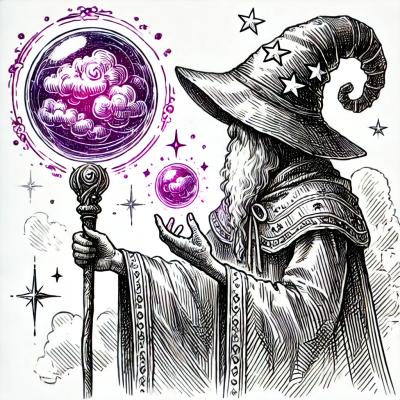
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@lanetix/microservice
Advanced tools
Wrapper for writing JWT-authenticated microservices for internal use within Lanetix.
Wrapper for writing JWT-authenticated microservices for internal use within Lanetix.
npm install --save @lanetix/microservice
Optimally, the creation of the express app should be separated out from the creation of the HTTP server for ease of testing.
// server.js - build up your express app
import express from 'express'
import { server } from 'lanetix-microservice'
import fooRouter from './routers/foo'
import bonkRouter from './routers/bonk'
const app = express()
export default server(app, {
postAuthentication: [
// middleware goes here
],
routers: {
'/foo': fooRouter,
'/bonk': bonkRouter
}
})
// web.js - bind to a port and launch your application
import { createServer } from 'http'
import server from './server'
const port = 9999
const httpServer = createServer(server)
httpServer.listen(port, () => {
console.log(`listening on port ${port}`)
})
Logging convenience methods are appended to the request
object that automatically append the x-request-id
, if available.
module.exports = (req, res, next) {
req.error('oh no!')
next()
// output will be `> 12345 oh no!` where 12345 is the request id
}
The test
client allows you to make requests to an in-memory version of your application. GET, POST, PATCH, PUT, and DELETE are supported HTTP verbs.
A user can be impersonated by providing either:
user_id
property directly on the contextuser
property on the context that has an id
import { test } from 'lanetix-microservice'
import server from '../server'
describe('Microservice tests', () => {
it('should return 200 OK for a GET to /foo', () =>
test.serve(server, { user_id: 1337 })
.get('/foo')
.expect(200)
)
it('should return 201 Created for a POST to /bonk', () =>
test.serve(server, { user_id: 1337 })
.post('/bonk')
.send({ data: 'hello' })
.expect(201)
)
})
In order to avoid a 401 unauthorized
response, an authorization token should be present on each request.
You'll need this for native get requests that aren't capable of putting headers on the request. These will be traditional anchor tags:
<a href ="http://localhost:5015/download/csv?access_token=accesstoken">
The bearer token approach will be the most common. API calls will employ this strategy:
var request = {
method: 'POST',
url: 'http://localhost:5015/api/call',
headers: {
'Authorization': 'Bearer accesstoken' // this is the important part
}
};
// send request
FAQs
Wrapper for writing JWT-authenticated microservices for internal use within Lanetix.
The npm package @lanetix/microservice receives a total of 0 weekly downloads. As such, @lanetix/microservice popularity was classified as not popular.
We found that @lanetix/microservice demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 21 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.