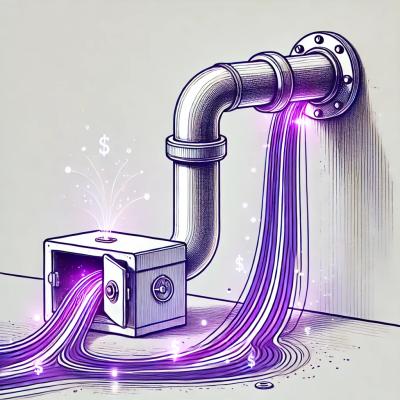
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@layerzerolabs/lz-definitions
Advanced tools
The LayerZero Definitions package provides a comprehensive set of type definitions and utilities for interacting with various blockchains. It includes enums, types, and interfaces that facilitate the development and integration of applications with the LayerZero protocol.
To install the LayerZero Definitions package, you can use npm or yarn:
npm install @layerzerolabs/lz-definitions
or
yarn add @layerzerolabs/lz-definitions
import {
networkToEndpointId,
EndpointVersion,
EndpointId,
} from "@layerzerolabs/lz-definitions";
// Converts a network name and version to an endpoint ID.
const endpointId: EndpointId = networkToEndpointId(
"ethereum-mainnet",
EndpointVersion.V1,
);
console.log(endpointId);
import {
networkToEnv,
EndpointVersion,
Environment,
} from "@layerzerolabs/lz-definitions";
// Converts a network name and version to an environment.
const environment: Environment = networkToEnv(
"ethereum-mainnet",
EndpointVersion.V1,
);
console.log(environment);
import { networkToStage, Stage } from "@layerzerolabs/lz-definitions";
// Converts a network name to a stage.
const stage: Stage = networkToStage("ethereum-mainnet");
console.log(stage);
import {
endpointIdToNetwork,
Environment,
Network,
SandboxV2EndpointId,
} from "@layerzerolabs/lz-definitions";
// Converts an endpoint ID to a network name.
const network: Network = endpointIdToNetwork(
SandboxV2EndpointId.APTOS_V2_SANDBOX,
Environment.MAINNET,
);
console.log(network);
import {
endpointIdToVersion,
EndpointVersion,
SandboxV2EndpointId,
} from "@layerzerolabs/lz-definitions";
// Converts an endpoint ID to an endpoint version.
const version: EndpointVersion = endpointIdToVersion(
SandboxV2EndpointId.APTOS_V2_SANDBOX,
);
console.log(version);
import {
endpointIdToChainKey,
ChainKey,
SandboxV2EndpointId,
} from "@layerzerolabs/lz-definitions";
// Converts an endpoint ID to a chain key.
const chainKey: ChainKey = endpointIdToChainKey(
SandboxV2EndpointId.APTOS_V2_SANDBOX,
);
console.log(chainKey);
import {
chainAndStageToEndpointId,
Chain,
Stage,
EndpointVersion,
EndpointId,
} from "@layerzerolabs/lz-definitions";
// Converts a chain and stage to an endpoint ID.
const endpointId: EndpointId = chainAndStageToEndpointId(
Chain.ETHEREUM,
Stage.MAINNET,
EndpointVersion.V1,
);
console.log(endpointId);
import {
chainAndStageToNetwork,
Chain,
Stage,
Network,
} from "@layerzerolabs/lz-definitions";
// Converts a chain and stage to a network name.
const network: Network = chainAndStageToNetwork(Chain.ETHEREUM, Stage.MAINNET);
console.log(network);
import {
endpointSpecToNetwork,
EndpointSpec,
Network,
} from "@layerzerolabs/lz-definitions";
const spec: EndpointSpec = {
chain: Chain.ETHEREUM,
stage: Stage.MAINNET,
version: EndpointVersion.V1,
env: Environment.MAINNET,
};
// Converts an endpoint specification to a network name.
const network: Network = endpointSpecToNetwork(spec);
console.log(network);
import {
endpointSpecToEnv,
EndpointSpec,
Environment,
} from "@layerzerolabs/lz-definitions";
const spec: EndpointSpec = {
chain: Chain.ETHEREUM,
stage: Stage.MAINNET,
version: EndpointVersion.V1,
env: Environment.MAINNET,
};
// Converts an endpoint specification to an environment.
