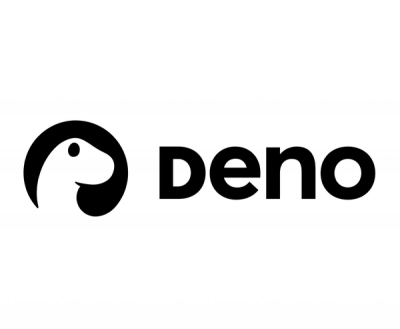
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@hapi/hapi
Advanced tools
@hapi/hapi is a rich framework for building applications and services in Node.js. It is known for its powerful plugin system, configuration-based approach, and focus on security and performance.
Routing
This code demonstrates how to set up a basic HTTP server with a single route using @hapi/hapi. The server listens on port 3000 and responds with 'Hello, world!' when the root URL is accessed.
const Hapi = require('@hapi/hapi');
const init = async () => {
const server = Hapi.server({
port: 3000,
host: 'localhost'
});
server.route({
method: 'GET',
path: '/',
handler: (request, h) => {
return 'Hello, world!';
}
});
await server.start();
console.log('Server running on %s', server.info.uri);
};
process.on('unhandledRejection', (err) => {
console.log(err);
process.exit(1);
});
init();
Plugins
This code demonstrates how to create and register a plugin in @hapi/hapi. The plugin adds a new route '/plugin' that responds with 'Hello from plugin!'.
const Hapi = require('@hapi/hapi');
const init = async () => {
const server = Hapi.server({
port: 3000,
host: 'localhost'
});
const plugin = {
name: 'myPlugin',
version: '1.0.0',
register: async function (server, options) {
server.route({
method: 'GET',
path: '/plugin',
handler: (request, h) => {
return 'Hello from plugin!';
}
});
}
};
await server.register(plugin);
await server.start();
console.log('Server running on %s', server.info.uri);
};
process.on('unhandledRejection', (err) => {
console.log(err);
process.exit(1);
});
init();
Validation
This code demonstrates how to use Joi for payload validation in @hapi/hapi. The route '/data' expects a POST request with a payload containing a 'name' and 'age' field, both of which are validated according to the specified rules.
const Hapi = require('@hapi/hapi');
const Joi = require('joi');
const init = async () => {
const server = Hapi.server({
port: 3000,
host: 'localhost'
});
server.route({
method: 'POST',
path: '/data',
options: {
validate: {
payload: Joi.object({
name: Joi.string().min(3).max(30).required(),
age: Joi.number().integer().min(0).required()
})
}
},
handler: (request, h) => {
return `Hello, ${request.payload.name}!`;
}
});
await server.start();
console.log('Server running on %s', server.info.uri);
};
process.on('unhandledRejection', (err) => {
console.log(err);
process.exit(1);
});
init();
Express is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. Compared to @hapi/hapi, Express is more lightweight and has a larger ecosystem of middleware, but it lacks the built-in configuration and plugin system that @hapi/hapi offers.
Koa is a new web framework designed by the team behind Express, aiming to be a smaller, more expressive, and more robust foundation for web applications and APIs. Koa uses async functions to eliminate callback hell and improve error handling. Compared to @hapi/hapi, Koa is more modern and minimalistic but requires more manual setup for features like routing and validation.
Fastify is a web framework highly focused on providing the best developer experience with the least overhead and a powerful plugin architecture. It is designed for high performance and low overhead. Compared to @hapi/hapi, Fastify is faster and more lightweight but has a different approach to plugins and configuration.
Build powerful, scalable applications, with minimal overhead and full out-of-the-box functionality - your code, your way.
FAQs
HTTP Server framework
The npm package @hapi/hapi receives a total of 511,548 weekly downloads. As such, @hapi/hapi popularity was classified as popular.
We found that @hapi/hapi demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.