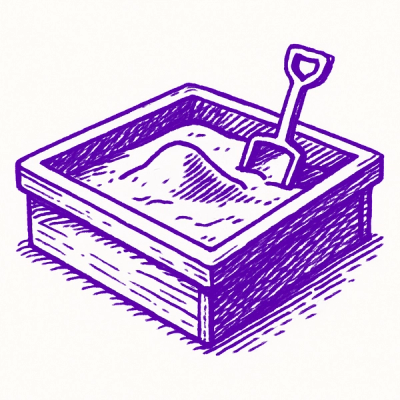
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
@keyv/redis
Advanced tools
@keyv/redis is an npm package that provides a Redis storage adapter for Keyv, a simple key-value storage library. It allows you to use Redis as a backend for storing key-value pairs, making it easy to integrate Redis into your Node.js applications.
Basic Key-Value Storage
This feature allows you to store and retrieve basic key-value pairs using Redis as the backend. The code sample demonstrates how to set and get a value from the Redis store.
const Keyv = require('keyv');
const keyv = new Keyv('redis://user:pass@localhost:6379');
(async () => {
await keyv.set('foo', 'bar');
const value = await keyv.get('foo');
console.log(value); // 'bar'
})();
Expiration of Keys
This feature allows you to set an expiration time for keys. The code sample demonstrates how to set a key with an expiration time and shows that the key is no longer available after the specified time.
const Keyv = require('keyv');
const keyv = new Keyv('redis://user:pass@localhost:6379');
(async () => {
await keyv.set('foo', 'bar', 1000); // Expires in 1 second
setTimeout(async () => {
const value = await keyv.get('foo');
console.log(value); // undefined
}, 1500);
})();
Namespace Support
This feature allows you to use namespaces to avoid key collisions. The code sample demonstrates how to set and get a value within a specific namespace.
const Keyv = require('keyv');
const keyv = new Keyv({ uri: 'redis://user:pass@localhost:6379', namespace: 'myapp' });
(async () => {
await keyv.set('foo', 'bar');
const value = await keyv.get('foo');
console.log(value); // 'bar'
})();
ioredis is a robust, full-featured Redis client for Node.js. It supports advanced Redis features like clustering, sentinel, and pipelining. Compared to @keyv/redis, ioredis offers more direct control over Redis commands and is suitable for more complex use cases.
redis is the official Node.js client for Redis. It provides a straightforward API for interacting with Redis and supports all Redis commands. While @keyv/redis focuses on key-value storage with a simple API, redis offers more granular control and flexibility.
node-cache is an in-memory caching module for Node.js. It provides a simple API for storing key-value pairs with optional expiration times. Unlike @keyv/redis, node-cache does not use Redis as a backend and is suitable for use cases where in-memory storage is sufficient.
Redis storage adapter for Keyv
Redis storage adapter for Keyv.
createKeyv
function for easy creation of Keyv instances.npm install --save keyv @keyv/redis
Here is a standard use case where we implement Keyv
and @keyv/redis
:
import Keyv from 'keyv';
import KeyvRedis from '@keyv/redis';
const keyv = new Keyv(new KeyvRedis('redis://user:pass@localhost:6379'));
keyv.on('error', handleConnectionError);
Here is the same example but with the Keyv
instance created with the createKeyv
function:
import { createKeyv } from '@keyv/redis';
const keyv = createKeyv('redis://user:pass@localhost:6379');
You only have to import the @keyv/redis
library if you are using the createKeyv
function. 🎉 Otherwise, you can import Keyv
and @keyv/redis
independently.
Here you can pass in the Redis options directly:
import Keyv from 'keyv';
import KeyvRedis from '@keyv/redis';
const redisOptions = {
url: 'redis://localhost:6379', // The Redis server URL (use 'rediss' for TLS)
password: 'your_password', // Optional password if Redis has authentication enabled
socket: {
host: 'localhost', // Hostname of the Redis server
port: 6379, // Port number
reconnectStrategy: (retries) => Math.min(retries * 50, 2000), // Custom reconnect logic
tls: false, // Enable TLS if you need to connect over SSL
keepAlive: 30000, // Keep-alive timeout (in milliseconds)
}
};
const keyv = new Keyv(new KeyvRedis(redisOptions));
Or you can create a new Redis instance and pass it in with KeyvOptions
such as setting the store
:
import Keyv from 'keyv';
import KeyvRedis, { createClient } from '@keyv/redis';
const redis = createClient('redis://user:pass@localhost:6379');
const keyvRedis = new KeyvRedis(redis);
const keyv = new Keyv({ store: keyvRedis});
The major change from v4 to v5 is that we are now using v5 of the @redis/client
library which has a new API. This means that some methods have changed but it should be a drop-in replacement for most use cases.
