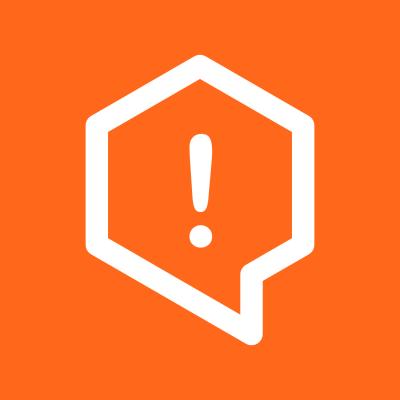
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@layerzerolabs/lz-aptos-sdk-v2
Advanced tools
The Aptos SDK is a comprehensive SDK designed to interact with the Aptos blockchain. It provides a set of utilities and modules to facilitate the development and integration of applications with the Aptos blockchain.
To install the Aptos SDK, you can use npm or yarn:
npm install @layerzerolabs/lz-aptos-sdk-v2
or
yarn add @layerzerolabs/lz-aptos-sdk-v2
import { Stage } from "@layerzerolabs/lz-definitions";
import { SDK as AptosSDK } from "@layerzerolabs/lz-aptos-sdk-v2";
import { Aptos, AptosConfig } from "@aptos-labs/ts-sdk";
import { Counter, Endpoint } from "@layerzerolabs/lz-movevm-sdk-v2";
// url is the aptos chain full node url
const sdk = new AptosSDK({
stage: Stage.SANDBOX,
provider: new Aptos(new AptosConfig({ fullnode: url })),
}).LayerzeroModule;
const counter = sdk.Counter;
const endpoint = sdk.Endpoint;
const count = await counter.getCount();
import { SandboxV2EndpointId } from '@layerzerolabs/lz-definitions'
import { Options } from '@layerzerolabs/lz-v2-utilities'
import { MnemonicAndPath } from '@layerzerolabs/move-definitions'
const counter = sdk.Counter
const endpoint = sdk.Endpoint
const sender: MnemonicAndPath = {
path: "m/44'/637'/0'/0'/26'",
mnemonic: 'test test test test test test test test test test test junk',
}
const eid = SandboxV2EndpointId.APTOS_V2_SANDBOX
const msgType = 1 // (VANILLA=1, COMPOSED=2, ABA=3, COMPOSED_ABA=4)
const value = 0 // native drop amount
const executorOptions = getExecutorLzReceiveOptions(msgType, value)
const [feeInNative] = await quote(eid, msgType, value)
const response = await this.counter.send(
sender,
eid,
msgType,
BigInt(feeInNative),
executorOptions.toBytes()
)
const txHash = response.hash // get send increment transaction hash.
// @return (native_fee, zro_fee)
async quote(
eid: number,
msgType: number,
value: string | number = 0
): Promise<[number, number]> {
const receiver = await counter.getPeer(remoteEid)
if (receiver === '') {
throw new Error(`Aptos Counter Peer not set for ${eid}`)
}
const message = Uint8Array.from([1, 2, 3, 4, 5])
return endpoint.quoteView(
counterAddress,
eid,
receiver,
message,
getExecutorLzReceiveOptions(msgType, value).toBytes(),
false // whether pay in LzToken
)
}
getExecutorLzReceiveOptions(msgType: number, value: string | number = 0): Options {
const options = Options.newOptions()
if (msgType === 1) { // VANILLA
// A -> B
options.addExecutorLzReceiveOption(300000, value)
} else if (msgType === 2) { // COMPOSED
// A -> B1 -> B2
options.addExecutorLzReceiveOption(200000, value).addExecutorComposeOption(0, 200000, 0)
} else if (msgType === 3) { // ABA
// A -> B -> A
options.addExecutorLzReceiveOption(500000, 20000)
} else if (msgType === 4) { // COMPOSED_ABA
// A -> B1 -> B2 -> A
options.addExecutorLzReceiveOption(200000, value).addExecutorComposeOption(0, 500000, '200000000000000')
}
return options
}
import { SDK as AptosSDK } from "@layerzerolabs/lz-aptos-sdk-v2";
import { Aptos, AptosConfig } from "@aptos-labs/ts-sdk";
import { Oft } from "@layerzerolabs/lz-movevm-sdk-v2";
// your oft contract address.
const address = "0x123";
// url is the aptos chain full node url
const sdk = new AptosSDK({
stage: Stage.SANDBOX,
provider: new Aptos(new AptosConfig({ fullnode: url })),
accounts: {
oft: address,
},
});
// false means native type, true is adapter type.
const oft = new Oft(sdk, false);
import { MnemonicAndPath } from '@layerzerolabs/move-definitions'
const address = '0x123'
const sdk = ...
