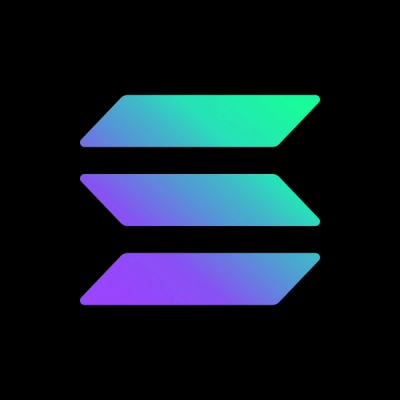
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
@ixo/client-sdk
Advanced tools
A complete package of client software for developing client applications which connect to the ixo software stack and build on the Internet of Impact.
const {makeWallet, makeClient} = require('@ixo/client-sdk')
const
wallet = await makeWallet(),
client = makeClient(wallet)
await client.register()
await client.sendTokens('<target address>', 10)
const someProject = await client.getProject('<a valid project DID>')
const someClaims = await client.listClaims(someProject)
console.log('Here are the claims', someClaims)
See wallet API and client API for details.
makeWallet(source, didPrefix = 'did:ixo:')
: Create a new wallet
source
: Either a mnemonic string or a plain object
representing a wallet state (possibly obtained via toJSON()
-see below for details). Optional.
If empty, a brand new wallet is generated. If a mnemonic string, recovers a wallet based on the mnemonic. If a state object, revives the wallet using that state.
didPrefix
: A DID prefix. Optional, defaults to did:ixo:
.
If the source
is a mnemonic which means that you are importing
a wallet from elsewhere, you may need to provide the didPrefix
as well to obtain the exact same wallet. If the DID prefix of
the wallet is different from did:ixo:
then it must be provided
here.
makeWallet
returns a wallet object with the following
properties:
agent
: A custom wallet instance implementing OfflineAminoSigner
toJSON()
: Standard
toJSON
method for JSON.stringify
integration. One can also use this
to get a representation of the wallet's internal state as a
plain object and use it in a context where a class instance is
not supported. (e.g. Global application state of a client app)
makeClient
to create a new clientClient methods:
register
getSecpAccount
getAgentAccount
balances
sendTokens
getDidDoc
listProjects
listTemplates
listCells
getProject
getTemplate
getCell
createProject
updateProject
createEntityFile
getEntityFile
updateProjectStatus
getProjectFundAddress
listAgents
createAgent
updateAgent
listClaims
createClaim
evaluateClaim
staking.listValidators
staking.getValidator
staking.myDelegations
staking.pool
staking.validatorDistribution
staking.delegatorValidatorRewards
staking.delegation
staking.delegatorDelegations
staking.delegatorUnbondingDelegations
staking.delegatorRewards
staking.delegate
staking.undelegate
staking.redelegate
bonds.byId
bonds.list
bonds.buy
bonds.sell
custom
makeClient(signer, blockchainURL, blocksyncURL)
: Create a new ixo client
signer
: Either a wallet object created by
makeWallet
or a custom signer object with the
following properties:
secp
: Object implementing OfflineAminoSigner
agent
: Object implementing OfflineAminoSigner with the following extra property:
did
See the wallet API for more info on secp
and
agent
keywords.
Optional. If empty, client methods that require a signer won't work.
blockchainURL
: The URL of the target ixo chain. Optional,
defaults to the current mainnet URL.
blocksyncURL
: The URL of the target ixo blocksync service.
Optional, defaults to the current main blocksync service URL.
register(pubKey)
: Register the current user
pubKey
: Only required if the client is configured to use
a custom signer. No need to provide this if the client is
initialized with a wallet created with
makeWallet
, as the value can be obtained from
it.
Note: Registering triggers a write operation on the
blockchain which requires a gas fee in terms of ixo tokens to
be paid. So the account you are trying to register has to have
some ixo tokens. To credit accounts on the testnet you can use
the faucet.
The specific address you will need to use is the address of
the agent subwallet. Get it with wallet.agent.getAccounts
.
