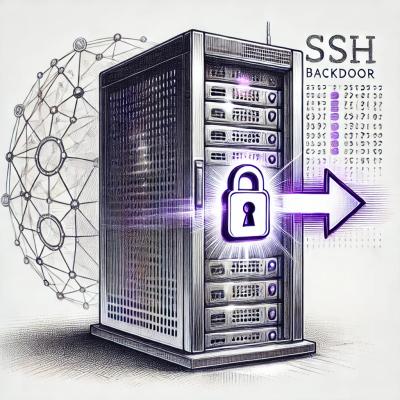
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@huddle01/observer-client-sdk
Advanced tools
This is the client SDK for the Observer service. It is a library that can be used to connect to the Observer service and execute operations on it.
npm install observer-client-sdk
import { ObserverDashboardClient } from '@huddle01/observer-client-sdk';
const dashboardClient = new ObserverClient({
// configuration options for connection
connection: {
// url of the observer service
url: 'http://localhost:8080',
// access token for the observer service
getAccessToken: async () => {
// fetch my access Token
}
},
});
dashboardClient.connect();
The ObserverDashboardClient
API provides an interface for interacting with data streams and real-time data updates via WebSocket connections. This client enables users to access call data, ongoing call information, client snapshots, and other related data through the following methods and events:
ObserverDashboardClient
.
connection
: Configuration for the WebSocket connection, using WebSocketConnectorConfig
.connect(accessToken?: string): Promise
accessToken
(optional): An access token to authenticate the connection.close(): void
findCalls(params: DashboardQueryOperations['findCalls']): Promise
params
: Object containing query filters such as producerId, consumerId, transportId, callId, roomId, clientId, and mediaTrackIdfetchOngoingCalls(): Promise
getSummaryStats(params: DashboardQueryOperations['getSummaryStats']): Promise
params
: Parameters to filter and aggregate the summary data.createDatabaseFrame(resourceId: K, conditions: DatabaseFrameConditions<DatabaseFrameSchema[K]>, updateOnCreate = true): Promise<DataFrame<DatabaseFrameSchema[K]>>
resourceId
: Identifier for the database resource.conditions
: Filter conditions for the data.updateOnCreate
(optional): Whether to fetch the initial data on creation.createCallSnapshotsFrame(callId: string): Promise<DataFrame>
callId
: Unique identifier of the call.createClientSnapshotsFrame(options: { callId: string, clientId: string }): Promise<DataFrame>
options
: An object containing:
callId
: Unique identifier of the call.clientId
: Unique identifier of the client.This API provides a structured way to access and interact with call and client data in real-time, making it suitable for applications that require live monitoring and data analysis.
The DataFrame
API provides a flexible and efficient interface for data extraction and real-time data updates. It allows users to either:
Batch Retrieval: Extract large volumes of data in chunks, such as comprehensive reports on calls or clients. This is useful for obtaining historical data or performing extensive data analysis.
Continuous Streaming: Subscribe to updates for a particular resource, receiving real-time data as it changes. This is ideal for monitoring ongoing events or tracking live updates, such as changes in call status or client activity.
constructor(
config: DataFrameConfig,
channel: WebSocketConnector,
sortFn?: (a: T, b: T) => number
)
dataFrameId
.
// Creating a new data frame instance
const myDataFrame = observerClient.createClientSnapshotsFrame({
callId: '12345',
clientId: '67890',
});
// Listen for new rows
myDataFrame.on('newrow', (row) => {
console.log('New data row received:', row);
});
// Listen for errors
myDataFrame.on('error', (error) => {
console.error('Data frame error:', error);
});
// Update the data frame
await myDataFrame.update();
// Iterate over the rows
for (const row of myDataFrame) {
console.log('Row:', row);
}
// Close the data frame when done
myDataFrame.close();
The ObserverAdminClient
is an extension of the ObserverClient
class designed to provide administrative control over the system. It offers additional methods to manage and monitor server and worker states, initiate and manage jobs, and clean up completed or failed tasks.
constructor(config: ObserverClientConfig)
ObserverClientConfig
.ConsumableJobMap
.jobId
of the started job.FAQs
ObserverClient SDK
We found that @huddle01/observer-client-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.