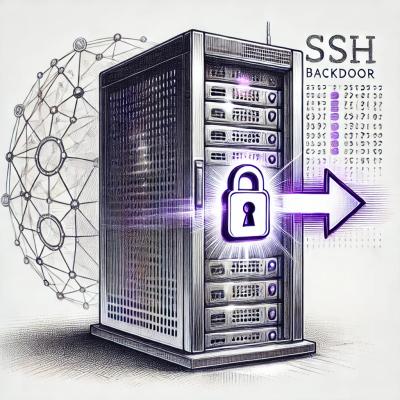
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@janiscommerce/model
Advanced tools
npm install @janiscommerce/model
The session injection is useful when you have a dedicated database per client.
Using the public setter session
, the session will be stored in the controller
instance.
All the controllers and models getted using that controller will have the session injected.
The Model
uses Database Dispatcher for getting the correct DBDriver for a Model
.
The DBDriver will perform all the queries to the database.
If you have the connection settings you should add a databaseKey
getter in you Model
.
class MyModel extends Model {
get databaseKey() {
return 'core';
}
}
Database Dispatcher will try to use one of the following settings
Using Settings, with settings in file /path/to/root/.janiscommercerc.json
:
{
"database": {
"core": {
"host": "http://my-host-name.org",
"type": "mysql",
// ...
}
}
}
When your Model
is a Client Model, and the database connection settings are in the injected session, you don't need to configurate the databaseKey
.
You can add database connection settings by adding the field names from the received client that contains the settings, with the setting what will be passed to the DBDriver. Also you can add config for read/write databases.
Example of settings:
// .janiscommercerc.json
{
"databaseWriteType" : "someDBDriver",
"databaseReadType": "someOtherDBDriver",
"clients": {
"database": {
"fields": {
"databaseKey": "core",
"table": "clients",
"identifierField": "code",
"read": {
"dbReadHost" : "host",
"dbReadProtocol" : "protocol",
"dbReadPort" : "port",
"elasticSearchPrefix" : "name",
"elasticSearchAws" : "awsCredentials"
},
"write": {
"dbWriteHost" : "host",
"dbWriteProtocol" : "protocol",
"dbWriteDatabase" : "database",
"dbWriteUser" : "user",
"dbWritePassword" : "password",
"dbWritePort" : "port"
}
}
}
}
}
/*
Received client:
{
"name": "someclient",
"dbReadHost": "http://localhost",
"dbReadPort": 27017,
"elasticSearchPrefix": "someclient",
"elasticSearchAws": true
}
Database connection settings:
{
"host": "http://localhost",
"port": 27017,
"prefix": "someclient",
"awsCredentials": true
}
*/
shouldCreateLogs (static getter).
Returns if the model should log the write operations. Default: true
. For more details about logging, read the logging section.
excludeFieldsInLog (static getter).
Returns the fields that will be removed from the logs as an array of strings. For example: ['password', 'super-secret']
. For more details about logging, read the logging section.
statuses (class getter).
Returns an object
with the default statuses (active
/ inactive
)
console.log(Model.statuses);
/*
{
active: 'active',
inactive: 'inactive'
}
*/
params
is an optional Object with filters, order, paginator.
const items = await myModel.get({ filters: { status: 'active' } });
id
is an array, or an object otherwise.id
is required. It can be one ID or an array of IDs
params
is an optional Object with filters, order, paginator.
const items = await myModel.getById(123, { filters: { status: 'active' } });
value
is an array, or an object otherwise.field
is required. A string as a field
value
is required. It can be one value or an array of values
params
is an optional Object with filters, order, paginator.
