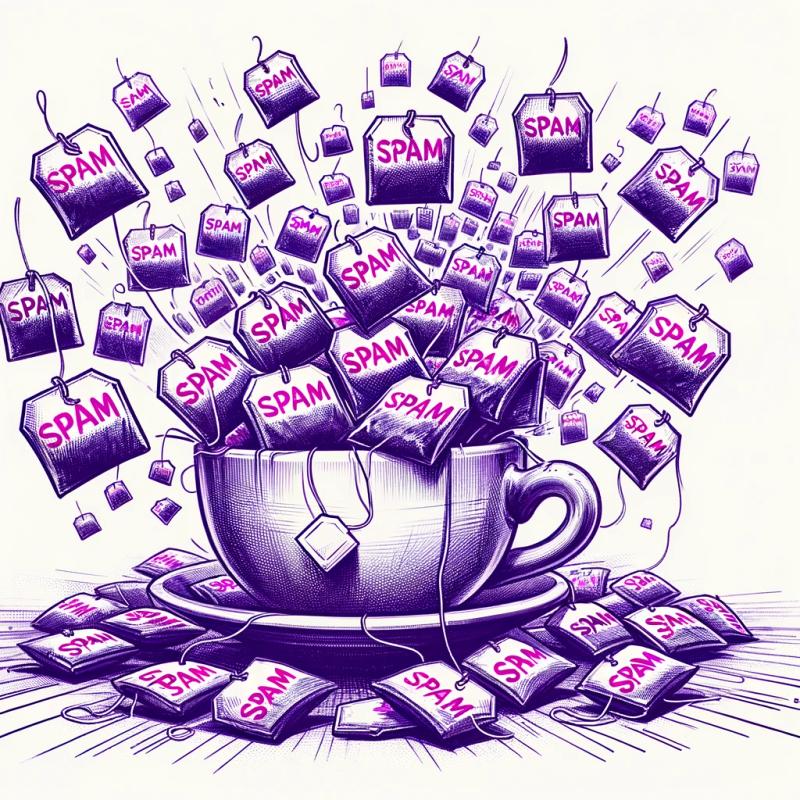
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@highlight-ui/typography
Advanced tools
This is the Typography package used by UI-components in Personio
Readme
The Typography components which manage the usage of typographic design tokens through props.
fontFamily
, fontSize
, fontWeight
, letterSpacing
, lineHeight
, textTransform
, and textDecoration
2xlarge
, xlarge
, large
, medium
, small
and xsmall
which are identical with the Figma assets.large
, base
, small
, strong-large
, strong-base
and strong-small
which are identical with the Figma assets.Using npm:
npm install @highlight-ui/typography
Using yarn:
yarn add @highlight-ui/typography
Using pnpm:
pnpm add @highlight-ui/typography
In your (S)CSS file:
@import url('@highlight-ui/typography');
Once the package is installed, you can import the library:
import { Body, Heading } from '@highlight-ui/typography';
import React from 'react';
import { Typography } from '@highlight-ui/typography';
export default function Example() {
return <Typography>Text</Typography>;
}
import React from 'react';
import { Heading } from '@highlight-ui/typography';
export default function Example() {
return (
<Heading as="h2" variant="xlarge" color="text-default">
some large heading
</Heading>
);
}
import React from 'react';
import { Body } from '@highlight-ui/typography';
export default function Example() {
return (
<Body variant="large" color="text-default">
some large text
</Body>
);
}
By default, the <Body />
component renders a span
element, whereas the <Heading />
component has no default (refer to the props table below). To change the HTML tag that will be used for rendering, the as
prop can be used to specify any other HTML tag.
import React from 'react';
import { Body } from '@highlight-ui/typography';
export default function Example() {
return (
<Body as="p" color="text-default">
Text
</Body>
);
}
By default, the Typography component applies the body-base
typography token. To override this default style, the token
prop can be used to change the applied typographic style.
import React from 'react';
import { Typography } from '@highlight-ui/typography';
export default function Example() {
return <Typography token="heading-medium">Text</Typography>;
}
Individual font properties can be overriden through props such as fontFamily
. Other properties that can be overriden are: fontSize
, fontWeight
, letterSpacing
, lineHeight
, textTransform
, and textDecoration
. For more details on these props, check out the component's props table.
import React from 'react';
import { Typography } from '@highlight-ui/typography';
export default function Example() {
return <Typography fontFamily="mono">Text</Typography>;
}
Use the className
prop to customize the component through CSS classes.