const environment: Environment = endpointSpecToEnv(spec);
console.log(environment);
import {
endpointSpecToEndpointId,
EndpointSpec,
EndpointId,
} from "@layerzerolabs/lz-definitions";
const spec: EndpointSpec = {
chain: Chain.ETHEREUM,
stage: Stage.MAINNET,
version: EndpointVersion.V1,
env: Environment.MAINNET,
};
// Converts an endpoint specification to an endpoint ID.
const endpointId: EndpointId = endpointSpecToEndpointId(spec);
console.log(endpointId);
import {
endpointIdToEndpointSpec,
EndpointId,
EndpointSpec,
Environment,
SandboxV2EndpointId,
} from "@layerzerolabs/lz-definitions";
// Converts an endpoint ID and environment to an endpoint specification.
const spec: EndpointSpec = endpointIdToEndpointSpec(
SandboxV2EndpointId.APTOS_V2_SANDBOX,
Environment.MAINNET,
);
console.log(spec);
import { networkToChain, Chain } from "@layerzerolabs/lz-definitions";
// Converts a network name to a chain.
const chain: Chain = networkToChain("ethereum-mainnet");
console.log(chain);
import { networkToChainType, ChainType } from "@layerzerolabs/lz-definitions";
// Converts a network name to a chain type.
const chainType: ChainType = networkToChainType("ethereum-mainnet");
console.log(chainType);
import {
chainToChainType,
Chain,
ChainType,
} from "@layerzerolabs/lz-definitions";
// Returns the chain type for a given chain.
const chainType: ChainType = chainToChainType(Chain.ETHEREUM);
console.log(chainType);
import {
endpointIdToChain,
Chain,
SandboxV2EndpointId,
} from "@layerzerolabs/lz-definitions";
// Converts an endpoint ID to a chain.
const chain: Chain = endpointIdToChain(SandboxV2EndpointId.APTOS_V2_SANDBOX);
console.log(chain);
import {
endpointIdToStage,
Stage,
SandboxV2EndpointId,
} from "@layerzerolabs/lz-definitions";
// Converts an endpoint ID to a stage.
const stage: Stage = endpointIdToStage(SandboxV2EndpointId.APTOS_V2_SANDBOX);
console.log(stage);
import {
endpointIdToChainType,
ChainType,
SandboxV2EndpointId,
} from "@layerzerolabs/lz-definitions";
// Converts an endpoint ID to a chain type.
const chainType: ChainType = endpointIdToChainType(
SandboxV2EndpointId.APTOS_V2_SANDBOX,
);
console.log(chainType);
import { getNetworksForStage, Stage } from "@layerzerolabs/lz-definitions";
// Gets the networks for a given stage.
const networks: string[] = getNetworksForStage(Stage.MAINNET);
console.log(networks);
import { getChainIdForNetwork } from "@layerzerolabs/lz-definitions";
// Gets the chain ID for a given network.
const chainId: string = getChainIdForNetwork("ethereum", "mainnet", "1.0.0");
console.log(chainId);
import {
getNetworkForChainId,
Chain,
Stage,
SandboxV2EndpointId,
} from "@layerzerolabs/lz-definitions";
// Gets the network for a given chain ID.
const networkInfo = getNetworkForChainId(SandboxV2EndpointId.APTOS_V2_SANDBOX);
console.log(networkInfo.chainName, networkInfo.env, networkInfo.ulnVersion);
import {
isNetworkEndpointIdSupported,
EndpointVersion,
} from "@layerzerolabs/lz-definitions";
// Checks if a network endpoint ID is supported.
const isSupported: boolean = isNetworkEndpointIdSupported(
"ethereum-mainnet",
EndpointVersion.V1,
);
console.log(isSupported);
import { isEvmChain, Chain } from "@layerzerolabs/lz-definitions";
// Determines if a chain is EVM based.
const isEvm: boolean = isEvmChain(Chain.ETHEREUM);
console.log(isEvm);
FAQs
LayerZero Utility
The npm package @layerzerolabs/lz-definitions receives a total of 4,393 weekly downloads. As such, @layerzerolabs/lz-definitions popularity was classified as popular.
We found that @layerzerolabs/lz-definitions demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.