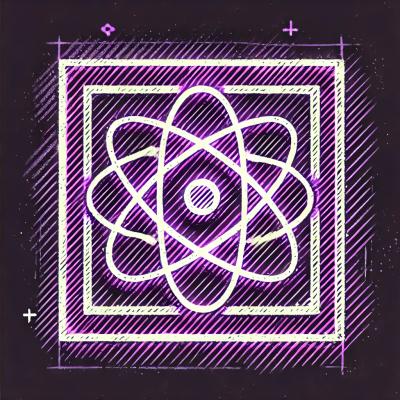
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
@interactivefitness/paclient
Advanced tools
A PulseAudio client written in pure JavaScript for node.js.
Currently most commands are implemented. SHM/MemFD-related commands are not supported.
npm install paclient
const PAClient = require('paclient');
const pa = new PAClient();
pa.on('ready', () => {
console.log('Ready');
pa.subscribe('all');
}).on('close', () => {
console.log('Connection closed');
}).on('new', (type, index) => {
pa[getFnFromType(type)](index, (err, info) => {
if (err) {
console.log(`Could not fetch ${type}, index ${index}: ${err.message}`);
return;
}
var name = info.name || info.description || '<unknown>';
console.log(`"${name}" (${type}) added`);
});
}).on('change', (type, index) => {
pa[getFnFromType(type)](index, (err, info) => {
if (err) {
console.log(`Could not fetch ${type}, index ${index}: ${err.message}`);
return;
}
var name = info.name || info.description || '<unknown>';
console.log(`"${name}" (${type}) changed`);
});
}).on('remove', (type, index) => {
console.log(`Removed ${type}, index #${index}`);
});
pa.connect();
function getFnFromType(type) {
var fn;
switch (type) {
case 'sink':
case 'card':
case 'source': fn = type; break;
case 'sinkInput':
case 'sourceOutput':
case 'client':
case 'module': fn = `${type}ByIndex`; break;
default:
throw new Error('Unexpected type: ' + type);
}
return 'get' + fn[0].toUpperCase() + fn.slice(1);
}
ready() - A successful, authenticated connection has been made.
close() - The connection to the server has been closed.
new(< string >type, < integer >index) - A new entity has been added.
change(< string >type, < integer >index) - An existing entity has changed.
remove(< string >type, < integer >index) - An existing entity has been removed.
(constructor)() - Creates and returns a new Client instance.
connect([< object >config]) - (void) - Attempts a connection to a server using the information given in config
. If no path
or host
are supplied then connection details are autodetected using the same algorithm as the official PulseAudio client library. Valid config
options are:
path - string - Path to a UNIX socket of the server. Default: Autodetected
host - string - Hostname or IP address of the server. Default: Autodetected
port - integer - Port number of the server. Default: 4713
serverString - string - Server string in PULSE_SERVER syntax. Default: Autodetected
cookie - mixed - An explicit cookie value to use when authenticating with the server. This can either be a Buffer or a hex string of the appropriate length. Default: Autodetected
properties - object - A set of properties to associate with the client on the server. These can be seen by other clients. Default: { application: { name: 'paclient.js' } }
end() - (void) - If connected, this will close the connection to the server.
getModules(< function >callback) - (void) - Retrieves a list of loaded modules. callback
has 2 parameters: < Error >err, < array >modules.
getClients(< function >callback) - (void) - Retrieves a list of connected clients. callback
has 2 parameters: < Error >err, < array >clients.
getSinks(< function >callback) - (void) - Retrieves a list of available sinks (outputs). callback
has 2 parameters: < Error >err, < array >sinks.
getSources(< function >callback) - (void) - Retrieves a list of available sources (inputs). callback
has 2 parameters: < Error >err, < array >sources.
getSinkInputs(< function >callback) - (void) - Retrieves a list of available sink inputs (streams connected to outputs). callback
has 2 parameters: < Error >err, < array >sinkInputs.
getSourceOutputs(< function >callback) - (void) - Retrieves a list of available source outputs (streams connected to inputs). callback
has 2 parameters: < Error >err, < array >sourceOutputs.
getCards(< function >callback) - (void) - Retrieves a list of available cards (hardware devices that usually combine a single source (for recording) and a single sink (for playback)). callback
has 2 parameters: < Error >err, < array >cards.
getServerInfo(< function >callback) - (void) - Retrieves information about the server. callback
has 2 parameters: < Error >err, < object >info.
getModuleByIndex(< integer >index, < function >callback) - (void) - Retrieves a module by its index. callback
has 2 parameters: < Error >err, < object >module.
getClientByIndex(< integer >index, < function >callback) - (void) - Retrieves a client by its index. callback
has 2 parameters: < Error >err, < object >client.
getSink(< mixed >criteria, < function >callback) - (void) - Retrieves a sink by either its index (integer) or its name (string). callback
has 2 parameters: < Error >err, < object >sink.
getSource(< mixed >criteria, < function >callback) - (void) - Retrieves a source by either its index (integer) or its name (string). callback
has 2 parameters: < Error >err, < object >source.
getSinkInputByIndex(< integer >index, < function >callback) - (void) - Retrieves a sink input by its index. callback
has 2 parameters: < Error >err, < object >sinkInput.
getSourceOutputByIndex(< integer >index, < function >callback) - (void) - Retrieves a source output by its index. callback
has 2 parameters: < Error >err, < object >sourceOutput.
