFlagName?
, quietFlagName?
): LineLogger
<(message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
>
Build an encapsulation of console output functions with console.log/info/warn/error.
Name | Type |
---|---|
FLAGS | extends Record <string , any > |
Name | Type | Default value | Description |
---|---|---|---|
flags | FLAGS | undefined | The flag object that contains fields for knowning whether debug is enabled and whether quiet mode is enabled. Values of those fields are evaluated only once within this function. They are not evaluated when debug/info/warn/error functions are called. |
debugFlagName | keyof FLAGS | 'debug' | Name of the debug field in the flags object |
quietFlagName | keyof FLAGS | 'quiet' | Name of the quiet field in the flags object. Quiet flag can override debug flag. |
LineLogger
<(message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
, (message?
: any
, ...optionalParams
: any
[]) => void
>
An LineLogger instance that uses console.log/info/warn/error.
FAQs
Miscellaneous utilities
The npm package @handy-common-utils/misc-utils receives a total of 90 weekly downloads. As such, @handy-common-utils/misc-utils popularity was classified as not popular.
We found that @handy-common-utils/misc-utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.