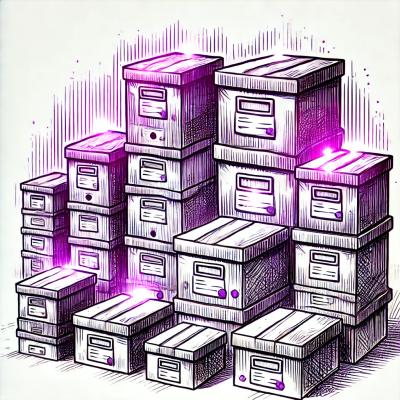
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
@inscreen/html-to-image
Advanced tools
Generates an image from a DOM node using HTML5 canvas and SVG.
✂️ Generates an image from a DOM node using HTML5 canvas and SVG.
Fork from dom-to-image with more maintainable code and some new features.
npm install --save html-to-image
/* ES6 */
import * as htmlToImage from 'html-to-image';
import { toPng, toJpeg, toBlob, toPixelData, toSvg } from 'html-to-image';
/* ES5 */
var htmlToImage = require('html-to-image');
All the top level functions accept DOM node and rendering options, and return a promise fulfilled with corresponding dataURL:
Go with the following examples.
Get a PNG image base64-encoded data URL and display it right away:
var node = document.getElementById('my-node');
htmlToImage.toPng(node)
.then(function (dataUrl) {
var img = new Image();
img.src = dataUrl;
document.body.appendChild(img);
})
.catch(function (error) {
console.error('oops, something went wrong!', error);
});
Get a PNG image base64-encoded data URL and download it (using download):
htmlToImage.toPng(document.getElementById('my-node'))
.then(function (dataUrl) {
download(dataUrl, 'my-node.png');
});
Get an SVG data URL, but filter out all the <i>
elements:
function filter (node) {
return (node.tagName !== 'i');
}
htmlToImage.toSvg(document.getElementById('my-node'), { filter: filter })
.then(function (dataUrl) {
/* do something */
});
Save and download a compressed JPEG image:
htmlToImage.toJpeg(document.getElementById('my-node'), { quality: 0.95 })
.then(function (dataUrl) {
var link = document.createElement('a');
link.download = 'my-image-name.jpeg';
link.href = dataUrl;
link.click();
});
Get a PNG image blob and download it (using FileSaver):
htmlToImage.toBlob(document.getElementById('my-node'))
.then(function (blob) {
if (window.saveAs) {
window.saveAs(blob, 'my-node.png');
} else {
FileSaver.saveAs(blob, 'my-node.png');
}
});
Get a HTMLCanvasElement, and display it right away:
htmlToImage.toCanvas(document.getElementById('my-node'))
.then(function (canvas) {
document.body.appendChild(canvas);
});
Get the raw pixel data as a Uint8Array with every 4 array elements representing the RGBA data of a pixel:
var node = document.getElementById('my-node');
htmlToImage.toPixelData(node)
.then(function (pixels) {
for (var y = 0; y < node.scrollHeight; ++y) {
for (var x = 0; x < node.scrollWidth; ++x) {
pixelAtXYOffset = (4 * y * node.scrollHeight) + (4 * x);
/* pixelAtXY is a Uint8Array[4] containing RGBA values of the pixel at (x, y) in the range 0..255 */
pixelAtXY = pixels.slice(pixelAtXYOffset, pixelAtXYOffset + 4);
}
}
});
import React, { useCallback, useRef } from 'react';
import { toPng } from 'html-to-image';
const App: React.FC = () => {
const ref = useRef<HTMLDivElement>(null)
const onButtonClick = useCallback(() => {
if (ref.current === null) {
return
}
toPng(ref.current, { cacheBust: true, })
.then((dataUrl) => {
const link = document.createElement('a')
link.download = 'my-image-name.png'
link.href = dataUrl
link.click()
})
.catch((err) => {
console.log(err)
})
}, [ref])
return (
<>
<div ref={ref}>
{/* DOM nodes you want to convert to PNG */}
</div>
<button onClick={onButtonClick}>Click me</button>
</>
)
}
(domNode: HTMLElement) => boolean
A function taking DOM node as argument. Should return true if passed node should be included in the output. Excluding node means excluding it's children as well.