You can pass in options to the KeyvRedis
constructor. Here are the available options:
export type KeyvRedisOptions = {
/**
* Namespace for the current instance.
*/
namespace?: string;
/**
* Separator to use between namespace and key.
*/
keyPrefixSeparator?: string;
/**
* Number of keys to delete in a single batch.
*/
clearBatchSize?: number;
/**
* Enable Unlink instead of using Del for clearing keys. This is more performant but may not be supported by all Redis versions.
*/
useUnlink?: boolean;
/**
* Whether to allow clearing all keys when no namespace is set.
* If set to true and no namespace is set, iterate() will return all keys.
* Defaults to `false`.
*/
noNamespaceAffectsAll?: boolean;
/**
* This is used to throw an error if the client is not connected when trying to connect. By default, this is
* set to true so that it throws an error when trying to connect to the Redis server fails.
*/
throwOnConnectError?: boolean;
/**
* This is used to throw an error if at any point there is a failure. Use this if you want to
* ensure that all operations are successful and you want to handle errors. By default, this is
* set to false so that it does not throw an error on every operation and instead emits an error event
* and returns no-op responses.
* @default false
*/
throwOnErrors?: boolean;
/**
* Timeout in milliseconds for the connection. Default is undefined, which uses the default timeout of the Redis client.
* If set, it will throw an error if the connection does not succeed within the specified time.
* @default undefined
*/
connectionTimeout?: number;
};
You can pass these options when creating a new KeyvRedis
instance:
import Keyv from 'keyv';
import KeyvRedis from '@keyv/redis';
const keyvRedis = new KeyvRedis({
namespace: 'my-namespace',
keyPrefixSeparator: ':',
clearBatchSize: 1000,
useUnlink: true,
noNamespaceAffectsAll: false,
connectTimeout: 200
});
const keyv = new Keyv({ store: keyvRedis });
You can also set these options after the fact by using the KeyvRedis
instance properties:
import {createKeyv} from '@keyv/redis';
const keyv = createKeyv('redis://user:pass@localhost:6379');
keyv.store.namespace = 'my-namespace';
createKeyv
functionThe createKeyv
function is a convenience function that creates a new Keyv
instance with the @keyv/redis
store. It automatically sets the useKeyPrefix
option to false
. Here is an example of how to use it:
import { createKeyv } from '@keyv/redis';
const keyv = createKeyv('redis://user:pass@localhost:6379');
To use a namespace you can do it here and this will set Keyv up correctly to avoid the double namespace issue:
import { createKeyv } from '@keyv/redis';
const keyv = createKeyv('redis://user:pass@localhost:6379', {namespace: 'my-namespace'});
createKeyvNonBlocking
functionThe createKeyvNonBlocking
function is a convenience function that creates a new Keyv
instance with the @keyv/redis
store does what createKeyv
does but also disables throwing errors, removes the offline queue redis functionality, and reconnect strategy so that when used as a secondary cache in libraries such as cacheable it does not block the primary cache. This is useful when you want to use Redis as a secondary cache and do not want to block the primary cache on connection errors or timeouts when using nonBlocking
. Here is an example of how to use it:
import { createKeyvNonBlocking } from '@keyv/redis';
const keyv = createKeyvNonBlocking('redis://user:pass@localhost:6379');
You can set a namespace for your keys. This is useful if you want to manage your keys in a more organized way. Here is an example of how to set a namespace
with the store
option:
import Keyv from 'keyv';
import KeyvRedis, { createClient } from '@keyv/redis';
const redis = createClient('redis://user:pass@localhost:6379');
const keyvRedis = new KeyvRedis(redis);
const keyv = new Keyv({ store: keyvRedis, namespace: 'my-namespace', useKeyPrefix: false });
To make this easier, you can use the createKeyv
function which will automatically set the namespace
option to the KeyvRedis
instance:
import { createKeyv } from '@keyv/redis';
const keyv = createKeyv('redis://user:pass@localhost:6379', { namespace: 'my-namespace' });
This will prefix all keys with my-namespace:
and will also set useKeyPrefix
to false
. This is done to avoid double prefixing of keys as we transition out of the legacy behavior in Keyv. You can also set the namespace after the fact:
keyv.namespace = 'my-namespace';
NOTE: If you plan to do many clears or deletes, it is recommended to read the Performance Considerations section.
