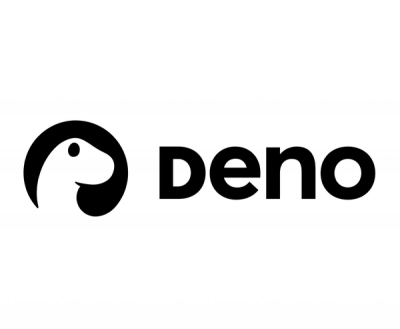
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@jest/expect
Advanced tools
This package extends `expect` library with `jest-snapshot` matchers. It exports `jestExpect` object, which can be used as standalone replacement of `expect`.
@jest/expect is a part of the Jest testing framework that provides a set of matchers to assert different conditions in your tests. It allows you to write assertions in a readable and expressive way, making it easier to test your JavaScript code.
Basic Matchers
Basic matchers allow you to test simple values. In this example, `toBe` is used to check if the result of `2 + 2` is `4`.
expect(2 + 2).toBe(4);
Truthiness
Matchers for different types of truthiness. Here, `toBeNull` checks if the value is `null`.
expect(null).toBeNull();
Numbers
Matchers for comparing numbers. In this example, `toBeGreaterThan` checks if `4` is greater than `3`.
expect(4).toBeGreaterThan(3);
Strings
Matchers for strings. This example uses `not.toMatch` to check that the string 'team' does not contain the letter 'I'.
expect('team').not.toMatch(/I/);
Arrays and Iterables
Matchers for arrays and iterables. Here, `toContain` checks if the array contains the element 'Alice'.
expect(['Alice', 'Bob']).toContain('Alice');
Exceptions
Matchers for exceptions. This example uses `toThrow` to check if a function throws an error with the message 'error'.
expect(() => { throw new Error('error'); }).toThrow('error');
Chai is a BDD / TDD assertion library for node and the browser that can be delightfully paired with any javascript testing framework. It provides a variety of assertion styles (should, expect, assert) and is highly extensible.
Expect is a simple assertion library for JavaScript. It is often used with testing frameworks like Mocha. It provides a similar set of matchers to Jest's expect, but is more lightweight and less integrated.
Should is an expressive, readable, framework-agnostic assertion library. It extends the Object prototype with a single non-enumerable property, which allows for a very expressive and readable style.
This package extends expect
library with jest-snapshot
matchers. It exports jestExpect
object, which can be used as standalone replacement of expect
.
The jestExpect
function used in Jest. You can find its documentation on Jest's website.
29.2.2
[@jest/test-sequencer]
Make sure sharding does not produce empty groups (#13476)[jest-circus]
Test marked as todo
are shown as todo when inside a focussed describe (#13504)[jest-mock]
Ensure mock resolved and rejected values are promises from correct realm (#13503)[jest-snapshot]
Don't highlight passing asymmetric property matchers in snapshot diff (#13480)FAQs
This package extends `expect` library with `jest-snapshot` matchers. It exports `jestExpect` object, which can be used as standalone replacement of `expect`.
The npm package @jest/expect receives a total of 15,399,178 weekly downloads. As such, @jest/expect popularity was classified as popular.
We found that @jest/expect demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.