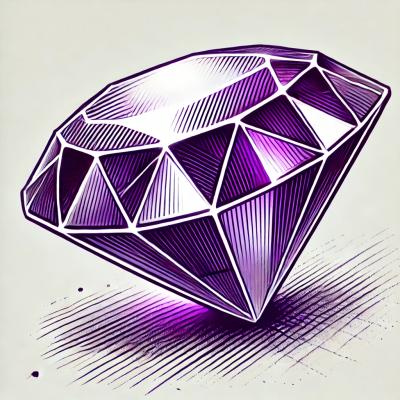
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@jsenv/url-meta
Advanced tools
@jsenv/url-meta allows to associate value to urls using pattern matching.
import { urlToMeta } from "@jsenv/url-meta"
// conditionally associates url and values
const structuredMetaMap = {
color: {
"file:///*": "black",
"file:///*.js": "red",
},
}
const urlToColor = (url) => {
return urlToMeta({ url, structuredMetaMap }).color
}
console.log(`file.json color is ${urlToColor("file:///file.json")}`)
console.log(`file.js color is ${urlToColor("file:///file.js")}`)
Code above logs
file.json color is black
file.js color is red
pattern | Description |
---|---|
**/ | Everything |
*/**/ | Inside a directory |
**/.*/ | Inside directory starting with a dot |
**/node_modules/ | Inside any node_modules directory |
node_modules/ | Inside root node_modules directory |
**/*.map | Ending with .map |
**/*.test.* | Contains .test. |
* | Inside the root directory only |
*/* | Inside a directory of depth 1 |
metaMap and structuredMetaMap below translates into: "files are visible except thoose in .git/ directory"
const metaMap = {
"**/*": { visible: true },
"**/.git/": { visible: false },
}
const structuredMetaMap = {
visible: {
"**/*/": true,
"**/.git/": false,
},
}
structuredMetaMap allows to group patterns per property which are easier to read and compose. For this reason it's the object structure used by @jsenv/url-meta API.
applyPatternMatching is a function returning a matchResult indicating if and how a pattern matches an url.
import { applyPatternMatching } from "@jsenv/url-meta"
const matchResult = applyPatternMatching({
pattern: "file:///**/*",
url: "file://Users/directory/file.js",
})
matchResult.matched // true
pattern parameter is a string looking like an url but where *
and **
can be used so that one specifier can match several url.
This parameter is required.
url parameter is a string representing a url.
This parameter is required.
matchResult represents if and how a pattern matches an url.
import { applyPatternMatching } from "@jsenv/url-meta"
const fullMatch = applyPatternMatching({
pattern: "file:///**/*",
url: "file:///Users/directory/file.js",
})
console.log(JSON.stringify(fullMatch, null, " "))
{
"matched": true,
"patternIndex": 12,
"urlIndex": 31,
"matchGroups": ["file.js"]
}
import { applyPatternMatching } from "@jsenv/url-meta"
const partialMatch = applyPatternMatching({
pattern: "file:///*.js",
url: "file:///file.jsx",
})
console.log(JSON.stringify(partialMatch, null, " "))
{
"matched": false,
"patternIndex": 12,
"urlIndex": 15,
"matchGroups": ["file"]
}
normalizeStructuredMetaMap is a function resolving structuredMetaMap keys against an url.
import { normalizeStructuredMetaMap } from "@jsenv/url-meta"
const structuredMetaMapNormalized = normalizeStructuredMetaMap(
{
visible: {
"**/*/": true,
"**/.git/": false,
},
},
"file:///Users/directory/",
)
console.log(JSON.stringify(structuredMetaMapNormalized, null, " "))
{
"visible": {
"file:///Users/directory/**/*/": true,
"file:///Users/directory/**/.git/": false
}
}
urlCanContainsMetaMatching is a function designed to ignore directory content that would never have specific metas.
import { urlCanContainsMetaMatching } from "@jsenv/url-meta"
const structuredMetaMap = {
color: {
"file:///**/*": "blue",
"file:///**/node_modules/": "green",
},
}
const firstUrlCanHaveFilesWithColorBlue = urlCanContainsMetaMatching({
url: "file:///src/",
specifierMetaMap,
predicate: ({ color }) => color === "blue",
})
firstUrlCanHaveFilesWithColorBlue // true
const secondUrlCanHaveFileWithColorBlue = urlCanContainsMetaMatching({
url: "file:///node_modules/src/",
specifierMetaMap,
predicate: ({ color }) => color === "blue",
})
secondUrlCanHaveFileWithColorBlue // false
urlToMeta is a function returning an object being the composition of all meta where pattern matched the url.
import { urlToMeta } from "@jsenv/url-meta"
const structuredMetaMap = {
insideSrc: {
"file:///src/": true,
},
extensionIsJs: {
"file:///**/*.js": true,
},
}
const urlA = "file:///src/file.js"
const urlB = "file:///src/file.json"
console.log(
`${urlA}: ${JSON.stringify(
urlToMeta({ url: urlA, structuredMetaMap }),
null,
" ",
)}`,
)
console.log(
`${urlB}: ${JSON.stringify(
urlToMeta({ url: urlB, structuredMetaMap }),
null,
" ",
)}`,
)
Console output
file:///src/file.js: {
"insideSrc": true,
"extensionIsJs": true
}
file:///src/file.json: {
"insideSrc": true
}
npm install @jsenv/url-meta
Can be used in browsers and Node.js.
36.1.0
ignore
param to build
and startDevServer
FAQs
Associate value to urls using pattern matching.
The npm package @jsenv/url-meta receives a total of 232 weekly downloads. As such, @jsenv/url-meta popularity was classified as not popular.
We found that @jsenv/url-meta demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.