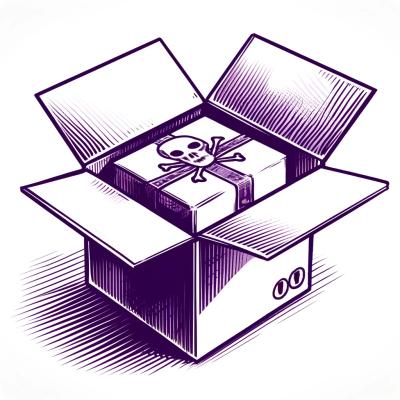
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@humanwhocodes/retry
Advanced tools
@humanwhocodes/retry is an npm package designed to simplify the process of retrying asynchronous operations. It provides a flexible and configurable way to handle retries, making it easier to manage operations that may fail intermittently, such as network requests or database queries.
Basic Retry
This feature allows you to retry a function a specified number of times if it fails. In this example, the `fetchData` function is retried up to 5 times until it succeeds.
const { retry } = require('@humanwhocodes/retry');
async function fetchData() {
// Simulate a function that may fail
if (Math.random() < 0.7) {
throw new Error('Fetch failed');
}
return 'Data fetched successfully';
}
retry(fetchData, { retries: 5 })
.then(result => console.log(result))
.catch(error => console.error(error));
Exponential Backoff
This feature allows you to implement exponential backoff, where the wait time between retries increases exponentially. In this example, the `fetchData` function is retried up to 5 times with an increasing delay between each retry.
const { retry } = require('@humanwhocodes/retry');
async function fetchData() {
if (Math.random() < 0.7) {
throw new Error('Fetch failed');
}
return 'Data fetched successfully';
}
retry(fetchData, { retries: 5, factor: 2, minTimeout: 1000 })
.then(result => console.log(result))
.catch(error => console.error(error));
Custom Retry Logic
This feature allows you to define custom retry logic by providing a `shouldRetry` function. In this example, the `shouldRetry` function logs each failed attempt and always returns true to continue retrying.
const { retry } = require('@humanwhocodes/retry');
async function fetchData() {
if (Math.random() < 0.7) {
throw new Error('Fetch failed');
}
return 'Data fetched successfully';
}
retry(fetchData, {
retries: 5,
shouldRetry: (error, attempt) => {
console.log(`Attempt ${attempt} failed: ${error.message}`);
return true;
}
})
.then(result => console.log(result))
.catch(error => console.error(error));
The `retry` package provides a similar functionality for retrying operations with customizable options. It supports exponential backoff and custom retry strategies. Compared to @humanwhocodes/retry, it offers a more extensive set of configuration options and is widely used in the community.
The `promise-retry` package is designed for retrying promise-based operations. It supports custom retry strategies and exponential backoff. It is similar to @humanwhocodes/retry but focuses specifically on promise-based workflows, making it a good choice for modern JavaScript applications.
The `async-retry` package provides a simple and flexible way to retry asynchronous operations. It supports custom retry strategies, exponential backoff, and is compatible with both promises and async/await. It is similar to @humanwhocodes/retry but offers a more concise API and is widely adopted in the community.
If you find this useful, please consider supporting my work with a donation or nominate me for a GitHub Star.
A utility for retrying failed async JavaScript calls based on the error returned.
npm install @humanwhocodes/retry
# or
yarn add @humanwhocodes/retry
Import into your Node.js project:
// CommonJS
const { Retrier } = require("@humanwhocodes/retry");
// ESM
import { Retrier } from "@humanwhocodes/retry";
Install using JSR:
deno add @humanwhocodes/retry
#or
jsr add @humanwhocodes/retry
Then import into your Deno project:
import { Retrier } from "@humanwhocodes/retry";
Install using this command:
bun add @humanwhocodes/retry
Import into your Bun project:
import { Retrier } from "@humanwhocodes/retry";
It's recommended to import the minified version to save bandwidth:
import { Retrier } from "https://cdn.skypack.dev/@humanwhocodes/retry?min";
However, you can also import the unminified version for debugging purposes:
import { Retrier } from "https://cdn.skypack.dev/@humanwhocodes/retry";
After importing, create a new instance of Retrier
and specify the function to run on the error. This function should return true
if you want the call retried and false
if not.
// this instance will retry if the specific error code is found
const retrier = new Retrier(error => {
return error.code === "ENFILE" || error.code === "EMFILE";
});
Then, call the retry()
method around the function you'd like to retry, such as:
import fs from "fs/promises";
const retrier = new Retrier(error => {
return error.code === "ENFILE" || error.code === "EMFILE";
});
const text = await retrier.retry(() => fs.readFile("README.md", "utf8"));
The retry()
method will either pass through the result on success or wait and retry on failure. Any error that isn't caught by the retrier is automatically rejected so the end result is a transparent passing through of both success and failure.
You can control how long a task will attempt to retry before giving up by passing the timeout
option to the Retrier
constructor. By default, the timeout is one minute.
import fs from "fs/promises";
const retrier = new Retrier(error => {
return error.code === "ENFILE" || error.code === "EMFILE";
}, { timeout: 100_000 });
const text = await retrier.retry(() => fs.readFile("README.md", "utf8"));
When a call times out, it rejects the first error that was received from calling the function.
When processing a large number of function calls, you can limit the number of concurrent function calls by passing the concurrency
option to the Retrier
constructor. By default, concurrency
is 1000.
import fs from "fs/promises";
const retrier = new Retrier(error => {
return error.code === "ENFILE" || error.code === "EMFILE";
}, { concurrency: 100 });
const filenames = getFilenames();
const contents = await Promise.all(
filenames.map(filename => retrier.retry(() => fs.readFile(filename, "utf8"))
);
AbortSignal
You can also pass an AbortSignal
to cancel a retry:
import fs from "fs/promises";
const controller = new AbortController();
const retrier = new Retrier(error => {
return error.code === "ENFILE" || error.code === "EMFILE";
});
const text = await retrier.retry(
() => fs.readFile("README.md", "utf8"),
{ signal: controller.signal }
);
npm install
to setup dependenciesnpm test
to run testsEnable debugging output by setting the DEBUG
environment variable to "@hwc/retry"
before running.
Apache 2.0
This utility is inspired by, and contains code from graceful-fs
.
FAQs
A utility to retry failed async methods.
We found that @humanwhocodes/retry demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.