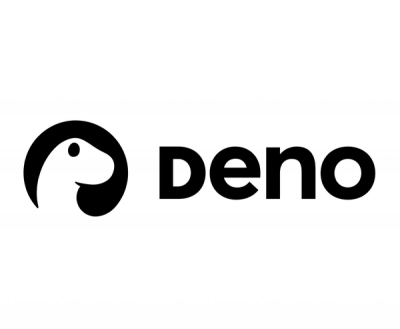
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@itsmapleleaf/gatekeeper
Advanced tools
a slash command and interaction framework for discord.js
this is a slash command framework for discord.js and it's very not done don't use it
maybe more docs later
import {
actionRowComponent,
applyCommands,
buttonComponent,
CommandHandler,
} from "@itsmapleleaf/gatekeeper"
import { Client, Intents } from "discord.js"
const commands: CommandHandler[] = [
// list of commands here
]
const client = new Client({
intents: [Intents.FLAGS.GUILDS],
})
applyCommands(client, commands)
client.on("ready", () => {
console.info("bot running ayy lmao")
})
await client.login(process.env.BOT_TOKEN).catch(console.error)
const commands: CommandHandler[] = [
{
name: "ping",
description: "pong",
async run(context) {
await context.addReply("pong!")
},
},
]
creates a /counter
command which responds with a counter button. each counter button has its own count. try running it multiple times!
const commands: CommandHandler[] = [
{
name: "counter",
description: "make a counter",
async run(context) {
const reply = await context.addReply("ok one sec")
let times = 0
while (true) {
const counterId = `counter-${Math.random()}`
const doneId = `done-${Math.random()}`
await reply.edit(
`button pressed ${times} times`,
actionRowComponent(
buttonComponent({
style: "PRIMARY",
label: "press it",
customId: counterId,
}),
buttonComponent({
style: "PRIMARY",
label: "i'm bored",
customId: doneId,
})
)
)
const interaction = await context.waitForInteraction()
if (interaction?.customId === counterId) {
times += 1
}
if (interaction?.customId === doneId) {
break
}
}
await reply.edit("well fine then")
},
},
]
FAQs
a slash command and interaction framework for discord.js
The npm package @itsmapleleaf/gatekeeper receives a total of 1 weekly downloads. As such, @itsmapleleaf/gatekeeper popularity was classified as not popular.
We found that @itsmapleleaf/gatekeeper demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.