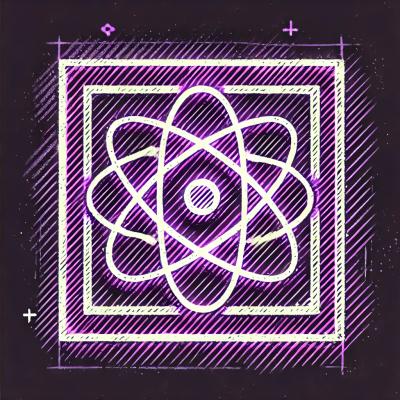
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
@itrocks/class-type
Advanced tools
Helper types and functions to identify, validate, and manipulate classes, objects, prototypes and their properties
Helper types and functions to identify, validate, and manipulate classes, objects, prototypes and their properties.
npm install @itrocks/class-type
The library is published in both CommonJS and ESModule formats and includes TypeScript type definitions.
This library provides utility types and functions to work more seamlessly with JavaScript/TypeScript classes, objects,
and their constructors. It helps identify, inspect, and manipulate objects and their associated class types at runtime.
In particular, @itrocks/class-type
focuses on classes and objects where properties are strings.
For domain objects, this means that numeric and symbol properties are not supported, ensuring stronger type safety and
predictability in handling object structures.
type AnyObject = Record<string, any>
Represents a generic object with string keys and values of any type.
type KeyOf<T> = Extract<keyof T, string>
KeyOf<T>
is similar to keyof T
, but it filters out non-string keys, ensuring that only string-based property names
of T
are extracted.
This is particularly useful when working with domain objects where properties are expected to be strings.
Example without KeyOf<T>
:
function displayValueOf(object: object, property: string)
{
console.log('property ' + property, object[property])
}
This code will cause a TypeScript error (TS7053),
because property
is not strongly linked to the object
parameter's type.
Example with KeyOf<T>
:
function displayValueOf<T extends object>(object: T, property: KeyOf<T>)
{
console.log('property ' + property, object[property])
}
By using KeyOf<T>
, the property
argument must be a valid string key of object
, providing stronger type safety.
Using keyof T
alone would have caused a TypeScript error (TS2469), since property
could have been a Symbol
,
which does not support the +
operator.
type ObjectOrType<T extends object = object> = T | Type<T>
Represents either an instance of T
or its class type Type<T>
.
Example:
function doSomething(arg: ObjectOrType) {}
doSomething(new Object) // instance of object
doSomething(Object) // constructor for Object
doSomething(class {}) // Anonymous class constructor
All these calls are valid and type-checked by TypeScript.
type StringObject = Record<string, string>
Represents an object where both keys and values are strings.
type Type<T extends object = object> = new (...args: any[]) => T
This type helper represents a class for objects of type T
.
Note that in JavaScript, classes are represented as constructors.
function addReservedClassNames(...classNames: string[])
Registers specific class names as reserved. Reserved class names are treated by baseType as if they were unnamed, allowing you to skip certain dynamically generated or overloaded classes when determining the base class.
This is particularly useful in frameworks or applications that create intermediate class layers with predefined names that should be ignored.
Parameters:
...classNames
: A list of class names (string[]
) to mark as reserved.Example:
import { addReservedClassNames, baseType } from './class-type'
class Foo {}
class Bar extends Foo {}
console.log(baseType(Bar).name) // Outputs 'Bar'
// Mark 'Bar' as a reserved class name
addReservedClassNames('Bar')
console.log(baseType(Bar).name) // Outputs 'Foo'
In this example:
addReservedClassNames
, baseType
identifies Bar
as the base class.addReservedClassNames('Bar')
, the function treats Bar
as a reserved class name,
so it continues traversing the inheritance chain and identifies Foo
as the base class.function baseType<T extends object>(target: Type<T>): Type<T>
Returns the base type (class/constructor) of the given class by walking up its prototype chain until it finds a
meaningful named constructor (not an empty name).
This is especially useful if your framework or application generates anonymous class wrappers
(e.g., proxy classes or dynamically built classes without a proper name).
Calling baseType
helps you retrieve the original named class or its most relevant ancestor in the inheritance chain.
To mark certain class names as reserved words, use addReservedClassNames.
These names will be treated by baseType
as non-base classes, just as if they were unnamed.
Parameters:
target
: The class constructor from which to retrieve the base type.Returns:
Type<T>
of the given target.Example:
class OriginalClass {}
const AnonymousClass = (() => class extends OriginalClass {})()
console.log(baseType(AnonymousClass)) // Outputs: OriginalClass
function inherits(type: Type, superType: Type): boolean
Checks if a class (or Type) is derived from another class class, mimicking the behaviour of instanceof, but operating at the class level.
Parameters:
type
: The class whose inheritance chain will be checkedsuperType
: The parent class to check against.Returns:
boolean
: Returns true
if type
is equal to or inherits from superType
, otherwise false
.function isAnyFunction(value: any): value is Function
Checks if the given value is a function (excluding class constructors).
Parameters:
value
: The value to check.Returns:
true
if value is a function, false
otherwise.function isAnyFunctionOrType(value: any): value is Function | Type
Checks if the given value is either a function or a class constructor.
Parameters:
value
: The value to check.Returns:
true
if value is a function or class constructor, false
otherwise.function isAnyObject<T extends object = object>(value: any): value is T
Checks if the given value is an object (excluding null
).
Parameters:
value
: The value to check.Returns:
true
if value
is a non-null object, false
otherwise.function isAnyType(value: any): value is Type
Checks if the given value is a class type (i.e., a function that is recognized as a class constructor).
Parameters:
value
: The value to check.Returns:
true
if value
is a class constructor, false
otherwise.function isObject<T extends object>(target: ObjectOrType<T>): target is T
Checks if target
is an object instance rather than a class constructor (i.e. a Type).
This function is similar to isAnyObject, but it is optimized for cases where the argument is already known to be either an object instance or a class Type.
Parameters:
target
: Either an object or a class constructor.Returns:
true
if target is an T
object instance, false
if it is a Type.function isType<T extends object>(target: ObjectOrType<T>): target is Type<T>
Checks if target
is a class constructor (i.e. a Type) rather than an object instance.
This function is similar to isAnyType, but it is optimized for cases where the argument is already known to be either an object instance or a class Type.
Parameters:
target
: Either an object or a class constructor.Returns:
true
if target is a Type (i.e. class constructor), false
if it is a T
object instance.function prototypeOf<T extends object>(target: ObjectOrType<T>): T
Returns target
as a prototype.
If target
is a class constructor (i.e. Type, it returns target.prototype
.
If target
is an object instance, it returns target
itself.
Parameters:
target
: Either an object or a class constructor.Returns:
function typeIdentifier(type: Type): Symbol
Returns a Symbol for the given class constructor (i.e. Type).
Parameters:
type
: The class constructor.Returns:
function typeOf<T extends object>(target: ObjectOrType<T>): Type<T>
Retrieves the Type (i.e. class constructor) for target
.
If target
is an object, it returns its Type;
if target
is already a Type, it returns it as-is.
Parameters:
target
: Either an object or a Type (i.e. class constructor).Returns:
Type<T>
representing the Type of target
.FAQs
Helper types and functions to identify, validate, and manipulate classes, objects, prototypes and their properties
The npm package @itrocks/class-type receives a total of 126 weekly downloads. As such, @itrocks/class-type popularity was classified as not popular.
We found that @itrocks/class-type demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.