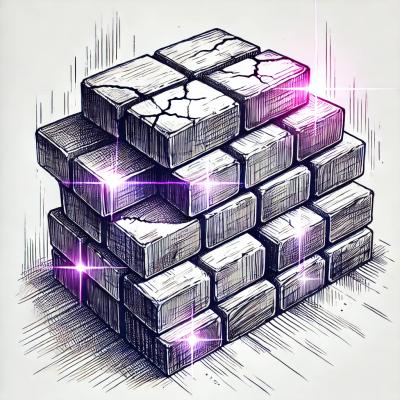
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@iherman/minicrypto
Advanced tools
Set of functions that can be used to perform basic cryptographic functions hiding all the intricacies of WebCrypto.
NOT FINAL, DO NOT USE IT FOR CRITICAL APPLICATIONS
This package contains a set of functions that can be used to perform some basic cryptographic functions on top of the WebCrypto API standard, without getting into all the intricacies required by that API (or other, similar packages). Obviously, some of the more complex options are not available; goal is to provide an interface to the simplest usages.
The interface functions are as follows.
The functions can be used to generate a private/public (also referred to as secret/public) keys. The package gives an option among four different asymmetric cryptographic key types:
A key pair is either stored as
The coding examples (see the API documentation for details):
// Simple key generation
import { generateKeysJWK, generateKeysMK } from "@iherman/minicrypto";
const keyPairEdDSACrypto = await generateKeys("eddsa");
// Alternatively generate keys in JWK and Multikey formats.
const keyPairEdDSAJWK = await generateKeysJWK("eddsa");
const keyPairECDSAMK = await generateKeysMK("ecdsa");
// ECDSA Keys using P-384
const keyPairECDSAMK384 = await generateKeysMK("ecdsa", {namedCurve: "P-384"});
// RSA Keys generated with a larger modulus (larger key, more secure) for signature/verification
const keyPairRSAPSS = await generateKeysJWK("rsa-pss", {modulusLength: 4096}); // Default is 2048
// RSA Keys for encryption/decryption, using the default modulus length
const keyPairRSAOAEP = await generateKeys("rsa-oaep");
The keys can be used to sign a string message and to verify the signature. The signature itself is encoded as base64 (more precisely, base-64-url-no-pad) or base58 encoding (more precisely, base-58-btc) and, optionally, stored as a Multibase string. By default, if the key is in JWK or using the binary format, the signature is generated as a plain, base64 string. If the key is Multikey/Multibase, then by default the signature is stored as a Multibase, base58 string. Other combinations must be set explicitly.
Note that the sign
and verify
functions accept the keys in any formats, i.e., as pairs or as individual keys
(private or secret, depending on the function). The only exception is that the sign
function requires a full
Multikey, the (Multibase encoded) secret key by itself is not accepted.
The coding examples (see the API for details):
import { sign, verify } from "@iherman/minicrypto";
const message = "This is the string to be signed";
// Signature stored as plain base64
const signature64: string = await sign(message, keyPairRSAPSS);
const isValid64: boolean = await verify(message, signature, keyPairRSAPSS.publicKeyJwk);
// Signature stored as multibase base58
const signature58: string = await sign(message, keyPairECDSAMK);
const isValid58: boolean = await verify(message, signature, keyPairECDSAMK.publicKeyMultibase);
By default, if the key is in JWK, the ciphertext is generated as a plain, base64 string. Other combinations must be set explicitly.
import { encrypt, decrypt } from "@iherman/minicrypto";
const message2 = "This is the string to be encrypted";
// Signature stored as plain base64
const ciphertext64: string = await encrypt(message2, keyPairRSAOAEP.publicKey);
// Signature stored as multibase base58
const ciphertext58: string = await encrypt(message2, keyPairRSAOAEP.publicKey, {encoding: "base58", format: "multibase"});
// Decrypt a ciphertext stored in multibase. Note that the encoding field is not required, it is automatically recognized
const message2_decrypted: string = await decrypt(ciphertext58, keyPairRSAOAEP.privateKey, {format: "multibase"});
Very frequently used for various type of data. There are many hash functions around, the most widely used these days is "SHA-256", with "SHA-384" as a more secure alternative.
import { hash } from "@iherman/minicrypto";
const message = "This is the string to be hashed";
const hash_256 = await hash(message)
const hash_384 = await hash(message, "SHA-384"); // Default is SHA-256
The package contains some functions to convert JWK keys or key pairs to and from their binary equivalents. For the sake of convenience, ths package also re-exports similar functions for Multikeys and Multibase, though it is implemented by a different, underlying package called multikey-webcrypto.
FAQs
Set of functions that can be used to perform basic cryptographic functions hiding all the intricacies of WebCrypto.
The npm package @iherman/minicrypto receives a total of 114 weekly downloads. As such, @iherman/minicrypto popularity was classified as not popular.
We found that @iherman/minicrypto demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.