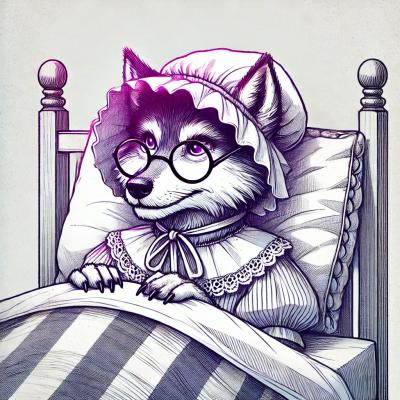
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@hapi/pez is a streaming parser for multipart payloads, which is commonly used in handling file uploads in web applications. It is part of the hapi ecosystem and provides a robust solution for parsing multipart/form-data content types.
Multipart Parser
This code demonstrates how to use @hapi/pez to parse a multipart payload. The parser is configured with a boundary string and listens for 'part' events, which represent individual parts of the multipart payload. Each part is then piped to the standard output.
const Pez = require('@hapi/pez');
const internals = {};
internals.multipart = new Pez.Multipart({
boundary: '----WebKitFormBoundaryE19zNvXGzXaLvS5C'
});
internals.multipart.on('part', (part) => {
part.pipe(process.stdout);
});
internals.multipart.write('------WebKitFormBoundaryE19zNvXGzXaLvS5C\r\n');
internals.multipart.write('Content-Disposition: form-data; name="file"; filename="example.txt"\r\n');
internals.multipart.write('Content-Type: text/plain\r\n\r\n');
internals.multipart.write('Hello World\r\n');
internals.multipart.write('------WebKitFormBoundaryE19zNvXGzXaLvS5C--\r\n');
internals.multipart.end();
File Upload Handling
This example shows how to handle file uploads using @hapi/pez. Each part of the multipart payload is written to a file using a writable stream. The filename is extracted from the part's metadata.
const Pez = require('@hapi/pez');
const fs = require('fs');
const internals = {};
internals.multipart = new Pez.Multipart({
boundary: '----WebKitFormBoundaryE19zNvXGzXaLvS5C'
});
internals.multipart.on('part', (part) => {
const fileStream = fs.createWriteStream(part.filename);
part.pipe(fileStream);
});
internals.multipart.write('------WebKitFormBoundaryE19zNvXGzXaLvS5C\r\n');
internals.multipart.write('Content-Disposition: form-data; name="file"; filename="example.txt"\r\n');
internals.multipart.write('Content-Type: text/plain\r\n\r\n');
internals.multipart.write('Hello World\r\n');
internals.multipart.write('------WebKitFormBoundaryE19zNvXGzXaLvS5C--\r\n');
internals.multipart.end();
Busboy is a fast and low-level streaming parser for HTML form data, especially file uploads. It is similar to @hapi/pez in that it handles multipart/form-data, but it is often used with the express framework and provides a more minimalistic API.
Multer is a middleware for handling multipart/form-data, which is primarily used for uploading files. It is built on top of busboy and provides a higher-level API compared to @hapi/pez, making it easier to integrate with Express applications.
Formidable is a Node.js module for parsing form data, especially file uploads. It is similar to @hapi/pez in functionality but offers a more comprehensive API for handling various types of form data, including multipart and urlencoded forms.
FAQs
Multipart parser
The npm package @hapi/pez receives a total of 0 weekly downloads. As such, @hapi/pez popularity was classified as not popular.
We found that @hapi/pez demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.