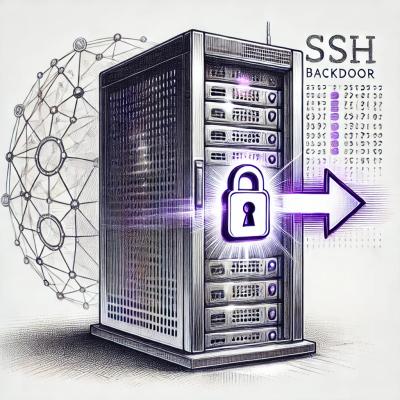
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@jmondi/oauth2-server
Advanced tools
[](https://github.com/jasonraimondi/typescript-oauth2-server) [;
authorizationServer.enableGrantType(new ClientCredentialsGrant(...);
authorizationServer.enableGrantType(new AuthCodeGrant(...);
authorizationServer.enableGrantType(new RefreshTokenGrant(...);
const app = Express();
app.use(json());
app.use(urlencoded({ extended: false }));
/token
endpointThe /token
endpoint is a back channel endpoint that issues a useable access token.
app.post("/token", async (req: Express.Request, res: Express.Response) => {
const response = new OAuthResponse(res);
try {
const oauthResponse = await authorizationServer.respondToAccessTokenRequest(req, response);
return handleResponse(req, res, oauthResponse);
} catch (e) {
handleError(e, res);
return;
}
});
/authorize
endpointThe /authorize
endpoint is a front channel endpoint that issues an authorization code. The authorization code can then be exchanged to the AuthorizationServer
endpoint for a useable access token.
The endpoint should redirect the user to login, and then to accept the scopes requested by the application, and only when the user accepts, should it send the user back to the clients redirect uri.
app.get("/authorize", async (req: Express.Request, res: Express.Response) => {
const request = new OAuthRequest(req);
try {
// Validate the HTTP request and return an AuthorizationRequest object.
const authRequest = await authorizationServer.validateAuthorizationRequest(request);
// The auth request object can be serialized and saved into a user's session.
// You will probably want to redirect the user at this point to a login endpoint.
// Once the user has logged in set the user on the AuthorizationRequest
console.log("Once the user has logged in set the user on the AuthorizationRequest");
const user = { identifier: "abc", email: "user@example.com" };
authRequest.user = user;
// At this point you should redirect the user to an authorization page.
// This form will ask the user to approve the client and the scopes requested.
// Once the user has approved or denied the client update the status
// (true = approved, false = denied)
authRequest.isAuthorizationApproved = true;
// Return the HTTP redirect response
const oauthResponse = await authorizationServer.completeAuthorizationRequest(authRequest);
return handleResponse(req, res, oauthResponse);
} catch (e) {
handleError(e, res);
}
});
Deciding which grant to use depends on the type of client the end user will be using.
+-------+
| Start |
+-------+
V
|
|
+------------------------+ +-----------------------+
| Have a refresh token? |>----Yes----->| Refresh Token Grant |
+------------------------+ +-----------------------+
V
|
No
|
+---------------------+
| Who is the | +--------------------------+
| Access token owner? |>---A Machine---->| Client Credentials Grant |
+---------------------+ +--------------------------+
V
|
|
A User
|
|
+----------------------+
| What type of client? |
+----------------------+
|
| +---------------------------+
|>-----------Server App---------->| Auth Code Grant with PKCE |
| +---------------------------+
|
| +---------------------------+
|>-------Browser Based App------->| Auth Code Grant with PKCE |
| +---------------------------+
|
| +---------------------------+
|>-------Native Mobile App------->| Auth Code Grant with PKCE |
+---------------------------+
For machine to machine communications
When applications request an access token to access their own resources, not on behalf of a user.
POST /token HTTP/1.1
Host: example.com
grant_type=client_credentials
&client_id=xxxxxxxxxx
&client_secret=xxxxxxxxxx
HTTP/1.1 200 OK
Content-Type: application/json
Cache-Control: no-store
Pragma: no-cache
{
"access_token":"f",
"token_type":"bearer",
"expires_in":3600,
"refresh_token":"IwOGYzYTlmM2YxOTQ5MGE3YmNmMDFkNTVk",
"scope":"create"
}
A temporary code that the client will exchange for an access token. The user authorizes the application, they are redirected back to the application with a temporary code in the URL. The application exchanges that code for the access token.
If the client is unable to keep a secret, the client secret should be undefined and should not be used during the authorization_code flow.
const code_verifier = base64urlencode(crypto.randomBytes(40));
const code_challenge = base64urlencode(crypto.createHash("sha256").update(codeVerifier).digest("hex"));
A complete authorization request will include the following parameters
GET /authorize HTTP/1.1
Host: example.com
id=123
&response_type=code
&client_id=xxxxxxx
&redirect_uri=http://localhost
&scope=xxxx
&state=xxxx
&code_challenge=xxxx
&code_challenge_method=s256
A complete access token request will include the following parameters:
POST /token HTTP/1.1
Host: example.com
grant_type=authorization_code
&code=xxxxxxxxx
&redirect_uri=xxxxxxxxxx
&client_id=xxxxxxxxxx
&code_verifier=xxxxxxxxxx
Access tokens eventually expire. The refresh_token enables the client to refresh the access token.
A complete refresh token request will include the following parameters:
POST /token HTTP/1.1
Host: example.com
Authorization: Basic Y4NmE4MzFhZGFkNzU2YWRhN
grant_type=refresh_token
&refresh_token=xxxxxxxxx
&client_id=xxxxxxxxx
&client_secret=only-required-if-client-has-secret
&scope=xxxxxxxxxx
// @todo document
Industry best practice recommends using the Authorization Code Grant without a client secret for native and browser-based apps.
// @todo document
Request
POST /token_info HTTP/1.1
Host: example.com
Authorization: Basic Y4NmE4MzFhZGFkNzU2YWRhN
token=MTQ0NjJkZmQ5OTM2NDE1ZTZjNGZmZjI3
Response
HTTP/1.1 200 OK
Host: example.com
Content-Type: application/json; charset=utf-8
{
"active": true,
"scope": "read write email",
"client_id": "J8NFmU4tJVgDxKaJFmXTWvaHO",
"username": "aaronpk",
"exp": 1437275311
}
This project was heavily influenced by the PHP League OAuth2 Server and shares a lot of the same ideas.
https://github.com/thephpleague/oauth2-server
https://tools.ietf.org/html/rfc6749#section-4.4
https://www.oauth.com/oauth2-servers/access-tokens/client-credentials/
https://www.oauth.com/oauth2-servers/access-tokens/access-token-response/
https://tools.ietf.org/html/rfc6749#section-4.1
https://tools.ietf.org/html/rfc7636
https://www.oauth.com/oauth2-servers/pkce/
https://www.oauth.com/oauth2-servers/pkce/authorization-request/
FAQs
[](https://jsr.io/@jmondi/oauth2-server) [](https://www.npmjs.com/package/@jmondi/oauth2-server) [
The npm package @jmondi/oauth2-server receives a total of 3,581 weekly downloads. As such, @jmondi/oauth2-server popularity was classified as popular.
We found that @jmondi/oauth2-server demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.