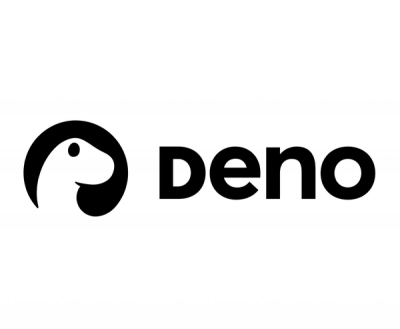
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@isvend/capacitor-udp
Advanced tools
UDP plugin for capacitor
npm install @isvend/capacitor-udp
npx cap sync
create(...)
update(...)
bind(...)
send(...)
close(...)
closeAllSockets()
setBroadcast(...)
setPaused(...)
getInfo(...)
getSockets()
joinGroup(...)
leaveGroup(...)
getJoinedGroups()
setMulticastTimeToLive(...)
setMulticastLoopbackMode(...)
addListener('receive', ...)
addListener('receiveError', ...)
removeAllListeners()
UDP socket plugin for Capacitor.
Only available on Android and iOS.
create(options?: CreateOptions | undefined) => Promise<CreateResult>
Create a socket for udp, and you can create more than one differentiated by the socket id.
Only available on Android and iOS.
Param | Type |
---|---|
options | CreateOptions |
Returns: Promise<CreateResult>
Since: 5.0.0
update(options: UpdateOptions) => Promise<void>
Update the socket info including socket name and buffer size.
Only available on Android and iOS.
Param | Type |
---|---|
options | UpdateOptions |
Since: 5.0.0
bind(options: BindOptions) => Promise<void>
You need to bind a socket before sending and receiving data.
Only available on Android and iOS.
Param | Type |
---|---|
options | BindOptions |
Since: 5.0.0
send(options: SendOptions) => Promise<void>
Send udp data
Only available on Android and iOS.
Param | Type |
---|---|
options | SendOptions |
Since: 5.0.0
close(options: CloseOptions) => Promise<void>
Close one socket
Only available on Android and iOS.
Param | Type |
---|---|
options | CloseOptions |
Since: 5.0.0
closeAllSockets() => Promise<void>
Close All Sockets
Only available on Android and iOS.
Since: 5.0.0
setBroadcast(options: SetBroadcastOptions) => Promise<void>
After enabling broadcasting, you can send data with target address 255.255.255.255.
Only available on Android and iOS.
Param | Type |
---|---|
options | SetBroadcastOptions |
Since: 5.0.0
setPaused(options: SetPausedOptions) => Promise<void>
Pause receiving data.
Only available on Android and iOS.
Param | Type |
---|---|
options | SetPausedOptions |
Since: 5.0.0
getInfo(options: InfoOptions) => Promise<InfoResult>
Get Socket information
Only available on Android and iOS.
Param | Type |
---|---|
options | InfoOptions |
Returns: Promise<InfoResult>
Since: 5.0.0
getSockets() => Promise<GetSocketsResult>
Obtain all the sockets available.
Only available on Android and iOS.
Returns: Promise<GetSocketsResult>
Since: 5.0.0
joinGroup(options: JoinGroupOptions) => Promise<void>
Join a particular group address. For IPv4, it's like "238.12.12.12". For IPv6, it's like "ff02::08".
Only available on Android and iOS.
Param | Type |
---|---|
options | JoinGroupOptions |
Since: 5.0.0
leaveGroup(options: LeaveGroupOptions) => Promise<void>
Leave a particular group address. For IPv4, it's like "238.12.12.12". For IPv6, it's like "ff02::08".
Only available on Android and iOS.
Param | Type |
---|---|
options | LeaveGroupOptions |
Since: 5.0.0
getJoinedGroups() => Promise<GetJoinedGroupsResult>
Get joined groups
Only available on Android and iOS.
Returns: Promise<GetJoinedGroupsResult>
Since: 5.0.0
setMulticastTimeToLive(options: SetMulticastTimeToLiveOptions) => Promise<void>
Set the time to live (TTL) for multicast packets
Only available on Android and iOS.
Param | Type |
---|---|
options | SetMulticastTimeToLiveOptions |
Since: 5.0.0
setMulticastLoopbackMode(options: SetMulticastLoopbackModeOptions) => Promise<void>
Set whether to enable multicast loopback mode
Only available on Android and iOS.