If you are using Keyv
with @keyv/redis
as the storage adapter, you may notice that keys are being prefixed twice. This is because Keyv
has a default prefixing behavior that is applied to all keys. To fix this, you can set the useKeyPrefix
option to false
when creating the Keyv
instance:
import Keyv from 'keyv';
import KeyvRedis from '@keyv/redis';
const keyv = new Keyv(new KeyvRedis('redis://user:pass@localhost:6379'), { useKeyPrefix: false });
To make this easier, you can use the createKeyv
function which will automatically set the useKeyPrefix
option to false
:
import { createKeyv } from '@keyv/redis';
const keyv = createKeyv('redis://user:pass@localhost:6379');
When initializing KeyvRedis
, you can specify the type of the values you are storing and you can also specify types when calling methods:
import Keyv from 'keyv';
import KeyvRedis, { createClient } from '@keyv/redis';
type User {
id: number
name: string
}
const redis = createClient('redis://user:pass@localhost:6379');
const keyvRedis = new KeyvRedis<User>(redis);
const keyv = new Keyv({ store: keyvRedis });
await keyv.set("user:1", { id: 1, name: "Alice" })
const user = await keyv.get("user:1")
console.log(user.name) // 'Alice'
// specify types when calling methods
const user = await keyv.get<User>("user:1")
console.log(user.name) // 'Alice'
With namespaces being prefix based it is critical to understand some of the performance considerations we have made:
clear()
- We use the SCAN
command to iterate over keys. This is a non-blocking command that is more efficient than KEYS
. In addition we are using UNLINK
by default instead of DEL
. Even with that if you are iterating over a large dataset it can still be slow. It is highly recommended to use the namespace
option to limit the keys that are being cleared and if possible to not use the clear()
method in high performance environments. If you don't set namespaces, you can enable noNamespaceAffectsAll
to clear all keys using the FLUSHDB
command which is faster and can be used in production environments.
delete()
- By default we are now using UNLINK
instead of DEL
for deleting keys. This is a non-blocking command that is more efficient than DEL
. If you are deleting a large number of keys it is recommended to use the deleteMany()
method instead of delete()
.
clearBatchSize
- The clearBatchSize
option is set to 1000
by default. This is because Redis has a limit of 1000 keys that can be deleted in a single batch. If no namespace is defined and noNamespaceAffectsAll is set to true
this option will be ignored and the FLUSHDB
command will be used instead.
useUnlink
- This option is set to true
by default. This is because UNLINK
is a non-blocking command that is more efficient than DEL
. If you are not using UNLINK
and are doing a lot of deletes it is recommended to set this option to true
.
setMany
, getMany
, deleteMany
- These methods are more efficient than their singular counterparts. These will be used by default in the Keyv
library such as when using keyv.delete(string[])
it will use deleteMany()
.
If you want to see even better performance please see the Using Cacheable with Redis section as it has non-blocking and in-memory primary caching that goes along well with this library and Keyv.
This is because we are using UNLINK
by default instead of DEL
. This is a non-blocking command that is more efficient than DEL
but will slowly remove the memory allocation.
If you are deleting or clearing a large number of keys you can disable this by setting the useUnlink
option to false
. This will use DEL
instead of UNLINK
and should reduce the memory usage.
const keyv = new Keyv(new KeyvRedis('redis://user:pass@localhost:6379', { useUnlink: false }));
// Or
keyv.useUnlink = false;
When using @keyv/redis
, it is important to handle connection errors gracefully. You can do this by listening to the error
event on the KeyvRedis
instance. Here is an example of how to do that:
import Keyv from 'keyv';
import KeyvRedis from '@keyv/redis';
const keyv = new Keyv(new KeyvRedis('redis://user:pass@localhost:6379'));
keyv.on('error', (error) => {
console.error('error', error);
});
By default, the KeyvRedis
instance will throw an error
if the connection fails to connect. You can disable this behavior by setting the throwOnConnectError
option to false
when creating the KeyvRedis
instance. If you want this to throw you will need to also set the Keyv instance to throwOnErrors: true
:
import Keyv from 'keyv';
import KeyvRedis from '@keyv/redis';
const keyv = new Keyv(new KeyvRedis('redis://bad-uri:1111', { throwOnConnectError: false }));
keyv.throwOnErrors = true; // This will throw an error if the connection fails
await keyv.set('key', 'value'); // this will throw the connection error only.
On get
, getMany
, set
, setMany
, delete
, and deleteMany
, if the connection is lost, it will emit an error and return a no-op value. You can catch this error and handle it accordingly. This is important to ensure that your application does not crash due to a lost connection to Redis.
