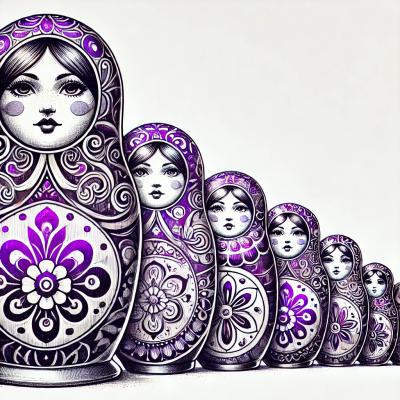
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
@ircam/sc-utils
Advanced tools
Simple generic utilities (type check, common math functions, etc.)
npm install --save @ircam/sc-utils
Convert a linear gain into dB
Alias: linearToDecibel
val
number Value to convertimport { atodb } from '@ircam/sc-utils';
atodb(0);
// > 1
Returns number
Create a counter function.
from
number Start of the counter, included (optional, default 0
)to
number End of the counter, included (optional, default Nmuber.MAX_SAFE_INTEGER
)step
number Increment / decrement step, if 0 returns from
forever (optional, default 1
)Returns Function import { counter } from '@ircam/sc-utils'; const myCounter = counter(0.1, 0.3, 0.1); counter(); // 0.1 counter(); // 0.2 counter(); // 0.3 counter(); // 0.1 // ...
Convert a dB into linear gain
Alias: decibelToLinear
val
number Value to convertimport { dbtoa } from '@ircam/sc-utils';
dbtoa(0);
// > 1
Returns number
Convert a dB into linear gain (i.e. gain)
Alias: dbtoa
val
number Value to convertimport { decibelToLinear } from '@ircam/sc-utils';
decibelToLinear(0);
// > 1
Returns number
Convert a dB into power gain
val
number Value to convertimport { decibelToPower } from '@ircam/sc-utils';
decibelToPower(0);
// > 1
Returns number
Wait for a given number of milliseconds.
See also sleep
ms
Number Number of milliseconds to waitimport { delay } from '@ircam/sc-utils';
// wait for 1 second
await delay(1000);
Returns Promise
Create an exponential scale function.
inputStart
number Start value of input rangeinputEnd
number End value of input rangeoutputStart
number Start value of output rangeoutputEnd
number End value of output rangebase
number Base value for exponential scaling, default to 2
(optional, default 2
)clip
boolean Clip output to output range, default to false
(optional, default false
)const { exponentialScale } = utils;
const midiToFreq = exponentialScale(69, 81, 440, 880);
midiToFreq(57);
// > 220
Convert a frequency in Hz to a MIDI note
freq
number Frequency to convertimport { ftom } from '@ircam/sc-utils';
const freq = ftom(440);
// > 69
Returns number
Provide a unified clock in seconds accross platforms, with an origin defined by the start of the process.
import { getTime } from '@ircam/sc-utils';
setInterval(() => {
const now = getTime();
// ...
}, 1000);
Convert a frequency in Hertz to a normalised one in [0, 1].
Normalised frequency of 1 is half the sample-rate (Nyquist frequency).
frequencyHertz
number Frequency in Hertz to convert
sampleRate
number Twice the Nyquist frequency (optional, default {}
)
sampleRate.sampleRate
(optional, default 2
)import { hertzToNormalised } from '@ircam/sc-utils';
hertzToNormalised(12000, {sampleRate: 48000});
// > 0.5
Returns number
Create a iterator of incrementing ids
DEPRECATED Use the more generic and user friendly counter
instead.
