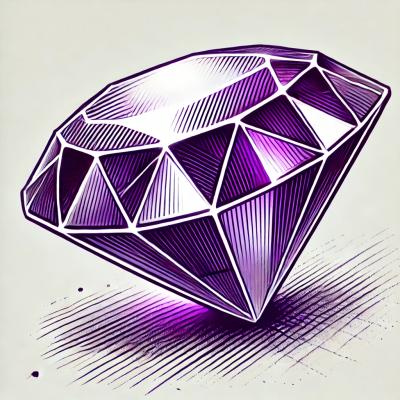
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@improbable-eng/grpc-web
Advanced tools
@improbable-eng/grpc-web is a JavaScript library that allows you to use gRPC in web applications. It provides a way to communicate with gRPC services from the browser using HTTP/1.1 or HTTP/2, enabling real-time communication and efficient data transfer.
Unary RPC
This feature allows you to make a unary RPC call to a gRPC service. The code sample demonstrates how to create a request, set its parameters, and send it to the server. The response is handled in the onEnd callback.
const {grpc} = require('@improbable-eng/grpc-web');
const {MyService} = require('./my_service_pb_service');
const {MyRequest} = require('./my_service_pb');
const request = new MyRequest();
request.setMessage('Hello, World!');
grpc.unary(MyService.MyMethod, {
request: request,
host: 'http://localhost:8080',
onEnd: (response) => {
const {status, message} = response;
if (status === grpc.Code.OK && message) {
console.log('Response:', message.toObject());
}
}
});
Server Streaming RPC
This feature allows you to make a server streaming RPC call to a gRPC service. The code sample demonstrates how to create a request, set its parameters, and handle incoming messages from the server in real-time.
const {grpc} = require('@improbable-eng/grpc-web');
const {MyService} = require('./my_service_pb_service');
const {MyRequest} = require('./my_service_pb');
const request = new MyRequest();
request.setMessage('Hello, World!');
const stream = grpc.invoke(MyService.MyStreamingMethod, {
request: request,
host: 'http://localhost:8080',
onMessage: (message) => {
console.log('Received message:', message.toObject());
},
onEnd: (status, statusMessage, trailers) => {
if (status === grpc.Code.OK) {
console.log('Stream ended successfully');
} else {
console.error('Stream ended with error:', statusMessage);
}
}
});
Client Streaming RPC
This feature allows you to make a client streaming RPC call to a gRPC service. The code sample demonstrates how to create a stream, send multiple requests, and handle the end of the stream.
const {grpc} = require('@improbable-eng/grpc-web');
const {MyService} = require('./my_service_pb_service');
const {MyRequest} = require('./my_service_pb');
const stream = grpc.client(MyService.MyClientStreamingMethod, {
host: 'http://localhost:8080'
});
stream.onEnd((status, statusMessage, trailers) => {
if (status === grpc.Code.OK) {
console.log('Stream ended successfully');
} else {
console.error('Stream ended with error:', statusMessage);
}
});
const request1 = new MyRequest();
request1.setMessage('Message 1');
stream.send(request1);
const request2 = new MyRequest();
request2.setMessage('Message 2');
stream.send(request2);
stream.finishSend();
Bidirectional Streaming RPC
This feature allows you to make a bidirectional streaming RPC call to a gRPC service. The code sample demonstrates how to create a stream, send multiple requests, and handle incoming messages from the server in real-time.
const {grpc} = require('@improbable-eng/grpc-web');
const {MyService} = require('./my_service_pb_service');
const {MyRequest} = require('./my_service_pb');
const stream = grpc.client(MyService.MyBidirectionalStreamingMethod, {
host: 'http://localhost:8080'
});
stream.onMessage((message) => {
console.log('Received message:', message.toObject());
});
stream.onEnd((status, statusMessage, trailers) => {
if (status === grpc.Code.OK) {
console.log('Stream ended successfully');
} else {
console.error('Stream ended with error:', statusMessage);
}
});
const request1 = new MyRequest();
request1.setMessage('Message 1');
stream.send(request1);
const request2 = new MyRequest();
request2.setMessage('Message 2');
stream.send(request2);
stream.finishSend();
grpc-web is an official library from the gRPC team that provides similar functionality to @improbable-eng/grpc-web. It allows you to use gRPC in web applications by providing a JavaScript client library. Compared to @improbable-eng/grpc-web, grpc-web is more tightly integrated with the official gRPC ecosystem and may have better support and documentation.
grpc is the official Node.js library for gRPC. While it is primarily designed for server-side use, it can also be used in client-side applications with some additional setup. Compared to @improbable-eng/grpc-web, grpc is more versatile and can be used in a wider range of environments, but it may require more configuration to work in web applications.
Library for making gRPC-Web requests from a browser
This library is intended for both JavaScript and TypeScript usage from a web browser or NodeJS (see Usage with NodeJS).
Note: This only works if the server supports gRPC-Web
A Golang gRPC-Web middleware and a Golang-based gRPC-Web proxy are available here.
Please see the full gRPC-Web README for known limitations.
@improbable-eng/grpc-web
has peer dependencies of google-protobuf
and @types/google-protobuf
.
npm install google-protobuf @types/google-protobuf @improbable-eng/grpc-web --save
There is an example project available here
ts-protoc-gen
with protoc
to generate .js
and .d.ts
files for your request and response classes. ts-protoc-gen
can also generate gRPC service definitions with the service=true
argument.
unary()
, invoke()
or client()
import {grpc} from "@improbable-eng/grpc-web";
// Import code-generated data structures.
import {BookService} from "./generated/proto/examplecom/library/book_service_pb_service";
import {GetBookRequest} from "./generated/proto/examplecom/library/book_service_pb";
const getBookRequest = new GetBookRequest();
getBookRequest.setIsbn(60929871);
grpc.unary(BookService.GetBook, {
request: getBookRequest,
host: host,
onEnd: res => {
const { status, statusMessage, headers, message, trailers } = res;
if (status === grpc.Code.OK && message) {
console.log("all ok. got book: ", message.toObject());
}
}
});
const request = grpc.unary(BookService.GetBook, { ... });
request.cancel();
There are three functions for making gRPC requests:
grpc.unary
This is a convenience function for making requests that consist of a single request message and single response message. It can only be used with unary methods.
rpc GetBook(GetBookRequest) returns (Book) {}
grpc.invoke
This is a convenience function for making requests that consist of a single request message and a stream of response messages (server-streaming). It can also be used with unary methods.
rpc GetBook(GetBookRequest) returns (Book) {}
rpc QueryBooks(QueryBooksRequest) returns (stream Book) {}
grpc.client
grpc.client
returns a client. Dependant upon transport compatibility this client is capable of sending multiple request messages (client-streaming) and receiving multiple response messages (server-streaming). It can be used with any type of method, but will enforce limiting the sending of messages for unary methods.
rpc GetBook(GetBookRequest) returns (Book) {}
rpc QueryBooks(QueryBooksRequest) returns (stream Book) {}
rpc LogReadPages(stream PageRead) returns (google.protobuf.Empty) {}
rpc ListenForBooks(stream QueryBooksRequest) returns (stream Book) {}
Refer to grpc-web-node-http-transport.
FAQs
gRPC-Web client for browsers (JS/TS)
The npm package @improbable-eng/grpc-web receives a total of 69,684 weekly downloads. As such, @improbable-eng/grpc-web popularity was classified as popular.
We found that @improbable-eng/grpc-web demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.