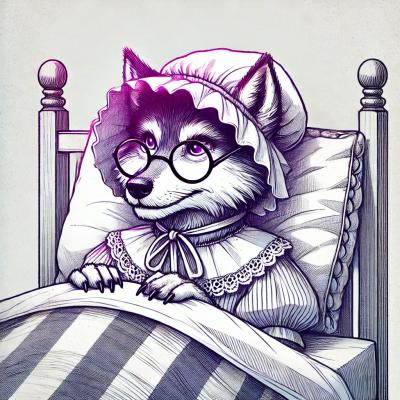
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@kesha-antonov/react-native-action-cable
Advanced tools
Use Rails 5+ ActionCable channels with React Native for realtime magic.
Use Rails 5+ ActionCable channels with React Native for realtime magic.
This is a fork from https://github.com/schneidmaster/action-cable-react
The react-native-action-cable
package exposes two modules: ActionCable, Cable.
ActionCable
: holds info and logic of connection and automatically tries to reconnect when connection is lost.Cable
: holds references to channels(subscriptions) created by action cable.yarn add react-native-action-cable
Import:
import {
ActionCable,
Cable,
} from 'react-native-action-cable'
Define once ActionCable and Cable in your application setup in your store (like Redux
or MobX
).
Create your consumer:
const actionCable = ActionCable.createConsumer('ws://localhost:3000/cable')
Right after that create Cable instance. It'll hold info of our channels.
const cable = new Cable({})
Then, you can subscribe to channel:
const channel = cable.setChannel(
`chat_${chatId}_${userId}`, // channel name to which we will pass data from Rails app with `stream_from`
actionCable.subscriptions.create({
channel: 'ChatChannel', // from Rails app app/channels/chat_channel.rb
chatId,
otherParams...
})
)
channel
.on( 'received', this.handleReceived )
.on( 'connected', this.handleConnected )
.on( 'rejected', this.handleDisconnected )
.on( 'disconnected', this.handleDisconnected )
...later we can remove event listeners and unsubscribe from channel:
const channelName = `chat_${chatId}_${userId}`
const channel = cable.channel(channelName)
if (channel) {
channel
.removeListener( 'received', this.handleReceived )
.removeListener( 'connected', this.handleConnected )
.removeListener( 'rejected', this.handleDisconnected )
.removeListener( 'disconnected', this.handleDisconnected )
channel.unsubscribe()
delete( cable.channels[channelName] )
}
You can combine React's lifecycle hooks componentDidMount
and componentWillUnmount
to subscribe and unsubscribe from channels. Or implement custom logic in your store
.
Here's example how you can handle events:
handleReceived = (data) => {
switch(data.type) {
'new_incoming_message': {
this.onNewMessage(data.message)
}
...
}
}
handleConnected = () => {
if (this.state.isWebsocketConnected) return
this.setState({ isWebsocketConnected: true })
}
handleDisconnected = () => {
if (!this.state.isWebsocketConnected) return
this.setState({ isWebsocketConnected: false })
}
Send message to Rails app:
cable.channel(channelName).perform('send_message', { text: 'Hey' })
cable.channel('NotificationsChannel').perform('appear')
ActionCable
top level methods:
.createConsumer(websocketUrl)
- create actionCable consumer and start connecting.startDebugging()
- start logging.stopDebugging()
- stop loggingActionCable
instance methods:
.open()
- try connect.connection.isOpen()
- check if connected
.connection.isActive()
- check if connected
or connecting
.subscriptions.create({ channel, otherParams... })
- create subscription to Rails app.disconnect()
- disconnects from Rails appCable
instance methods:
.setChannel(name, actionCable.subscriptions.create())
- set channel to get it later.channel(name)
- get channel by namechannel
methods:
.perform(action, data)
- send message to channel. action - string
, data - json
.on(eventName, eventListener)
- subscribe to events. eventName can be received
, connected
, rejected
, disconnected
.removeListener(eventName, eventListener)
- unsubscribe from event.unsubscribe()
- unsubscribe from channelObviously, this project is heavily indebted to the entire Rails team, and most of the code in lib/action_cable
is taken directly from Rails 5. This project also referenced fluxxor for implementation details and props binding.
MIT
FAQs
Use Rails 5+ ActionCable channels with React Native for realtime magic.
We found that @kesha-antonov/react-native-action-cable demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.