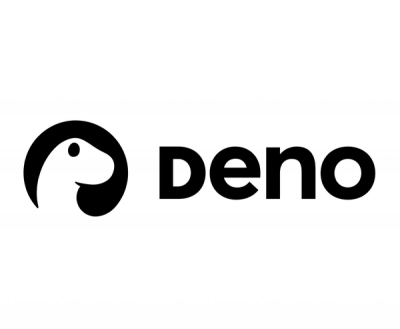
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@jridgewell/trace-mapping
Advanced tools
The @jridgewell/trace-mapping package is designed to work with source maps, which are files that provide a mapping between the transformed, bundled, or minified code and the original source files. This package allows users to trace the source location of a piece of code through a source map, which is particularly useful for debugging purposes when working with compiled or minified JavaScript.
Trace Source Location
This feature allows you to trace the original source location of a specific line and column in the transformed file. You can use the source map to find out where in the original source code a piece of the transformed code came from.
{"version":3,"file":"min.js","names":["bar","baz","n"],"sources":["one.js","two.js"],"sourceRoot":"http://example.com/www/js/","mappings":"CAAC,IAAI,IAAM,SAAUA,GAClB,OAAOC,MAAM,K"}
Generate Source Map
This feature allows you to generate a new source map by adding mappings between the original and the transformed code. This is useful when you are creating a new tool that transforms code and you want to provide source maps for debugging.
const { SourceMapGenerator } = require('@jridgewell/trace-mapping');
const generator = new SourceMapGenerator({ file: 'min.js' });
generator.addMapping({
generated: { line: 1, column: 0 },
source: 'one.js',
original: { line: 1, column: 0 }
});
const map = generator.toJSON();
The 'source-map' package provides similar functionalities for generating and consuming source maps. It allows users to create new source maps, parse existing ones, and find original source positions from generated code. Compared to @jridgewell/trace-mapping, 'source-map' is a more established package with a broader user base and has been a standard choice for source map operations in the JavaScript ecosystem.
The 'source-map-js' package is a fork of the 'source-map' library that aims to be faster and more efficient. It offers similar features for working with source maps, such as generating source maps and finding original positions from generated code. The comparison with @jridgewell/trace-mapping would be similar to that with 'source-map', with the additional consideration of performance improvements claimed by 'source-map-js'.
Trace the original position through a source map
trace-mapping
allows you to take the line and column of an output file and trace it to the
original location in the source file through a source map.
You may already be familiar with the source-map
package's SourceMapConsumer
. This
provides the same originalPositionFor
API, without requires WASM.
npm install @jridgewell/trace-mapping
import { TraceMap } from '@jridgewell/trace-mapping';
// also exported as default.
const tracer = new TraceMap({
version: 3,
sources: ['input.js'],
names: ['foo'],
mappings: 'KAyCIA',
});
// Lines start at line 1, columns at column 0.
const traced = tracer.originalPositionFor({ line: 1, column: 5 });
assert.deepEqual(traced, {
source: 'input.js',
line: 42,
column: 4,
name: 'foo',
});
We also provide a lower level API to get the actual segment that matches our line and column. Unlike
originalPositionFor
, traceSegment
uses a 0-base for line
:
// line is 0-base.
const traced = tracer.originalPositionFor(/* line */ 0, /* column */ 5);
// Segments are [outputColumn, sourcesIndex, sourceLine, sourceColumn, namesIndex]
// Again, line is 0-base and so is sourceLine
assert.deepEqual(traced, [5, 0, 41, 4, 0]);
FAQs
Trace the original position through a source map
The npm package @jridgewell/trace-mapping receives a total of 69,749,033 weekly downloads. As such, @jridgewell/trace-mapping popularity was classified as popular.
We found that @jridgewell/trace-mapping demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.