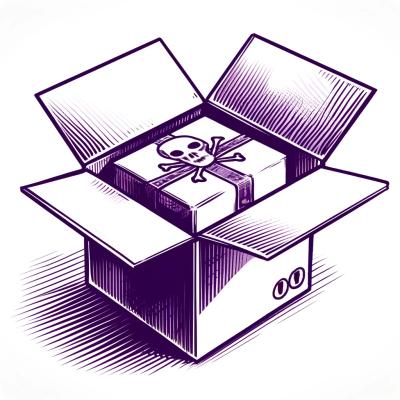
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@hapi/wreck
Advanced tools
@hapi/wreck is a powerful HTTP client library for Node.js, designed to make HTTP requests and handle responses with ease. It is part of the hapi ecosystem and provides a simple and consistent API for making HTTP requests, handling redirects, and managing cookies.
Making HTTP GET Requests
This feature allows you to make HTTP GET requests to a specified URL and handle the response. The example fetches data from a placeholder API and logs the response.
const Wreck = require('@hapi/wreck');
async function fetchData() {
const { res, payload } = await Wreck.get('https://jsonplaceholder.typicode.com/posts/1');
console.log(JSON.parse(payload.toString()));
}
fetchData();
Making HTTP POST Requests
This feature allows you to make HTTP POST requests with a payload. The example sends a JSON object to a placeholder API and logs the response.
const Wreck = require('@hapi/wreck');
async function postData() {
const payload = JSON.stringify({ title: 'foo', body: 'bar', userId: 1 });
const { res, payload: responsePayload } = await Wreck.post('https://jsonplaceholder.typicode.com/posts', { payload });
console.log(JSON.parse(responsePayload.toString()));
}
postData();
Handling Redirects
This feature allows you to handle HTTP redirects automatically. The example makes a GET request to a URL and follows up to 3 redirects.
const Wreck = require('@hapi/wreck');
async function fetchWithRedirect() {
const { res, payload } = await Wreck.get('http://example.com', { redirects: 3 });
console.log(payload.toString());
}
fetchWithRedirect();
Managing Cookies
This feature allows you to manage cookies for your HTTP requests. The example sets a cookie and makes a GET request with the cookie jar.
const Wreck = require('@hapi/wreck');
async function fetchWithCookies() {
const jar = new Wreck.CookieJar();
await jar.setCookie('session=abc123', 'http://example.com');
const { res, payload } = await Wreck.get('http://example.com', { cookies: jar });
console.log(payload.toString());
}
fetchWithCookies();
Axios is a popular promise-based HTTP client for the browser and Node.js. It provides a simple and intuitive API for making HTTP requests and handling responses. Compared to @hapi/wreck, Axios has a larger community and more extensive documentation.
Node-fetch is a lightweight module that brings the Fetch API to Node.js. It is minimalistic and focuses on providing a familiar API for making HTTP requests. Compared to @hapi/wreck, node-fetch is simpler and more lightweight, but it lacks some of the advanced features like cookie management.
Request is a comprehensive and flexible HTTP client for Node.js. It supports a wide range of features, including authentication, redirects, and cookies. However, it has been deprecated in favor of more modern alternatives like Axios and node-fetch. Compared to @hapi/wreck, Request is more feature-rich but is no longer actively maintained.
wreck is part of the hapi ecosystem and was designed to work seamlessly with the hapi web framework and its other components (but works great on its own or with other frameworks). If you are using a different web framework and find this module useful, check out hapi – they work even better together.
FAQs
HTTP Client Utilities
The npm package @hapi/wreck receives a total of 815,636 weekly downloads. As such, @hapi/wreck popularity was classified as popular.
We found that @hapi/wreck demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.