If you want to handle connection errors, retries, and timeouts more gracefully, you can use the throwOnErrors
option. This will throw an error if any operation fails, allowing you to catch it and handle it accordingly:
There is a default Reconnect Strategy
if you pass in just a uri
connection string we will automatically create a Redis client for you with the following reconnect strategy:
export const defaultReconnectStrategy = (attempts: number): number | Error => {
// Exponential backoff base: double each time, capped at 2s.
// Parentheses make it clear we do (2 ** attempts) first, then * 100
const backoff = Math.min((2 ** attempts) * 100, 2000);
// Add random jitter of up to ±50ms to avoid thundering herds:
const jitter = (Math.random() - 0.5) * 100;
return backoff + jitter;
};
If you are wanting to see even better performance with Redis, you can use Cacheable which is a multi-layered cache library that has in-memory primary caching and non-blocking secondary caching. Here is an example of how to use it with Redis:
import KeyvRedis from '@keyv/redis';
import Cacheable from 'cacheable';
const secondary = new KeyvRedis('redis://user:pass@localhost:6379');
const cache = new Cacheable( { secondary } );
For even higher performance you can set the nonBlocking
option to true
:
const cache = new Cacheable( { secondary, nonBlocking: true } );
This will make it so that the secondary does not block the primary cache and will be very fast. 🚀
If you are using a Redis Cluster or need to use TLS, you can pass in the redisOptions
directly. Here is an example of how to do that:
import Keyv from 'keyv';
import KeyvRedis, { createCluster } from '@keyv/redis';
const cluster = createCluster({
rootNodes: [
{
url: 'redis://127.0.0.1:7000',
},
{
url: 'redis://127.0.0.1:7001',
},
{
url: 'redis://127.0.0.1:7002',
},
],
});
const keyv = new Keyv({ store: new KeyvRedis(cluster) });
You can learn more about the createCluster
function in the documentation at https://github.com/redis/node-redis/tree/master/docs.
Here is an example of how to use TLS:
import Keyv from 'keyv';
import KeyvRedis from '@keyv/redis';
const tlsOptions = {
socket: {
host: 'localhost',
port: 6379,
tls: true, // Enable TLS connection
rejectUnauthorized: false, // Ignore self-signed certificate errors (for testing)
// Alternatively, provide CA, key, and cert for mutual authentication
ca: fs.readFileSync('/path/to/ca-cert.pem'),
cert: fs.readFileSync('/path/to/client-cert.pem'), // Optional for client auth
key: fs.readFileSync('/path/to/client-key.pem'), // Optional for client auth
}
};
const keyv = new Keyv({ store: new KeyvRedis(tlsOptions) });
1000
.UNLINK
command for deleting keys isntead of DEL
.false
).noNamespaceAffectsAll
is set to true
.Quit
command. If you set force
to true
it will force the disconnect.noNamespaceAffectsAll
is set to true
.Keyv by default supports the error
event across all storage adapters. If you want to listen to other events you can do so by accessing the client
property of the KeyvRedis
instance. Here is an example of how to do that:
import {createKeyv} from '@keyv/redis';
const keyv = createKeyv('redis://user:pass@localhost:6379');
const redisClient = keyv.store.client;
redisClient.on('connect', () => {
console.log('Redis client connected');
});
redisClient.on('reconnecting', () => {
console.log('Redis client reconnecting');
});
redisClient.on('end', () => {
console.log('Redis client disconnected');
});
Here are some of the events you can listen to: https://www.npmjs.com/package/redis#events
Overall the API is the same as v3 with additional options and performance improvements. Here are the main changes:
ioredis
library has been removed in favor of the redis
aka node-redis
library. If you want to use ioredis you can use @keyv/valkey
useUnlink
option has been added to use UNLINK
instead of DEL
and set to true by default.clearBatchSize
option has been added to set the number of keys to delete in a single batch.clear()
and delete()
methods now use UNLINK
instead of DEL
. If you want to use DEL
you can set the useUnlink
option to false
.keyv
with @keyv/redis
as the storage adapter you can do the following:import Keyv from 'keyv';
import KeyvRedis from '@keyv/redis';
const redis = new KeyvRedis('redis://user:pass@localhost:6379');
const keyv = new Keyv({ store: redis, namespace: 'my-namespace', useKeyPrefix: false });
This will make it so the storage adapter @keyv/redis
will handle the namespace and not the keyv
instance. If you leave it on it will just look duplicated like my-namespace:my-namespace:key
.
We no longer support redis sets. This is due to the fact that it caused significant performance issues and was not a good fit for the library.
FAQs
Redis storage adapter for Keyv
The npm package @keyv/redis receives a total of 383,877 weekly downloads. As such, @keyv/redis popularity was classified as popular.
We found that @keyv/redis demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.