const items = await myModel.getBy(orderId, 123, { filters: { status: 'active' } });
params
See get() method
callback
A function to be executed for each page. Receives three arguments: the items found, the current page and the page limit
await myModel.getPaged({ filters: { status: 'active' } }, (items, page, limit) => {
// items is an array with the result from DB
});
get()
sometimes you need data of totals. This method returns an object with that information.Result object structure: pages: The total pages for the filters applied page: The current page limit: The limit applied in get total: The total number of items in DB for the applied filters
await myModel.get({ filters: { status: 'active' } });
const totals = await myModel.getTotals();
/**
totals content:
{
pages: 3,
page: 1,
limit: 500,
total: 1450
}
*/
referenceIds
and values: id
, only those founds.Array<strings>
List of References Ids
await myModel.mapIdByReferenceId(['some-ref-id', 'other-ref-id', 'foo-ref-id']);
/**
{
some-ref-id: 'some-id',
foo-ref-id: 'foo-id'
}
*/
params
is an optional Object with filters.
const uniqueValues = await myModel.distinct('status');
const uniqueValues = await myModel.distinct('status', {
filters: {
type: 'some-type'
}
});
await myModel.insert({ foo: 'bar' });
const items = await myModel.get({ filters: { foo: 'bar' }});
/**
itemInserted content:
[
{
foo: 'bar'
}
//...
]
*/
setOnInsert
to add default values on Insert, optionalawait myModel.save({ foo: 'bar' }, { status: 'active' });
const items = await myModel.get({ filters: { foo: 'bar' }});
/**
items content:
[
{
foo: 'bar',
status: 'active'
}
//...
]
*/
filter
.await myModel.update({ updated: 1 }, { status: 5 });
// will set updated = 1 for the items that has status = 5
await myModel.remove({ foo: 'bar' });
const items = await myModel.get({ filters: { foo: 'bar' }});
/**
items content:
[]
*/
await myModel.multiInsert([{ foo: 1 }, { foo: 2 }]);
const items = await myModel.get();
/**
items content:
[
{ foo: 1 },
{ foo: 2 }
]
*/
setOnInsert
to add default values on Insert, optionalawait myModel.multiSave([{ foo: 1 }, { foo: 2, status: 'pending' }], { status: 'active' });
const items = await myModel.get();
/**
items content:
[
{ foo: 1, status: 'active' },
{ foo: 2, status: 'pending' }
]
*/
await myModel.multiRemove({ status: 2 });
const items = await myModel.get({ filters: { status: 2 }});
/**
items content:
[]
*/
await myModel.increment({ uniqueIndex: 'bar' }, { increment: 1, decrement: -1 });
/**
before:
items content:
[
{
increment: 1,
decrement: 2
}
//...
]
after:
items content:
[
{
increment: 2,
decrement: 1
}
//...
]
*/
Only if Database support it
await myModel.getIndexes();
/*
[
{ name: '_id_', key: { _id_: 1}, unique: true },
{ name: 'code', key: { code: 1} }
]
*/
await myModel.createIndex({ name: 'name', key: { name: 1}, unique: true });
await myModel.createIndexes([{ name: 'name', key: { name: 1}, unique: true }, { name: 'code', key: { code: -1 }}]);
await myModel.dropIndex('name');
await myModel.dropIndexes(['name', 'code']);
const dbDriver = await myModel.getDb();
await dbDriver.specialMethod(myModel);
This package automatically logs any write operation such as:
static getter
shouldCreateLogs
to false:class MyModel extends Model {
static get shouldCreateLogs() {
return false;
}
}
You can exclude fields for logs in case you have sensitive information in your entries such as passwords, addresses, etc.
static getter
excludeFieldsInLog
:class MyModel extends Model {
static get excludeFieldsInLog() {
return [
'password',
'address',
'secret'
]
}
}
By setting this when you do an operation with an item like:
await myModel.insert({
user: 'johndoe',
password: 'some-password',
contry: 'AR',
address: 'Fake St 123'
});
It will be logged as:
{
id: '5ea30bcbe28c55870324d9f0',
user: 'johndoe',
contry: 'AR'
}
FAQs
A package for managing Janis Models
We found that @janiscommerce/model demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.