import { idGenerator } from '@ircam/sc-utils';
const generator = idGenerator();
const id = generator.next().value
Returns Iterator
Check if the platform is a browser or a node process
import { isBrowser } from '@ircam/sc-utils';
isBrowser();
// > true|false
Returns boolean
Check if the value is a function
val
any Value to checkimport { isFunction } from '@ircam/sc-utils';
isFunction(() => {});
// > true
Returns boolean
Check if the value is a number, including Infinity. If you want to excluse Infinity, check the native Number.isFinite function
val
any Value to checkimport { isNumber } from '@ircam/sc-utils';
isNumber(42);
// > true
Returns boolean
Check if the value is a Plain Old Javascript Object (POJO)
val
any Value to checkimport { isPlainObject } from '@ircam/sc-utils';
isPlainObject({ a: 1 });
// > true
Returns boolean
Check if the value is a sequence (Array
or TypedArray
) of finite numbers
val
any Value to checkimport { isSequence } from '@ircam/sc-utils';
isSequence([1, 2, 3]);
// > true
Returns boolean
Check if the value is a string
val
any Value to checkimport { isString } from '@ircam/sc-utils';
isString('test');
// > true
Returns boolean
Check if the device supports touch events
import { isTouchDevice } from '@ircam/sc-utils';
isTouchDevice();
// > true|false
Returns boolean
Check if the value is a TypedArray
val
any Value to checkimport { isTypedArray } from '@ircam/sc-utils';
isTypedArray(new Float32Array([1, 2, 3]));
// > true
Returns boolean
Check if the value is a valid URL
url
val
any Value to checkimport { isURL } from '@ircam/sc-utils';
isURL('http://sub.my-site.org/abcd?test=123');
// > true
Returns boolean
Create a linear scale function.
inputStart
number Start value of input rangeinputEnd
number End value of input rangeoutputStart
number Start value of output rangeoutputEnd
number End value of output rangeclip
boolean Clip output to output range, default to false
(optional, default false
)import { scale } from '@ircam/sc-utils';
const myScale = scale(0, 1, 50, 100);
myScale(0.5);
// > 75
Returns Function
Convert a linear gain into dB
Alias: atodb
val
number Value to convertimport { decibelToPower } from '@ircam/sc-utils';
decibelToPower(0);
// > 1
Returns number
Create a logarithmic scale function.
inputStart
number Start value of input rangeinputEnd
number End value of input rangeoutputStart
number Start value of output rangeoutputEnd
number End value of output rangebase
number Base value for logarithmic scaling, default to 2
(optional, default 2
)clip
boolean Clip output to output range, default to false
(optional, default false
)const { logarithmicScale } = utils;
const freqToMidi = logarithmicScale(440, 880, 69, 81);
freqToMidi(220);
// > 57
Convert a MIDI note to frequency
midiNote
number MIDI Note to convertimport { mtof } from '@ircam/sc-utils';
const freq = mtof(69);
// > 440
Returns number
Convert a normalised frequency, in [0, 1], to a frequency in Hertz.
Normalised frequency of 1 is half the sample-rate (Nyquist frequency).
frequencyNormalised
number Normalised frequency to convert
sampleRate
number Twice the Nyquist frequency (optional, default {}
)
sampleRate.sampleRate
(optional, default 2
)import { normalisedToHertz } from '@ircam/sc-utils';
normalisedToHertz(0.5, {sampleRate: 48000});
// > 12000
Returns number
Create a scale function that returns a linearly interpolated value from the given transfert table according to the given normalized position.
import { normalizedToTableScale } from '@ircam/sc-utils'
const scale = normalizedToTableScale([1, 2, 4])
scale(0); // 1
scale(0.25); // 1.5
scale(0.5); // 2
scale(0.75); // 3
scale(1); // 4
Returns function
Convert a linear gain into dB
val
number Value to convertimport { decibelToPower } from '@ircam/sc-utils';
decibelToPower(0);
// > 1
Returns number
Wait for a given number of seconds.
See also delay
sec
Number Number of seconds to waitimport { sleep } from '@ircam/sc-utils';
// wait for 1 second
await sleep(1);
Returns Promise
Create a scale function that returns a normalized position in the transfert table according to the given value.
import { tableToNormalized } from '@ircam/sc-utils'
const scale = tableToNormalized([1, 2, 4])
scale(1); // 0
scale(1.5); // 0.25
scale(2); // 0.5
scale(3); // 0.75
scale(4); // 1
Returns function
FAQs
Set of simple generic utilities (type check, common math functions, etc.)
The npm package @ircam/sc-utils receives a total of 157 weekly downloads. As such, @ircam/sc-utils popularity was classified as not popular.
We found that @ircam/sc-utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.