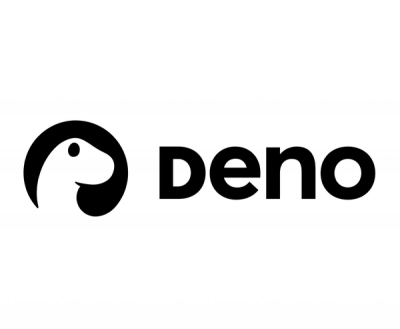
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@holaluz/npm-scripts
Advanced tools
[](https://img.shields.io/npm/v/@holaluz/npm-scripts.svg)
npm-scripts
This package contains basic npm
scripts configs for Holaluz' front-end projects.
npm install --save-dev @holaluz/npm-scripts
...and then import/extend each config file with the ones from this library:
// babel.config.js
const babelConfig = require('@holaluz/npm-scripts').babel
module.exports = babelConfig
// .eslintrc.js
const eslintConfig = require('@holaluz/npm-scripts').eslint
module.exports = eslintConfig
// prettier.config.js
const prettierConfig = require('@holaluz/npm-scripts').prettier
module.exports = prettierConfig
// stylelint.config.js
const stylelintConfig = require('@holaluz/npm-scripts').stylelint
module.exports = stylelintConfig
// postcss.config.js
const postcssConfig = require('@holaluz/npm-scripts').postcss
module.exports = postcssConfig
// lint-staged.config.js
const lintStagedConfig = require('@holaluz/npm-scripts').lintStaged
module.exports = lintStagedConfig
// simple-git-hooks.js
const simpleGitHooks = require('@holaluz/npm-scripts').simpleGitHooks
module.exports = simpleGitHooks
You can find a check-node
script which will be triggered every time this package is installed because it resides in the preinstall
npm script. Thus displaying on the terminal if the node version requirement is not meet.
# Example of the shown log when the node versions do not match
> @holaluz/npm-scripts
> node check-node.js
Version mismatch!
You must use Node version 16 or greater to run the scripts within this repo.
Version used: v14
This config should give you autoformatting on save:
{
"[javascript]": {
"editor.formatOnSave": true,
"editor.defaultFormatter": "esbenp.prettier-vscode",
},
"[css]": {
"editor.formatOnSave": true,
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[postcss]": {
"editor.formatOnSave": true,
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[vue]": {
"editor.formatOnSave": true,
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"vetur.format.defaultFormatter.html": "js-beautify-html",
"vetur.validation.template": false
}
npm-scripts
uses Semantic Release to handle the release pipeline.
Triggering a new release will create the associated Git tag, the GitHub release entry, and publish a new version on npm.
In order to trigger a new version, make sure you add the appropriate prefix and message to the squashed commit. It is based on the Angular Commit Message Conventions. In short:
# Creates a patch release (v1.0.0 -> v1.0.1)
> fix: commit message
# Creates a feature release (v1.0.0 -> v1.1.0)
> feat: commit message
# Creates a breaking release (v1.0.0 -> v2.0.0)
> fix: commit message
>
> BREAKING CHANGE: explain the breaking change # "BREAKING CHANGE:" is what triggers the breaking release
There's no need to overcomplicate things here. Keep it simple: fix
, feat
,
and chore
(plus BREAKING CHANGE
) should be enough for now.
First of all, ask yourself: Does this rule really need to be local to your project? Or should I create a PR to this repo, so that it becomes available to everyone?
If the answer is "yes, it needs to be local to my project", then you can extend any config by merging the exported object with your custom config:
const merge = require('lodash.merge')
const eslintConfig = require('@holaluz/npm-scripts').eslint
module.exports = merge(eslintConfig, {
rules: {'your-rule': 'error'}
})
FAQs
[](https://img.shields.io/npm/v/@holaluz/npm-scripts.svg)
The npm package @holaluz/npm-scripts receives a total of 8 weekly downloads. As such, @holaluz/npm-scripts popularity was classified as not popular.
We found that @holaluz/npm-scripts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.