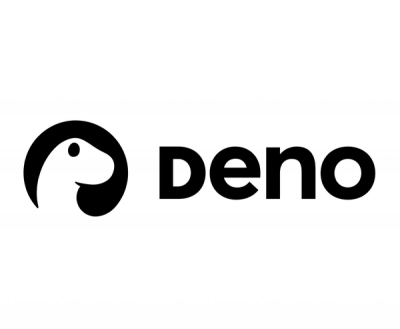
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@hoory/embed
Advanced tools
Hoory Business Embed UI
yarn add @hoory/embed
Simply put this hook in your app layout
or main
file, and it
will load the embedded chat on your website.
import { useEffect } from "react";
import { useHoory } from "@hoory/embed";
export default function App() {
const { isScriptLoaded, isChatInitialized } = useHoory("SLUG");
useEffect(() => {
if (isScriptLoaded) {
window.hoory.openChat();
}
}, [isScriptLoaded]);
return (
<div className="App">
<h1>Hoory script is {isScriptLoaded ? "isInitialized" : "not isInitialized"}</h1>
</div>
);
}
Also, you can pass additional options as second parameter to useHoory
hook and
control the widget.
type Options = {
env?: "DEV" | "PROD";
forceCacheClean?: boolean;
initializeHidden?: boolean;
forceChatInit?: boolean;
user?: {
firstName?: string;
lastName?: string;
email?: string;
avatar?: string;
};
onChatOpen?: () => void;
onChatClose?: () => void;
onShowButton?: () => void;
onHideButton?: () => void;
onChatInit?: () => void;
onScriptLoad?: () => void;
};
const api = useHoory('SLUG', options);
on the other hand, you can use the object returned from this hook in order to control the widget:
const {
isScriptLoaded,
isChatInitialized,
// Functions
showButton,
hideButton,
openChat,
closeChat,
setUserInfo,
// Listeners
onChatOpen,
onChatClose,
onShowButton,
onHideButton,
onChatInit,
onScriptLoad,
} = useHoory("WORKSPACE");
Function | Description |
---|---|
showButton | to show toggle button |
hideButton | to hide toggle button |
openChat | to open chat frame |
closeChat | to close chat frame |
setUserInfo | to set user information after an async action |
Callback Function | Description |
---|---|
onChatOpen | will be called after chat open |
onChatClose | will be called after chat close |
onShowButton | will be called after toggle button shown |
onHideButton | will be called after toggle button goes hidden |
onChatInit | will be called after chat initialized |
onScriptLoad | will be called after hoory embed scripts are loaded |
Read through our Contributing guidelines to learn about our submission process, coding rules and more
FAQs
Hoory script embedder
The npm package @hoory/embed receives a total of 27 weekly downloads. As such, @hoory/embed popularity was classified as not popular.
We found that @hoory/embed demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.