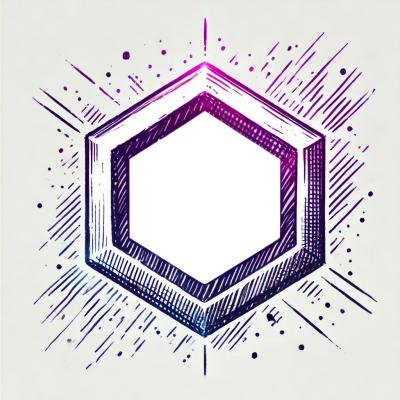
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
@journeyapps/pdf-reports
Advanced tools
Node library to generate PDF reports from HTML.
yarn add @journeyapps/pdf-reports
const pdf = require("@journeyapps/pdf-reports");
const fs = require("fs");
pdf.setApiToken(process.env.JOURNEY_PDF_KEY);
async function test() {
const result = await pdf.generatePdf({ html: "<h1>Test Pdf</h1>" });
const buffer = await result.toBuffer();
fs.writeFileSync("out.pdf", buffer);
}
test().catch(console.error);
const pdf = require("@journeyapps/pdf-reports");
const fs = require("fs");
pdf.setApiToken(process.env.JOURNEY_PDF_KEY);
const BASE_UPLOAD_CONFIG = {
bucket: process.env.JOURNEY_PDF_BUCKET,
prefix: 'test-reports/',
credentials: {
accessKeyId: process.env.AWS_ACCESS_KEY_ID,
secretAccessKey: process.env.AWS_SECRET_ACCESS_KEY,
region: 'us-east-1'
}
};
async function test() {
// This function uploads the PDF directly to S3 from the service,
// no intermediate download is required.
const result = await pdf.generateAndUploadPdf({
html: "<h1>Test PDF</h1>",
{name: 'test1.pdf', ...BASE_UPLOAD_CONFIG}
});
// URL is valid for 7 days by default
const url = result.getSignedUrl();
console.log('url', url);
}
test().catch(console.error);
// Generate from an online URL
await pdf.generatePdf({ url: "https://en.wikipedia.org/wiki/Portable_Document_Format" });
// Specify print options
await pdf.generatePdf({
html: "<h1>Test PDF</h1>",
print: {
// These are the defaults used by the service.
// Specify any of these to override the value.
landscape: false,
displayHeaderFooter: false,
printBackground: true,
scale: 1,
paperWidth: 8.27, // A4
paperHeight: 11.69, // A4
marginTop: 0,
marginBottom: 0,
marginLeft: 0,
marginRight: 0,
pageRanges: ''
}
});
Official documentation for the options are here:
https://chromedevtools.github.io/devtools-protocol/1-3/Page#method-printToPDF
A template for a header and/or footer can be provided, to render on every page. When specifying a class of pageNumber, date, title, url or totalPages, the contents of the tag is automatically replaced with the computed content.
await pdf.generatePdf({
html: "<h1>Test PDF</h1>",
print: {
// These are the defaults used by the service.
// Specify any of these to override the value.
landscape: false,
displayHeaderFooter: true,
headerTemplate: `
<div class="pageNumber" id='num' style="font-size: 10px;"></div>
<div class="date" style="font-size: 10px;"></div>
<div class="title" style="font-size: 10px;"></div>
<div class="url" style="font-size: 10px;"></div>
<div class="totalPages" style="font-size: 10px;"></div>
`,
footerTemplate: '',
printBackground: true,
scale: 1,
paperWidth: 8.27, // A4
paperHeight: 11.69, // A4
marginTop: 1,
marginBottom: 1,
marginLeft: 1,
marginRight: 1,
pageRanges: ''
}
});
There are some caveats and limitations to be aware of:
displayHeaderFooter
must be set to true for the templates to have an effect.-webkit-print-color-adjust:exact;
should be added to any styles that rely on background colors.By default, a Chrome rendering service is used. To use DocRaptor instead,
use the generatePdfDocRaptor
function instead:
const pdf = require("@journeyapps/pdf-reports");
const fs = require("fs");
pdf.setDocRaptorToken(process.env.DOCRAPTOR_TOKEN);
async function test() {
const result = await pdf.generatePdfDocRaptor({
html: "<h1>Test Pdf</h1>",
docraptor: {
// Any additional DocRaptor API options here
}
});
const buffer = await result.toBuffer();
fs.writeFileSync("out.pdf", buffer);
// The PDF may be uploaded to S3 afterwards:
const s3result = await pdf.uploadToS3(result, {name: 'test1.pdf', ...BASE_UPLOAD_CONFIG}});
const url = s3result.getSignedUrl();
console.log('url', url);
}
test().catch(console.error);
Clone this repo, then run:
yarn
To run the tests, the following environment variables are required:
JOURNEY_PDF_KEY # Key for the PDF service
JOURNEY_PDF_BUCKET # AWS bucket name to store test reports
# AWS Credentials
AWS_ACCESS_KEY_ID
AWS_SECRET_ACCESS_KEY
Then run:
yarn test
FAQs
Node library to generate PDF reports from HTML.
The npm package @journeyapps/pdf-reports receives a total of 0 weekly downloads. As such, @journeyapps/pdf-reports popularity was classified as not popular.
We found that @journeyapps/pdf-reports demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.