Param | Type |
---|---|
options | SetMulticastLoopbackModeOptions |
Since: 5.0.0
addListener(eventName: 'receive', listenerFunc: (event: ReceiveEvent) => void) => Promise<PluginListenerHandle> & PluginListenerHandle
Listening for data reception events
Only available on Android and iOS.
Param | Type |
---|---|
eventName | 'receive' |
listenerFunc | (event: ReceiveEvent) => void |
Returns: Promise<PluginListenerHandle> & PluginListenerHandle
Since: 5.0.0
addListener(eventName: 'receiveError', listenerFunc: (event: ReceiveEvent) => void) => Promise<PluginListenerHandle> & PluginListenerHandle
Listening for data reception exception events
Only available on Android and iOS.
Param | Type |
---|---|
eventName | 'receiveError' |
listenerFunc | (event: ReceiveEvent) => void |
Returns: Promise<PluginListenerHandle> & PluginListenerHandle
Since: 5.0.0
removeAllListeners() => Promise<void>
Remove all native listeners for this plugin
Only available on Android and iOS.
Since: 5.0.0
Result of creating a UDP socket
Prop | Type | Description |
---|---|---|
socketId | number | The id of the socket |
ipv4 | string | ipv4 address |
ipv6 | string | ipv6 address |
Parameters for creating a UDP socket
Prop | Type |
---|---|
properties | { name?: string; bufferSize?: number; } |
Parameters for updating a UDP socket
Prop | Type | Description |
---|---|---|
socketId | number | The id of the socket |
properties | { name?: string; bufferSize?: number; } |
Parameters for binding a UDP socket
Prop | Type | Description |
---|---|---|
socketId | number | The id of the socket |
address | string | The address to bind to. If not specified, the socket will be bound to the wildcard address. |
port | number | The port to bind to. |
Parameters for sending data
Prop | Type | Description |
---|---|---|
socketId | number | The id of the socket |
address | string | The address of the remote host. |
port | number | The port of the remote host. |
buffer | string | The data to send. |
Parameters for closing a UDP socket
Prop | Type | Description |
---|---|---|
socketId | number | The id of the socket |
Parameters for settings broadcast mode
Prop | Type | Description |
---|---|---|
socketId | number | The id of the socket |
enabled | boolean | Whether to enable broadcast mode. |
Parameters for binding a UDP socket
Prop | Type | Description |
---|---|---|
socketId | number | The id of the socket |
paused | boolean | Whether to pause receiving data |
Result of getting information about a UDP socket
Prop | Type | Description |
---|---|---|
socketId | number | The id of the socket |
name | string | null | The name of the socket, which can be used to distinguish between multiple sockets. |
bufferSize | number | The size of the buffer used to receive data. |
paused | boolean | Whether data reception has been suspended。 |
localAddress | string | The address to which the socket is bound. |
localPort | number | The port to which the socket is bound. |
Parameters for getting information about a UDP socket
Prop | Type | Description |
---|---|---|
socketId | number | The id of the socket |
Parameters for getting information about all UDP sockets
Prop | Type | Description |
---|---|---|
sockets | InfoResult[] | The list of UDP sockets |
Parameters for joining a multicast group
Prop | Type | Description |
---|---|---|
socketId | number | The id of the socket |
address | string | The address of the multicast group to join. For IPv4, it's like "238.12.12.12". For IPv6, it's like "ff02::08". |
Parameters for leaving a multicast group
Prop | Type | Description |
---|---|---|
socketId | number | The id of the socket |
address | string | The address of the multicast group to leave. For IPv4, it's like "238.12.12.12". For IPv6, it's like "ff02::08". |
Parameters for getting joined multicast groups
Prop | Type | Description |
---|---|---|
groups | [string] | The list of multicast group addresses |
Parameters for setting multicast time to live
Prop | Type | Description |
---|---|---|
socketId | number | The id of the socket |
ttl | number | The time to live value. |
Parameters for setting multicast loopback mode
Prop | Type | Description |
---|---|---|
socketId | number | The id of the socket |
enabled | boolean | Whether to enable multicast loopback mode. |
Prop | Type |
---|---|
remove | () => Promise<void> |
Result of receiving data
Prop | Type | Description |
---|---|---|
socketId | number | The id of the socket |
buffer | string | The data received. |
remoteAddress | string | The address of the remote host. |
remotePort | number | The port of the remote host. |
error | string | Error message |
FAQs
UDP plugin for capacitor
The npm package @isvend/capacitor-udp receives a total of 0 weekly downloads. As such, @isvend/capacitor-udp popularity was classified as not popular.
We found that @isvend/capacitor-udp demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.