import React from 'react';
import { Typography } from '@highlight-ui/typography';
import styles from './customStyles.scss';
export default function Example() {
return <Typography className={styles.wrappedText}>Text</Typography>;
}
Prop | Type | Required | Default | Description |
---|---|---|---|---|
color | TypographyColorToken | no | Specifies a text color | |
as | h1 ,h2 , h3 , h4 , h5 , h6 , span | yes | Specifies an HTML tag for rendering the element | |
variant | 2xlarge , xlarge , large , medium , small , xsmall | yes | Specifies a variant for rendering the element | |
truncate | boolean | no | false | Whether to truncate the text |
metadata | ComponentMetadata | no | An object consisting of testId and actionName properties which will be mapped as HTML data attributes onto the root element | |
id | string | no | Specifies a unique id for the element | |
title | string | no | Specifies extra information about the element |
Prop | Type | Required | Default | Description |
---|---|---|---|---|
color | TypographyColorToken | no | Specifies a text color | |
as | p ,span | no | span | Specifies an HTML tag for rendering the element |
variant | large , base , small , strong-large , strong-base , strong-small | no | base | Specifies a variant for rendering the element |
truncate | boolean | no | false | Whether to truncate the text |
metadata | ComponentMetadata | no | An object consisting of testId and actionName properties which will be mapped as HTML data attributes onto the root element | |
id | string | no | Specifies a unique id for the element | |
title | string | no | Specifies extra information about the element |
Note: This component has been deprecated. Please migrate to the Heading and Body components.
Prop | Type | Required | Default | Description |
---|---|---|---|---|
className | string | no | undefined | Allows providing a custom class name |
component | string | no | 'p' | Specifies an HTML tag for rendering the element |
token | TypographyToken | no | 'body-base' | Specifies typography token |
color | TypographyColorToken | no | undefined | Specifies a text color |
fontFamily | TypographyFontFamilyToken | no | undefined | Specifies font family |
fontSize | TypographyFontSizeToken | no | undefined | Specifies font size |
fontWeight | TypographyFontWeightToken | no | undefined | Specifies font weight |
letterSpacing | TypographyLetterSpacingToken | no | undefined | Specifies letter spacing |
lineHeight | TypographyLineHeightToken | no | undefined | Specifies line height |
textTransform | TypographyTextCaseToken | no | undefined | Specifies text case |
textDecoration | TypographyTextDecorationToken | no | undefined | Specifies text decoration |
Also accepts props from the React.HTMLAttributes<HTMLElement> type.
Type | Values | Description |
---|---|---|
TypographyToken | Typography token names | Used for the token prop |
TypographyColorToken | "Text" and "Text On" color token names | Used for the color prop |
TypographyFontFamilyToken | 'default' | 'mono' | Used for the fontFamily prop |
TypographyFontSizeToken | 'small' | 'base' | 'large' | 'xsmall' | 'xlarge' | '2xl' | '3xl' | Used for the fontSize prop |
TypographyFontWeightToken | 'bold' | 'regular' | 'semi-bold' | Used for the fontWeight prop |
TypographyLetterSpacingToken | 'base' | Used for the letterSpacing prop |
TypographyLineHeightToken | 'base' | 'large' | 'xlarge' | Used for the lineHeight prop |
TypographyTextCaseToken | 'none' | 'uppercase' | Used for the textTransform prop |
TypographyTextDecorationToken | 'none' | 'underline' | Used for the textDecoration prop |
import React from 'react';
import { render } from '@testing-library/react';
import { Body } from '@highlight-ui/typography';
describe('TestExample', () => {
it('test description', () => {
render(<Body>Text</Body>);
// write your expect here
});
});
These components should only be used to handle the styling of the text elements themselves. The spacing, alignment and semantics of the text elements should be managed by the parent. While doing this, it is important to consider the correct semantic page regions are being used such as <aside>
, <section>
, and <nav>
.
Accessible usage of the Typography components relies on the following:
<h1>
, <h2>
) are nested and used in a logical sequence.<h1>
(as="h1"
) element per page.The Typography component is being used in all of Highlight UI to display any kind of text element.
If you're interested in contributing, please visit our contribution page.
FAQs
This is the Typography package used by UI-components in Personio
The npm package @highlight-ui/typography receives a total of 4,625 weekly downloads. As such, @highlight-ui/typography popularity was classified as popular.
We found that @highlight-ui/typography demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 10 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.