See wallet API for more info.
getSecpAccount()
: Get account info for the SECP subwallet
getAgentAccount()
: Get account info for the agent subwallet
sendTokens(targetAddress, amount, denom)
targetAddress
: A wallet address to send coins to
amount
: Any positive number
denom
: Coin type. Optional, defaults to "uixo"
.
getDidDoc(did)
: Return the full DID document for the given DID
listProjects()
: Lists all available projects
listTemplates()
: Lists all available templates
listCells()
: Lists all available cells
getProject(projectDid)
: Get complete project record for the given DID
getTemplate(tplDid)
: Get complete template record for the given DID
getCell(cellDid)
: Get complete cell record for the given DID
createProject(projectData, cellnodeURL)
: Create a new project
projectData
: To be documented; for now please see
here
for an example
cellnodeURL
: URL of the cell node where various project
data will be kept. Optional, defaults to the URL of ixo's
shared cell node
updateProject(projectDocUpdates, cellnodeURL)
: Update an existing project
projectDocUpdates
: Updates to be made to the project
document: A subset of the projectData
argument of the
createProject
method above that satisfies the rules
described in the following link:
https://github.com/ixofoundation/ixo-blockchain/pull/230#issue-902602327
cellnodeURL
: URL of the cell node where various project
data will be kept. Optional, defaults to the URL of ixo's
shared cell node
createEntityFile(target, dataUrl)
: Upload a file to entity's cell node
target
: Either an entity record, an entity DID, or a cell
node URL
key
: Key of the target file, as returned from
createProjectFile
.
updateProjectStatus(target, status)
target
: Either a project record or a project DID
status
: "CREATED"
| "PENDING"
| "FUNDED"
| "STARTED"
| "STOPPED"
| "PAIDOUT"
Note I: Status is a state machine in that you can only
update it in the order seen above. So "CREATED"
-> "PENDING"
is a valid transition while "CREATED"
-> "STARTED"
is not.
Note II: Before setting a project's status to FUNDED
, you
have to send some tokens to the project's wallet first. If you
don't do that before trying to set the status to FUNDED
, you
won't get an error but the status won't be changed either. This
is because of a poor error handling logic in the ixo backend
which is going to be fixed as soon as possible. For now just
please keep this in mind. Use getProjectFundAddress
below to
get the wallet address to send tokens to.
Use getProjectFundAddress
below to get the wallet address to
send tokens to.
getProjectFundAddress(projectDid)
listAgents(projectRecordOrDid)
: List agents belonging to a given project
createAgent(projectRecordOrDid, agentRecord)
: Create an agent for the given project
agentRecord
: An object with the following properties:
did
: The DID of an already registered user
email
: Agent email
name
: Agent name
role
: Any one of the following:
"PO"
for owner"EA"
for evaluator"SA"
for service provider"IA"
for investorNote: The new agent will need to be approved before they
can start their activity. See updateAgent
below:
updateAgent(projectRecordOrDid, agentDid, updates)
updates
: An object with the following properties:
status
(Required): Any one of the following:
0
for pending1
for approved2
for revoked3
for invitedrole
(Required): See the role
property under createAgent
version
: Optional
listClaims(projectRecordOrDid, projectTemplateId)
projectTemplateId
: Optional, provide a value to filter claims
by template idcreateClaim(projectRecordOrDid, templateRecordOrDid, claimItems, opts)
claimItems
: Array of objects that has the following schema:
id
: Id of the claim item. Must match the value in the
claim template.
attribute
: Attribute of the claim item. Must match the
value in the claim template.
value
: Value of the claim item.
opts
: An object with the following properties:
dryRun
: Boolean, default false
. If true
, Instead of
creating the claims, returns the full HTTP request that will
create the claims.evaluateClaim(projectRecordOrDid, claimId, status)
claimId
: A claim id as returned from createClaim
status
: Any one of the following:
0
for pending1
for approved2
for rejectedstaking
:
An object that groups the following staking related methods:
See here for a description of the url params of the related endpoint, and its return value.
getValidator(validatorAddress)
validatorDistribution(validatorAddress)
delegatorValidatorRewards(delegatorAddress, validatorAddress)
delegation(delegatorAddr, validatorAddress)
delegatorDelegations(delegatorAddress)
delegatorUnbondingDelegations(delegatorAddress)
delegatorRewards(delegatorAddress)
delegate(validatorAddress, amount)
undelegate(validatorAddress, amount)
redelegate(validatorSourceAddress, validatorDestinationAddress)
bonds
:
An object that groups the following bond related methods:
custom(walletType, msg)
: Send a custom message to the blockchain
walletType
: Either "secp"
or "agent"
. See the wallet API
for more info
msg
: See
here
for available options
Put the ixo-client-sdk
string into your DEBUG
environment
variable to log network requests and responses. See the debug
package for more info.
FAQs
One ixo client to rule them all
We found that @ixo/client-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.