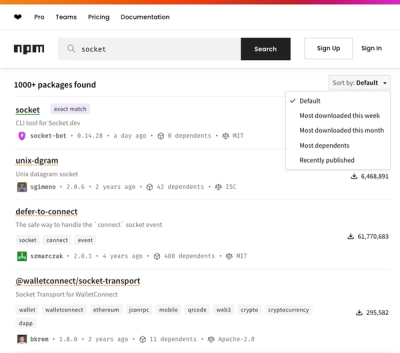
Security News
npm Updates Search Experience with New Objective Sorting Options
npm has a revamped search experience with new, more transparent sorting optionsβRelevance, Downloads, Dependents, and Publish Date.
@hedhog/auth
Advanced tools
hedhog/auth
HedHog Auth is a library designed to handle authentication tasks within the HedHog framework. It provides functionalities for user login, token management, multi-factor authentication (MFA), and password reset, ensuring secure and reliable user authentication processes.
This library is a part of the HedHog framework and is typically included as a dependency in your HedHog projects. Ensure you have the necessary dependencies and setup by following the installation guide in the HedHog framework documentation.
npm i @hedhog/auth
The AuthController
defines the following endpoints:
GET /auth/verify
: Verify the authentication status of the user.POST /auth/login
: Authenticate a user using email and password.POST /auth/otp
: Verify an OTP code for multi-factor authentication.POST /auth/forget
: Request a password reset link via email.The AuthService
provides methods for:
verifyToken(token: string)
: Verify the provided JWT token.generateRandomString(length: number)
: Generate a random string of specified length.generateRandomNumber()
: Generate a random 6-digit number.loginWithEmailAndPassword(email: string, password: string)
: Authenticate users with email and password and handle multi-factor authentication if required.getToken(user)
: Generate a JWT token for the authenticated user.forget({ email }: ForgetDTO)
: Initiate a password reset process for the specified email.otp({ token, code }: OtpDTO)
: Verify the OTP code provided by the user.auth/
βββ dist/ # Compiled JavaScript files from build
βββ node_modules/ # Discardable folder with all module dependencies
βββ src/
β βββ auth.controller.ts # Defines routes for authentication
β βββ auth.service.ts # Contains authentication logic
β βββ auth.module.ts # Authentication module
β βββ index.ts # Entry point for auth files
β βββ dto/
β β βββ forget.dto.ts # Data Transfer Object for password reset
β β βββ login.dto.ts # Data Transfer Object for login
β β βββ otp.dto.ts # Data Transfer Object for OTP verification
β βββ decorators/
β β βββ public.decorator.ts # Custom decorator to mark public routes
β β βββ user.decorator.ts # Custom decorator to get user from request
β βββ types/
β β βββ user.type.ts # Type definitions for user-related data
β βββ enums/
β β βββ multifactor-type.enum.ts # Enumeration for multi-factor authentication types
β βββ guards/
β β βββ auth.guard.ts # Guard for protecting routes
β βββ migrations/
β β βββ index.ts # Migration scripts
βββ .gitignore # Specifies which files Git should ignore
βββ package.json # Manages dependencies and scripts for the library
βββ package-lock.json # Lock file for package dependencies
βββ README.md # Documentation for the library
βββ tsconfig.lib.json # TypeScript configuration for the library
βββ tsconfig.production.json # TypeScript configuration for production builds
FAQs
Unknown package
The npm package @hedhog/auth receives a total of 0 weekly downloads. As such, @hedhog/auth popularity was classified as not popular.
We found that @hedhog/auth demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Β It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
npm has a revamped search experience with new, more transparent sorting optionsβRelevance, Downloads, Dependents, and Publish Date.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.