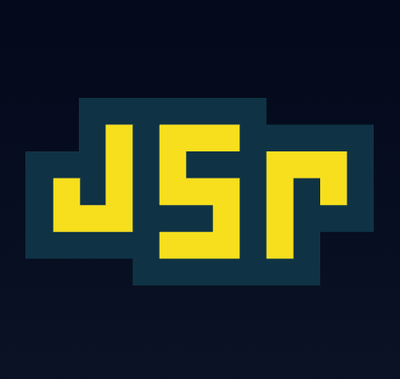
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@jimp/core
Advanced tools
@jimp/core is a JavaScript Image Manipulation Program (JIMP) that allows you to perform various image processing tasks such as resizing, cropping, and applying filters. It is designed to be simple and easy to use, making it a popular choice for developers who need to manipulate images in their Node.js applications.
Resizing Images
This feature allows you to resize images to specified dimensions. The code sample reads an image, resizes it to 256x256 pixels, and then saves the resized image.
const Jimp = require('@jimp/core');
Jimp.read('path/to/image.jpg').then(image => {
image.resize(256, 256) // resize to 256x256
.write('path/to/resized_image.jpg'); // save
}).catch(err => {
console.error(err);
});
Cropping Images
This feature allows you to crop a specific area of an image. The code sample reads an image, crops a 200x200 pixel area starting from coordinates (50, 50), and then saves the cropped image.
const Jimp = require('@jimp/core');
Jimp.read('path/to/image.jpg').then(image => {
image.crop(50, 50, 200, 200) // crop a 200x200 square starting at (50, 50)
.write('path/to/cropped_image.jpg'); // save
}).catch(err => {
console.error(err);
});
Applying Filters
This feature allows you to apply various filters to images. The code sample reads an image, applies a sepia filter, and then saves the filtered image.
const Jimp = require('@jimp/core');
Jimp.read('path/to/image.jpg').then(image => {
image.sepia() // apply sepia filter
.write('path/to/sepia_image.jpg'); // save
}).catch(err => {
console.error(err);
});
Sharp is a high-performance image processing library for Node.js. It is known for its speed and efficiency, especially with large images. Sharp supports a wide range of image formats and provides functionalities similar to @jimp/core, such as resizing, cropping, and applying filters.
GraphicsMagick (gm) is a Node.js wrapper for the GraphicsMagick and ImageMagick image processing libraries. It offers a comprehensive set of image manipulation features, including resizing, cropping, and applying filters. While gm provides more advanced functionalities, it requires the GraphicsMagick or ImageMagick software to be installed on the system.
Lightweight Image Processor (lwip) is a Node.js library for image processing. It is designed to be lightweight and fast, offering basic functionalities such as resizing, cropping, and applying filters. However, lwip is no longer actively maintained, which may be a consideration for some developers.
The main Jimp class. This class can be extended with types and bitmap manipulation functions. Out of the box it does not support any image type.
The Jimp class constructor.
Add constant or static methods to the Jimp constructor.
addConstants({
MIME_SPECIAL: 'image/special'
});
Add a bitmap manipulation method to Jimp constructor. These method should return this so that the function can be chain-able.
addJimpMethods({
cropCrazy: function() {
// Your custom image manipulation method
return this;
}
})
const image = await Jimp.read(...);
image.resize(10, Jimp.AUTO),
.cropCrazy();
await image.writeAsync('test.png');
Add a image mime type to Jimp constructor. First argument is a mime type and the second is an array of file extension for that type.
addType('image/special', ['spec', 'special']);
FAQs
Unknown package
We found that @jimp/core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.