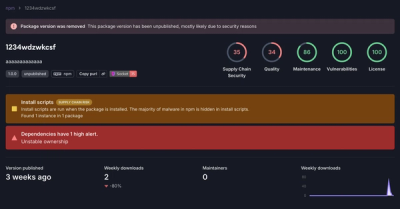
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
@headless-react/select
Advanced tools
Headless React Select component with @react-aia
and @react-stately
.
$ npm install @headless-react/select
import { Select } from '@headless-react/select'
const SelectExample = ({
label,
items
}) => {
return (
<Select items={items}>
<Select.Label>{label}</Select.Label>
<Select.PopoverTrigger>
{({ selectedItem }) => selectedItem.value.name}
</Select.PopoverTrigger>
<Select.Popover>
<Select.Options>
{({ options }) => options.map(option => (
<Select.Option key={option.key} option={option}>
{option.name}
</Select.Option>
))}
</Select.Options>
</Select.Popover>
</Select>
)
}
const MultiSelectExample = () => {
return (
<MultiSelect items={items}>
<MultiSelect.Label>{label}</Select.Label>
<MultiSelect.PopoverTrigger>
{({ selectedItems }) => selectedItems.map(item => <span>{item.value.name}</span>)}
</MultiSelect.PopoverTrigger>
<MultiSelect.Popover>
<MultiSelect.Options>
{({ options }) => options.map(option => (
<MultiSelect.Option key={option.key} option={option}>
{option.name}
</MultiSelect.Option>
))}
</MultiSelect.Options>
</MultiSelect.Popover>
</MultiSelect>
)
}
FAQs
Headless React components using react-stately and react-aria
The npm package @headless-react/select receives a total of 4 weekly downloads. As such, @headless-react/select popularity was classified as not popular.
We found that @headless-react/select demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.