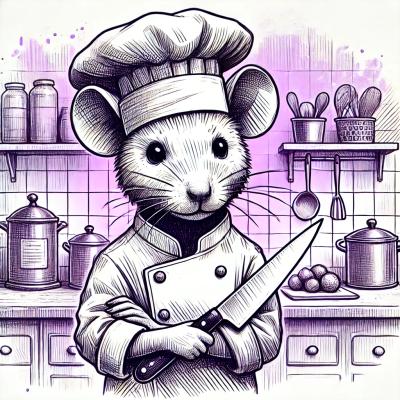
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@isnolan/nestjs-payment
Advanced tools
nestjs-payment is a comprehensive NestJS module for simplified subscription management and seamless integration with popular payment platforms.
SubscriptionModule
is a powerful NestJS module designed to streamline subscription management by providing seamless integration with popular payment platforms such as Stripe, Google Pay, and Apple Pay. It simplifies the handling of subscription events via webhooks, offering a unified solution for verifying and processing payment notifications securely.
Using PNPM Install the package along with the Stripe peer dependency:
pnpm install --save @isnolan/nestjs-payment
Synchronous Configuration To utilize SubscriptionModule, import and add it to the imports array of your NestJS module, typically AppModule. Here's a synchronous configuration example:
import { SubscriptionModule } from '@isnolan/nestjs-payment';
@Module({
imports: [
SubscriptionModule.forRoot({
stripe: {
apiSecretKey: `${process.env.STRIPE_SECRET_KEY}`,
webhookSecret: `${process.env.STRIPE_SECRET_WEBHOOK}`,
},
apple: {
signingKey: `${process.env.APPLE_SIGNING_KEY}`,
keyId: `${process.env.APPLE_KEY_ID}`,
issuerId: `${process.env.APPLE_ISSUER_ID}`,
bundleId: `${process.env.APPLE_BUNDLE_ID}`,
environment: 'Sandbox',
},
}),
],
providers: [AppService],
})
export class AppModule {}
Asynchronous Configuration SubscriptionModule also supports asynchronous configuration, useful for determining configurations dynamically at runtime:
import { SubscriptionModule } from '@isnolan/nestjs-payment';
import { ConfigService } from '@nestjs/config';
@Module({
imports: [
SubscriptionModule.forRootAsync({
inject: [ConfigService],
useFactory: async (config: ConfigService) => config.get('subscription'),
}),
],
providers: [AppService],
})
export class AppModule {}
The webhook URLs are as follows:
Follow the instructions from the App Store Documentation for remaining integration steps such as testing your integration with the CLI before you go live and properly configuring the endpoint from the Stripe dashboard so that the correct events are sent to your NestJS app.
Follow the instructions from the Google Play Documentation for remaining integration steps such as testing your integration with the CLI before you go live and properly configuring the endpoint from the Stripe dashboard so that the correct events are sent to your NestJS app.
Follow the instructions from the Stripe Documentation for remaining integration steps such as testing your integration with the CLI before you go live and properly configuring the endpoint from the Stripe dashboard so that the correct events are sent to your NestJS app.
It will be very complicated to connect the subscription interfaces and webhooks of Google Play, App Store, and Stripe at the same time, and we will have to spend more time to unify the interfaces. In order to simplify development, we abstracted and aggregated the events of three of them and defined our key events and state here:
Event | Description |
---|---|
SUBSCRIBED | Indicates that the user has created a new subscription, including re-subscription after cancellation. |
RENEWED | Indicates that the user's active subscription has entered a new cycle through renewal. |
GRACE_PERIOD | Indicates a grace period where the subscription is still considered active but payment has not been received yet. This typically occurs after a payment failure or during a trial period extension. |
EXPIRED | Indicates that the subscription has reached its expiration date and is no longer active. |
CANCELLED | Indicates that the user has voluntarily cancelled their subscription. |
UNHANDLED | Indicates an event that hasn't been processed or recognized by the module. |
State | Description |
---|---|
Active | Represents the active state of a subscription, indicating that the user has access to the subscribed services or content. |
Paused | Indicates that the subscription is temporarily paused, during which the user may not have access to the subscribed services or content but retains their subscription benefits. |
Expired | Represents the state where the subscription has reached its expiration date and is no longer active. |
Cancelled | Indicates that the subscription has been cancelled, either by the user or due to non-payment, and access to the subscribed services or content has been revoked. |
import { OnSubscriptionEvent, OnSubscriptionOriginalEvent, StripeProviderService } from '@isnolan/nestjs-payment';
import { Injectable } from '@nestjs/common';
@Injectable()
export class AppService {
constructor(private readonly stripe: StripeProviderService) {}
// All unified events
@OnSubscriptionEvent({ event: 'all' })
handleAppleSubsriptionSuccess(data: any) {
console.log(`[unified]all:`, data);
}
// specified unified event
@OnSubscriptionEvent({ event: 'SUBSCRIBED' })
handleAppleSubsriptionSuccess(data: any) {
console.log(`[unified]SUBSCRIBED:`, data);
}
// All provider event
@OnSubscriptionOriginalEvent({ provider: 'all', event: 'all' })
handleALLEventSuccess(data: any) {
console.log(`[all]all:`, data);
}
// Unified events for all provider
@OnSubscriptionOriginalEvent({ provider: 'all', event: 'RENEWED' }) //
handleStripeSubsriptionSuccess(data: any) {
console.log(`[all]RENEWED:`, data);
}
// Original events of the specified provider
@OnSubscriptionOriginalEvent({ provider: 'all', event: 'invoice.paid' }) // origin event
handleStripeOriginEventSuccess(data: any) {
console.log(`[all]invoice.paid:`, data);
}
// All events on the specified provider
@OnSubscriptionOriginalEvent({ provider: 'apple', event: 'all' })
handleAppleSubsriptionSuccess(data: any) {
console.log(`[apple]all:`, data);
}
}
import {SubscriptionService } from '@isnolan/nestjs-payment';
@Injectable()
export class AppService {
constructor(
private readonly subscription: SubscriptionService,
) {}
@Post('receipt')
async validateReceipt(@Body() payload: ValidateReceiptDto) {
const { platform, purchase_token } = payload;
const subscription = await this.subscription.validateReceipt(platform, purchase_token);
console.log(`[purchase]receipt`, subscription);
}
}
In order to comply with the general rules of Stripe, Google Play, and Apple Play. You may have to store two sets of purchased product IDs in your business database and use the corresponding IDs in Mobile and Web.
This is because:
FAQs
nestjs-payment is a comprehensive NestJS module for simplified subscription management and seamless integration with popular payment platforms.
We found that @isnolan/nestjs-payment demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.