You can add filter to every image function. For example,
const filter = (node: HTMLElement) => {
const exclusionClasses = ['remove-me', 'secret-div'];
return !exclusionClasses.some((classname) => node.classList?.contains(classname));
}
htmlToImage.toJpeg(node, { quality: 0.95, filter: filter});
or
htmlToImage.toPng(node, {filter:filter})
Not called on the root node.
A string value for the background color, any valid CSS color value.
Width and height in pixels to be applied to node before rendering.
Allows to scale the canva's size including the elements inside to a given width and height (in pixels).
An object whose properties to be copied to node's style before rendering. You might want to check this reference for JavaScript names of CSS properties.
A number between 0
and 1
indicating image quality (e.g. 0.92
=> 92%
) of the JPEG image.
Defaults to 1.0
(100%
)
Set to true to append the current time as a query string to URL requests to enable cache busting.
Defaults to false
Set false to use all URL as cache key. If the value has falsy value, it will exclude query params from the provided URL.
Defaults to false
A data URL for a placeholder image that will be used when fetching an image fails.
Defaults to an empty string and will render empty areas for failed images.
The pixel ratio of the captured image. Default use the actual pixel ratio of the device. Set 1
to
use as initial-scale 1
for the image.
The format required for font embedding. This is a useful optimisation when a webfont provider specifies several different formats for fonts in the CSS, for example:
@font-face {
name: 'proxima-nova';
src: url("...") format("woff2"), url("...") format("woff"), url("...") format("opentype");
}
Instead of embedding each format, all formats other than the one specified will be discarded. If this option is not specified then all formats will be downloaded and embedded.
When supplied, the library will skip the process of parsing and embedding webfont URLs in CSS,
instead using this value. This is useful when combined with getFontEmbedCSS()
to only perform the
embedding process a single time across multiple calls to library functions.
const fontEmbedCss = await htmlToImage.getFontEmbedCSS(element1);
html2Image.toSVG(element1, { fontEmbedCss });
html2Image.toSVG(element2, { fontEmbedCss });
When supplied, the library will skip the process of scaling extra large doms into the canvas object.
You may experience loss of parts of the image if set to true
and you are exporting a very large image.
Defaults to false
A string indicating the image format. The default type is image/png; that type is also used if the given type isn't supported. When supplied, the toCanvas function will return a blob matching the given image type and quality.
Defaults to image/png
Only standard lib is currently used, but make sure your browser supports:
<foreignObject>
tagIt's tested on latest Chrome, Firefox and Safari (49, 45 and 16 respectively at the time of writing), with Chrome performing significantly better on big DOM trees, possibly due to it's more performant SVG support, and the fact that it supports CSSStyleDeclaration.cssText
property.
Internet Explorer is not (and will not be) supported, as it does not support SVG <foreignObject>
tag.
There might some day exist (or maybe already exists?) a simple and standard way of exporting parts of the HTML to image (and then this script can only serve as an evidence of all the hoops I had to jump through in order to get such obvious thing done) but I haven't found one so far.
This library uses a feature of SVG that allows having arbitrary HTML content inside of the <foreignObject>
tag. So, in order to render that DOM node for you, following steps are taken:
@font-face
declarations that might represent web fonts<style>
element, then attach it to the clone<img>
elementsbackground
CSS property, in a fashion similar to fonts<foreignObject>
tag, then into the SVG, then make it a data URL<canvas>
element with something drawn on it, it should be handled fine, unless the canvas is tainted - in this case rendering will rather not succeed.Please let us know how can we help. Do check out issues for bug reports or suggestions first.
To become a contributor, please follow our contributing guide.
The scripts and documentation in this project are released under the MIT License
FAQs
Generates an image from a DOM node using HTML5 canvas and SVG.
The npm package @inscreen/html-to-image receives a total of 3 weekly downloads. As such, @inscreen/html-to-image popularity was classified as not popular.
We found that @inscreen/html-to-image demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.