getCard(< mixed >criteria, < function >callback) - (void) - Retrieves a card by either its index (integer) or its name (string). callback
has 2 parameters: < Error >err, < object >card.
getSinkIndexByName(< string >name, < function >callback) - (void) - Retrieves the index for a sink given its name
. callback
has 2 parameters: < Error >err, < integer >index.
getSourceIndexByName(< string >name, < function >callback) - (void) - Retrieves the index for a source given its name
. callback
has 2 parameters: < Error >err, < integer >index.
setSinkVolumes(< mixed >criteria, < array >volumes, < function >callback) - (void) - Sets the volumes for each of a sink's channels. criteria
can be an index (integer) or a name (string). callback
has 1 parameter: < Error >err.
setSourceVolumes(< mixed >criteria, < array >volumes, < function >callback) - (void) - Sets the volumes for each of a source's channels. criteria
can be an index (integer) or a name (string). callback
has 1 parameter: < Error >err.
setSinkInputVolumesByIndex(< integer >index, < array >volumes, < function >callback) - (void) - Sets the volumes for each of a sink input's channels. callback
has 1 parameter: < Error >err.
setSourceOutputVolumesByIndex(< integer >index, < array >volumes, < function >callback) - (void) - Sets the volumes for each of a source output's channels. callback
has 1 parameter: < Error >err.
setSinkMute(< mixed >criteria, < boolean >muted, < function >callback) - (void) - Sets the muted status for a sink by either its index (integer) or its name (string). callback
has 1 parameter: < Error >err.
setSourceMute(< mixed >criteria, < boolean >muted, < function >callback) - (void) - Sets the muted status for a source by either its index (integer) or its name (string). callback
has 1 parameter: < Error >err.
setSinkInputMuteByIndex(< integer >index, < boolean >muted, < function >callback) - (void) - Sets the muted status for a sink input. callback
has 1 parameter: < Error >err.
setSourceOutputMuteByIndex(< integer >index, < boolean >muted, < function >callback) - (void) - Sets the muted status for a source output. callback
has 1 parameter: < Error >err.
setSinkSuspend(< mixed >criteria, < boolean >suspended, < function >callback) - (void) - Sets the suspended status for a sink by either its index (integer) or its name (string). callback
has 1 parameter: < Error >err.
setSourceSuspend(< mixed >criteria, < boolean >suspended, < function >callback) - (void) - Sets the suspended status for a source by either its index (integer) or its name (string). callback
has 1 parameter: < Error >err.
setDefaultSinkByName(< string >name, < function >callback) - (void) - Sets the default sink. callback
has 1 parameter: < Error >err.
setDefaultSourceByName(< string >name, < function >callback) - (void) - Sets the default source. callback
has 1 parameter: < Error >err.
killClientByIndex(< integer >index, < function >callback) - (void) - Terminates the connection of the specified client. callback
has 1 parameter: < Error >err.
killSinkInputByIndex(< integer >index, < function >callback) - (void) - Terminates a sink input. callback
has 1 parameter: < Error >err.
killSourceOutputByIndex(< integer >index, < function >callback) - (void) - Terminates a source output. callback
has 1 parameter: < Error >err.
loadModule(< string >moduleName, < string >argument, < function >callback) - (void) - Load a module. callback
has 1 parameter: < Error >err.
unloadModuleByIndex(< integer >moduleIndex, < function >callback) - (void) - Unload a module. callback
has 1 parameter: < Error >err.
moveSinkInput(< integer >index, < mixed >destSink, < function >callback) - (void) - Moves a sink input to a different sink identified by either its index (integer) or its name (string). callback
has 1 parameter: < Error >err.
moveSourceOutput(< integer >index, < mixed >destSink, < function >callback) - (void) - Moves a source output to a different source identified by either its index (integer) or its name (string). callback
has 1 parameter: < Error >err.
setSinkPort(< mixed >criteria, < string >portName, < function >callback) - (void) - Sets the port for a sink identified by either its index (integer) or its name (string). callback
has 1 parameter: < Error >err.
setSourcePort(< mixed >criteria, < string >portName, < function >callback) - (void) - Sets the port for a source identified by either its index (integer) or its name (string). callback
has 1 parameter: < Error >err.
setCardProfile(< mixed >criteria, < string >profileName, < function >callback) - (void) - Sets the profile for a card identified by either its index (integer) or its name (string). callback
has 1 parameter: < Error >err.
updateClientProperties(< object >properties, < string >mode, < function >callback) - (void) - Updates this client's server-side properties. The update behavior is governed by mode
which can be one of: 'set'
(any/all old properties are removed), 'update'
(only add properties that do not already exist), or 'replace'
(only overwrite property values for existing properties). callback
has 1 parameter: < Error >err.
removeClientProperties(< array >propertyNames, < function >callback) - (void) - Removes specified properties from this client's server-side properties. callback
has 1 parameter: < Error >err.
subscribe(< mixed >events, < function >callback) - (void) - Sets the event subscription for events emitted by the server. events
can either be a string or array of strings containing one or more of: 'none'
, 'all'
, 'sink'
, 'source'
, 'sinkInput'
, 'sourceOutput'
, 'module'
, 'client'
, 'sampleCache'
, 'global'
, or 'card'
. callback
has 1 parameter: < Error >err.
FAQs
PulseAudio client written in pure JavaScript for node.js
The npm package @interactivefitness/paclient receives a total of 1 weekly downloads. As such, @interactivefitness/paclient popularity was classified as not popular.
We found that @interactivefitness/paclient demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.