What is @hapi/accept?
@hapi/accept is a utility library for HTTP content negotiation. It helps in parsing and matching HTTP headers like Accept, Accept-Charset, Accept-Encoding, and Accept-Language, allowing you to determine the best content to serve based on the client's preferences.
What are @hapi/accept's main functionalities?
Parsing Accept Header
This feature allows you to parse the 'Accept' header from an HTTP request and get an array of media types sorted by their quality values.
const Accept = require('@hapi/accept');
const headers = { 'accept': 'text/html, application/json;q=0.9, */*;q=0.8' };
const mediaTypes = Accept.mediaTypes(headers.accept);
console.log(mediaTypes); // ['text/html', 'application/json', '*/*']
Parsing Accept-Language Header
This feature allows you to parse the 'Accept-Language' header and get an array of languages sorted by their quality values.
const Accept = require('@hapi/accept');
const headers = { 'accept-language': 'en-US,en;q=0.9,fr;q=0.8' };
const languages = Accept.languages(headers['accept-language']);
console.log(languages); // ['en-US', 'en', 'fr']
Parsing Accept-Charset Header
This feature allows you to parse the 'Accept-Charset' header and get an array of charsets sorted by their quality values.
const Accept = require('@hapi/accept');
const headers = { 'accept-charset': 'utf-8, iso-8859-1;q=0.5' };
const charsets = Accept.charsets(headers['accept-charset']);
console.log(charsets); // ['utf-8', 'iso-8859-1']
Parsing Accept-Encoding Header
This feature allows you to parse the 'Accept-Encoding' header and get an array of encodings sorted by their quality values.
const Accept = require('@hapi/accept');
const headers = { 'accept-encoding': 'gzip, deflate;q=0.8, br;q=0.6' };
const encodings = Accept.encodings(headers['accept-encoding']);
console.log(encodings); // ['gzip', 'deflate', 'br']
Matching Media Types
This feature allows you to match the best media type from a list of available types based on the 'Accept' header.
const Accept = require('@hapi/accept');
const headers = { 'accept': 'text/html, application/json;q=0.9, */*;q=0.8' };
const mediaType = Accept.mediaType(headers.accept, ['application/json', 'text/html']);
console.log(mediaType); // 'text/html'
Other packages similar to @hapi/accept
negotiator
Negotiator is a library for HTTP content negotiation. It provides similar functionalities to @hapi/accept, such as parsing and matching Accept, Accept-Charset, Accept-Encoding, and Accept-Language headers. However, @hapi/accept is part of the hapi ecosystem and may have better integration with other hapi modules.
content-type
Content-Type is a library for parsing and formatting media types. While it does not provide the full range of content negotiation features like @hapi/accept, it is useful for handling media type parsing and formatting in a more lightweight manner.
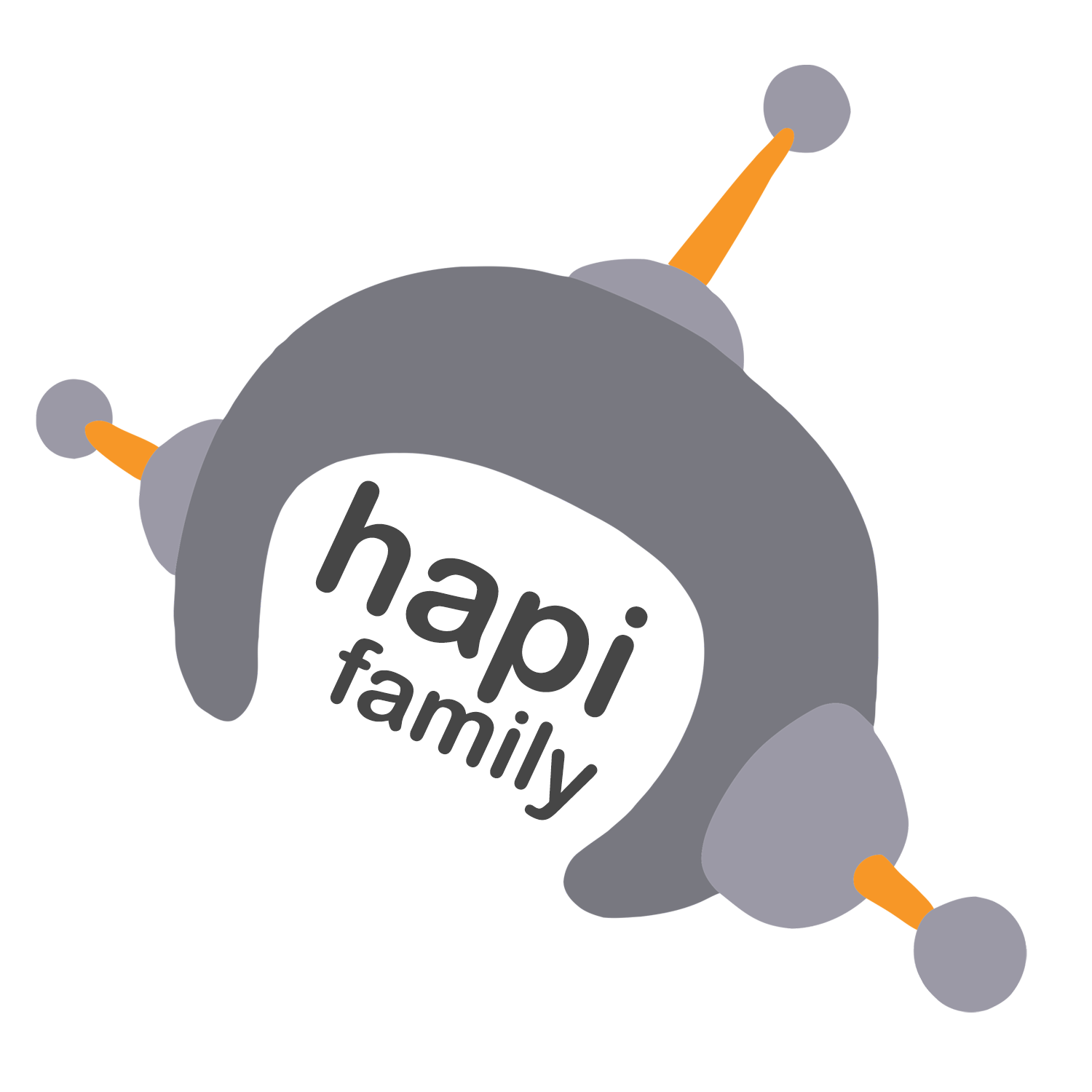
Accept
HTTP Accept-* headers parsing.

Introduction
Accept helps to answer the question of how best to respond to a HTTP request, based on the requesting browser's capabilities. Accept will parse the headers of a HTTP request and tell you what the preferred encoding is, what language should be used, and what charsets and media types are accepted.
Additional details about Accept headers and content negotiation can be found in IETF RFC 7231, Section 5.3.
API
For information on using Accept see the API documentation.