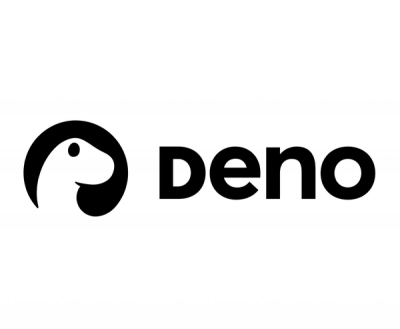
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
The @hapi/iron package is designed for encapsulated tokens (Sealed objects) creation and management. It provides a robust suite of tools for encrypting and decrypting data, creating tamper-proof, time-limited tokens that can securely transmit information between parties. This is particularly useful for creating authentication tokens, secure cookies, and other forms of secure data exchange in web applications.
Data Sealing
This feature allows you to encrypt an object, turning it into a sealed string. This string can then be safely transmitted or stored, with the assurance that it cannot be tampered with without detection.
const Iron = require('@hapi/iron');
async function sealData() {
const object = { a: 1, b: 2, c: 3 };
const password = 'some-not-very-secret-password';
const sealed = await Iron.seal(object, password, Iron.defaults);
console.log(sealed);
}
sealData();
Data Unsealing
This feature allows you to decrypt a previously sealed string, returning it to its original object form. This is crucial for accessing the data in a secure manner after it has been transmitted or stored.
const Iron = require('@hapi/iron');
async function unsealData(sealed) {
const password = 'some-not-very-secret-password';
const unsealed = await Iron.unseal(sealed, password, Iron.defaults);
console.log(unsealed);
}
// Assume 'sealed' is obtained from the sealData function
// unsealData(sealed);
jsonwebtoken (or JWT) is a package for handling JSON Web Tokens. It is similar to @hapi/iron in that it can be used for creating secure tokens for authentication and information exchange. However, JWT is specifically designed around the JSON Web Token standard, offering a different set of features focused on the structure and validation of tokens according to this standard.
node-jose is a library for JavaScript Object Signing and Encryption (JOSE). It provides functionalities for creating and handling JWTs, JWEs (JSON Web Encryption), JWSs (JSON Web Signature), and JWKs (JSON Web Key). While it overlaps with @hapi/iron in terms of creating secure tokens, node-jose offers a broader suite of tools for working with the JOSE standards, making it more versatile for applications needing to adhere to these specifications.
iron is a cryptographic utility for sealing a JSON object using symmetric key encryption with message integrity verification. Or in other words, it lets you encrypt an object, send it around (in cookies, authentication credentials, etc.), then receive it back and decrypt it. The algorithm ensures that the message was not tampered with, and also provides a simple mechanism for password rotation.
Note: the wire protocol has not changed since 1.x (the version increments reflected a change in the internal error format used by the module and by the node API as well as other node API changes).
iron provides methods for encrypting an object, generating a message authentication code (MAC), and serializing both into a cookie / URI / HTTP header friendly format. Sealed objects are useful in cases where state has to reside on other applications not under your control, without exposing the details of this state to those application.
For example, sealed objects allow you to encrypt the permissions granted to the authenticated user, store those permissions using a cookie, without worrying about someone modifying (or even knowing) what those permissions are. Any modification to the encrypted data will invalidate its integrity.
The seal process follows these general steps:
saltE
keyE
using saltE
and a passwordsaltI
keyI
using saltI
and the passwordiv
keyE
and iv
saltE
and iv
saltE
, saltI
, iv
, and the encrypted object into a URI-friendly stringTo seal an object:
const obj = {
a: 1,
b: 2,
c: [3, 4, 5],
d: {
e: 'f'
}
};
const password = 'some_not_random_password_that_is_at_least_32_characters';
try {
const sealed = await Iron.seal(obj, password, Iron.defaults);
} catch (err) {
console.log(err.message);
}
The result sealed
object is a string which can be sent via cookies, URI query parameter, or an
HTTP header attribute. To unseal the string:
try {
const unsealed = await Iron.unseal(sealed, password, Iron.defaults);
} catch (err) {
console.log(err.message);
}
See the detailed API Reference.
The greatest sources of security risks are usually found not in iron but in the policies and procedures surrounding its use. Implementers are strongly encouraged to assess how this module addresses their security requirements. This section includes an incomplete list of security considerations that must be reviewed and understood before using iron.
The iron password is only used to derive keys and is never sent or shared. However, in order to generate (and regenerate) the keys used to encrypt the object and compute the request MAC, the server must have access to the password in plaintext form. This is in contrast, for example, to modern operating systems, which store only a one-way hash of user credentials.
If an attacker were to gain access to the password - or worse, to the server's database of all such password - he or she would be able to encrypt and decrypt any sealed object. Accordingly, it is critical that servers protect these passwords from unauthorized access.
If you are looking for some prose explaining how all this works, there isn't any. iron is being developed as an open source project instead of a standard. In other words, the code is the specification. Not sure about something? Open an issue!
Yep.
Because you should know what you are doing and explicitly set it. The options matter a lot to the security properties of the implementation. While reasonable defaults are provided, you still need to explicitly state you want to use them.
Special thanks to Adam Barth for his infinite patience, and always insightful feedback and advice.
FAQs
Encapsulated tokens (encrypted and mac'ed objects)
The npm package @hapi/iron receives a total of 959,432 weekly downloads. As such, @hapi/iron popularity was classified as popular.
We found that @hapi/iron demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.