// false means native type, true is adapter type.
const oft = new Oft(sdk, false)
const sender: MnemonicAndPath = {
path: "m/44'/637'/0'/0'/45'",
mnemonic: 'test test test test test test test test test test test junk',
}
const originalBalance = await oft.balanceOf(address ?? sdk.accountToAddress(sender))
const metadata = await oft.metadata()
const decimals = metadata.decimals
// convert balance into a human-readable string representation
const balance = (BigInt(originalBalance) / BigInt(10) ** BigInt(decimals)).toString()
Quote the OFT for a particular send without sending
@return (
oft_limit: The minimum and maximum limits that can be sent to the recipient
fees: The fees that will be applied to the amount sent
amount_sent_ld: The amount that would be debited from the sender in local decimals
amount_received_ld: The amount that would be received by the recipient in local decimals
)
import { SandboxV2EndpointId } from '@layerzerolabs/lz-definitions'
import { addressToBytes32 } from '@layerzerolabs/lz-v2-utilities'
import { MnemonicAndPath } from '@layerzerolabs/move-definitions'
const sdk = ...
// false means native type, true is adapter type.
const oft = new Oft(sdk, false)
const amountLD = 100
const dstEid = SandboxV2EndpointId.APTOS_V2_SANDBOX
const payInLzToken = false
const sender: MnemonicAndPath = {
path: "m/44'/637'/0'/0'/45'",
mnemonic: 'test test test test test test test test test test test junk',
}
const userSender = sdk.accountToAddress(sender)
const toBytes32 = addressToBytes32(userSender)
const minAmountLD = (BigInt(amountLD) * 9n) / 10n // 10% tolerance
const options = Uint8Array.from([])
const composeMessage = Uint8Array.from([])
const [oftLimit, oftFeeDetails, amountSentLD, amountReceivedLD] = await oft.quoteOft(
dstEid,
toBytes32,
BigInt(amountLD),
minAmountLD,
options,
composeMessage
)
Quote the network fees for a particular send
@return (native_fee, zro_fee)
import { SandboxV2EndpointId } from '@layerzerolabs/lz-definitions'
import { addressToBytes32 } from '@layerzerolabs/lz-v2-utilities'
import { MnemonicAndPath } from '@layerzerolabs/move-definitions'
const sdk = ...
// false means native type, true is adapter type.
const oft = new Oft(sdk, false)
const amountLD = 100
const dstEid = SandboxV2EndpointId.APTOS_V2_SANDBOX
const payInLzToken = false
const sender: MnemonicAndPath = {
path: "m/44'/637'/0'/0'/45'",
mnemonic: 'test test test test test test test test test test test junk',
}
const userSender = sdk.accountToAddress(sender)
const toBytes32 = addressToBytes32(userSender)
const minAmountLD = (BigInt(amountLD) * 9n) / 10n // 10% tolerance
const options = Uint8Array.from([])
const composeMessage = Uint8Array.from([])
const [nativeFee, lzTokenFee] = await oft.quoteSend(
userSender,
dstEid,
toBytes32,
BigInt(amountLD),
minAmountLD,
payInLzToken,
options,
composeMessage
)
const sdk = ...
// false means native type, true is adapter type.
const oft = new Oft(sdk, false)
const amountLD = 100
const dstEid = SandboxV2EndpointId.APTOS_V2_SANDBOX
const payInLzToken = false
const sender: MnemonicAndPath = {
path: "m/44'/637'/0'/0'/45'",
mnemonic: 'test test test test test test test test test test test junk',
}
const userSender = sdk.accountToAddress(sender)
const toBytes32 = addressToBytes32(userSender)
const minAmountLD = (BigInt(amountLD) * 9n) / 10n // 10% tolerance
const options = Uint8Array.from([])
const composeMessage = Uint8Array.from([])
const [nativeFee, lzTokenFee] = await oft.quoteSend(
userSender,
dstEid,
toBytes32,
BigInt(amountLD),
minAmountLD,
payInLzToken,
options,
composeMessage
)
const response = await oft.send(
sender,
dstEid,
toBytes32,
BigInt(amountLD),
minAmountLD,
BigInt(nativeFee),
BigInt(lzTokenFee),
options,
composeMessage
)
const txHash = response.hash // get send transaction hash.
FAQs
Unknown package
We found that @layerzerolabs/lz-aptos-sdk-v2 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.